#include<iostream> using namespace std; void exch( int* x, int* y ) { int temp = *x; *x =*y; *y = temp; } void HeapSort( int* array, int n )//array[0]不存放元素 { for( int i = (n-1)/2; i > 0; i-- ) { if( array[2*i] > array[i] ) exch( &array[2*i], &array[i] ); if( array[2*i+1] > array[i] && (2*i+1) < n) exch( &array[2*i+1], &array[i] ); } } void OutPut( int* array, int n ) { for( int i = 1; i < n; i++ ) { cout<<array[i]<<" "; } cout<<endl; } int main() { const int N = 12; int a[N] = {0,13,2,3,4,16,336,3,8,9,10,11}; int size = N; OutPut( a, N); while( size > 1 ) { HeapSort( a, size ); exch( &a[1], &a[size-1] ); size--; } OutPut( a, N); return 0; }
HeapSort(堆排序)
精选 转载上一篇:CountSort(计数排序)
下一篇:InsertSort(插入排序)
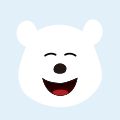
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
排序算法之计数排序的优化
排序算法之计数排序的优化
数组 计数排序 最小值 -
排序-堆排序
输入格式:输入第一行给出正整数N(≤105),随后一行给出N个(长整型范围内的)整数,其间以空
c++ 整型 #include