index.jsp
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8" contentType="text/html; charset=UTF-8"%>
<%
String path = request.getContextPath();
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<title>Uploadify上传</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
</head>
<body>
<a href="javascript:;"
</body>
<script type="text/javascript" >
function uploadify() {
var url = "<%=request.getContextPath()%>/uploadify2/uploadify.jsp";
window.open(url ,'newwindow','height=700,width=400,top=120,left=480,toolbar=no,menubar=no,scrollbars=yes, resizable=no,location=no, status=no');
}
</script>
</html>uploadify.jsp
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8" contentType="text/html; charset=UTF-8"%>
<%
String path = request.getContextPath();
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<title>Uploadify上传</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<link rel="stylesheet" href="uploadify/uploadify.css" type="text/css"></link>
<script type="text/javascript" src="uploadify/jquery-1.7.2.min.js"></script>
<script type="text/javascript"
src="uploadify/jquery.uploadify-3.1.min.js"></script>
<script type="text/javascript">
$(function() {
var imgData = document.getElementById('imgData');
var strHtml = "<span width='140px' height='150px'><a href='#1'><img src='#1' width='90px' height='90px' /></a></span><div> #1</div>";
$("#file_upload").uploadify({
'height' : 12,
'width' : 80,
'buttonText' : '添加图片',
'swf' : '<%=path%>/uploadify2/uploadify/uploadify.swf',
'uploader' : '<%=path%>/servlet/UploadifySerlet',
'auto' : false,
'fileTypeExts' : '*.JPG;*.GIF;*.PNG;*.BMP',
'formData' : {'userName':'','qq':''},
'onUploadSuccess' : function(file, data, response) {
var oldImgD = imgData.innerHTML;
var nHtml = strHtml.replace(/#1/g,data);
if(oldImgD != null && oldImgD !=""){
imgData.innerHTML = nHtml + "<br/>" + oldImgD;
}else{
imgData.innerHTML = nHtml;
}
}
});
});
</script>
</head>
<body>
<div align="left" style="padding-top:50px;">
<div style="overflow-y:scroll;height:290px; width:370px;">
<input type="file" name="uploadify" id="file_upload" />
</div>
<hr>
<a href="javascript:$('#file_upload').uploadify('upload','*')">开始上传</a>
<a href="javascript:$('#file_upload').uploadify('cancel', '*')">取消所有上传</a>
<div id ="imgData" style="overflow-y:scroll;height:290px; width:370px;">
</div>
</div>
</body>
</html>servlet类;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.PrintWriter;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.Iterator;
import java.util.List;
import java.util.Random;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.commons.fileupload.FileItem;
import org.apache.commons.fileupload.FileUploadException;
import org.apache.commons.fileupload.disk.DiskFileItemFactory;
import org.apache.commons.fileupload.servlet.ServletFileUpload;
public class UploadifySerlet extends BaseServlet {
/**
*
*/
private static final long serialVersionUID = 1L;
//上传文件的保存路径
protected String configPath = "attached/";
protected String dirTemp = "attached/temp/";
protected String dirName = "file";
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
this.doPost(request, response);
}
public void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
response.setContentType("text/html; charset=UTF-8");
PrintWriter out = response.getWriter();
//文件保存目录路径
String savePath = this.getServletContext().getRealPath("/") + configPath;
// 临时文件目录
String tempPath = this.getServletContext().getRealPath("/") + dirTemp;
String localHost = "http://localhost:8080/";
SimpleDateFormat sdf = new SimpleDateFormat("yyyyMM");
String ymd = sdf.format(new Date());
savePath += "/" + ymd + "/";
//创建文件夹
File dirFile = new File(savePath);
if (!dirFile.exists()) {
dirFile.mkdirs();
}
tempPath += "/" + ymd + "/";
//创建临时文件夹
File dirTempFile = new File(tempPath);
if (!dirTempFile.exists()) {
dirTempFile.mkdirs();
}
DiskFileItemFactory factory = new DiskFileItemFactory();
factory.setSizeThreshold(20 * 1024 * 1024); //设定使用内存超过5M时,将产生临时文件并存储于临时目录中。
factory.setRepository(new File(tempPath)); //设定存储临时文件的目录。
ServletFileUpload upload = new ServletFileUpload(factory);
upload.setHeaderEncoding("UTF-8");
try {
List items = upload.parseRequest(request);
Iterator itr = items.iterator();
String name = "";
String qq = "";
while (itr.hasNext()) {
FileItem item = (FileItem) itr.next();
String fileName = item.getName();
long fileSize = item.getSize();
if (!item.isFormField()) {
String fileExt = fileName.substring(fileName.lastIndexOf(".") + 1).toLowerCase();
SimpleDateFormat df = new SimpleDateFormat("yyyyMMddHHmmss");
String newFileName = df.format(new Date()) + "_" + new Random().nextInt(1000) + "." + fileExt;
try{
File uploadedFile = new File(savePath, newFileName);
/*
* 第一种方法
*
* 好处: 一目了然..简单啊...
* 弊端: 这种方法会导致上传的文件大小比原来的文件要大
*
* 推荐使用第二种
*/
//item.write(uploadedFile);
//--------------------------------------------------------------------
//第二种方法
OutputStream os = new FileOutputStream(uploadedFile);
InputStream is = item.getInputStream();
byte buf[] = new byte[1024];//可以修改 1024 以提高读取速度
int length = 0;
while( (length = is.read(buf)) > 0 ){
os.write(buf, 0, length);
}
//关闭流
os.flush();
os.close();
is.close();
System.out.println("上传成功!路径:"+savePath+"/"+newFileName);
out.print("http://localhost:8080/" + configPath + "/" + ymd + "/" + newFileName);
}catch(Exception e){
e.printStackTrace();
}
}else {
String filedName = item.getFieldName();
if (filedName.equals("userName")) {
name = item.getString();
}else {
qq = item.getString();
}
System.out.println("FieldName:"+filedName);
System.out.println("String:"+item.getString());
//System.out.println("String():"+item.getString(item.getName()));
System.out.println("===============");
}
}
} catch (FileUploadException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
out.flush();
out.close();
}
}
java语言uploadify插件图片上传
原创文章标签 java语言uploadify插件图片上 文章分类 Java 后端开发
上一篇: Apache TraceEnable关闭与测试方法
下一篇: Java 获取系统信息
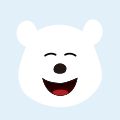
-
Bootstrap框架----单张图片上传实现---Uploadify插件
程服务器(二)---HttpClient方式上传到七牛云服务器参考:
图片 uploadify 上传 完整 示例 -
文件上传利器JQuery上传插件Uploadify
http://www.cnblogs.com/_popc/p/3877486.html
文件上传利器JQuery上传插件Uplo -
Bootstrap框架---Uploadify插件----多张图片上传交互方式二
Strap的后台框架,在此基础上记录 多张图片上传在Bootstr
spring mvc 布局 多图 上传 Uploadify -
Bootstrap框架---Uploadify插件----多张图片上传交互方式一
gMVC基础框架的基础上应用了BootSt
bootstrap 图片 插件 Uploadify 多图 -
jQuery上传插件uploadify 3.2.1 参数
1.属性名称默认值说明swf [必须设置]swf的路径uploader [必须设置]服务器端脚本
uploadify 上传 参数 文件上传 插件 -
uploadify上传图片
1、实现源码 uploadify上传图片 2、实现结果
html javascript css jquery java