<!DOCTYPE html>
<html>
<head>
<title>封装ajax</title>
</head>
<body>
<script type="text/javascript">
function createXHR()
{
if(typeof XMLHttpRequest != "undefined")
{
return new XMLHttpRequest();
}
else if(typeof ActiveXObject != "undefined")
{
var versions = [
"MSXML.2.XMLHttp.6.0",
"MSXML.2.XMLHttp.3.0",
"MSXML.2.XMLHttp"
];
for(var i = 0; i<versions.length;i++)
{
try{
return new ActiveXObject(version[i]);
}catch(e){
// 因类循环会报一个错,跳过些错
}
}
}
else
{
throw new Error("你的系统或浏览器不支持XHR对象!");
}
}
// 名值对转换字符串
function params(data)
{
var arr = [];
for(var i in data)
{
arr.push(encodeURIComponent(i)+"="+encodeURIComponent(data[i]));
}
return arr.join("&");
}
// ajax
function ajax(obj)
{
var xhr=createXHR();
obj.url=obj.url+"?rand="+Math.random();
obj.data = params(obj.data);
if(obj.method === "GET")
{
obj.url+=obj.url.indexOf("?") == -1 ?"?"+ obj.data:"&"+obj.data;
}
// if it's asynchronous
if(obj.async === true)
{
xhr.onreadystatechange = function()
{
if(xhr.readyState == 4)
{
callback();
}
}
}
xhr.open(obj.method,obj.url,obj.async);
if(obj.method === "POST")
{
xhr.setRequestHeader("Content-Type","application/x-www-form-urlencoded");
xhr.send(obj.data);
}
else
{
xhr.send(null);
}
// iF It's synchronization
if(obj.async === false)
{
callback();
}
// 封装重复调用代码
function callback()
{
if(xhr.status === 200)
{
obj.success(xhr.responseText);
}
else
{
alert("错误代码:"+xhr.status+"-错误信息:"+xhr.statusText);
}
}
}
// use ajax
addEventListener("click",function(){
ajax({
method:"POST",
url:"test.php",
data:{
"na&me":"ping",
"age":18
},
// 将对象传到text,然后对象又回调
success:function(text)
{
console.log("接收success数据为:"+text);
},
async:false
});
},false);
</script>
</body>
</html>
JavaScript的Ajax封装GET和POST
原创瓶子小 博主文章分类:JavaScript-Ajax ©著作权
文章标签 ajax 封装 文章分类 JavaScript 前端开发
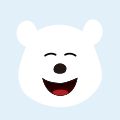
-
【JavaScript】利用Promise封装Ajax中get和post方法
PromiseES6 原生提供了 Promise 对象。Promise 对象代表了未来将要发生的事件,用来传递异步操作的消息。Promise 特点:1、Prom
ajax javascript json xml 异步操作 -
JavaScript:get和post的区别
get和post的区别
get请求 字符串 数据