interface Stack<T> { push(T t); T pop(); T peek(); boolean isEmpty(); } // Sort a stack? // Does it mean changing the order of elements in the stack? // What about popping all elements out to a list, sorting, and pushing back? sort(Stack<T> stack) { Stack<T> temp = initStack(); Stack<T> toReturn = initStack(); while (!stack.isEmpty()) { T t = stack.pop(); while (!toReturn.isEmpty() && t > toReturn.peek()) { temp.push(toReturn.pop()); } toReturn.push(t); while (!temp.isEmpty()) { toReturn.push(temp.pop()); } } return toReturn; }
CC150 3.6
精选 转载furuijie8679 博主文章分类:Interview
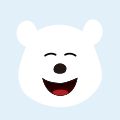
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章