package com.string; public class string1 { public static void main(String args[]){ //将char[]数组转换成String char[] ch={'h','我','是','中','国','人'}; String str=new String(ch); System.out.println(str); //结果为str="我是中国人" //将字符串转换为char数组,方法一使用getChars() char[] ch2 =new char[8]; str.getChars(0, 3, ch2, 2); System.out.println(ch2); //将字符串转换为char数组,方法二使用toCharArray()方法 ch2=str.toCharArray(); System.out.println(ch2); //获取字符串指定位置的字符charAt()方法 System.out.println(str.charAt(1)); //getBytes()方法将字符串变成一个Byte数组 byte[] bt = str.getBytes(); System.out.println(new String(bt)); //indexOf()方法判断字符串是否存在 if(str.indexOf("中国人")!=-1){ System.out.println("\"中国人\"字符串存在!"); }else{ System.out.println("\"中国人\"字符串不存在!"); } //trim()方法去掉左右空格 str=" "+str+" "; System.out.println(str); str=str.trim(); System.out.println(str); //substring() 字符串截取 System.out.println(str.substring(1,4)); //split()方法按指定的字符串拆分字符串成数组 String sp[]=str.split("是"); System.out.println(sp[0]); //toLowerCase()方法将字符串转换为小写 System.out.println(str.toLowerCase()); //toUpperCase()方法将字符串转换为大写 System.out.println(str.toUpperCase()); //startsWith()方法判断字符串是否以指定的内容开头 System.out.println(str.startsWith("h")?"以h开头":"非以h开头"); //endsWith()方法判断字符串是否以指定的内容结尾 System.out.println(str.endsWith("h")?"以h结尾":"非以h结尾"); //equals()方法区分大小写比较两个字符串 System.out.println(str.equals(new String("H我是中国人"))?"字符串相同":"字符串不相同"); //equalsIgnoreCase()方法不区分大小写比较两个字符串 System.out.println(str.equalsIgnoreCase(new String("H我是中国人"))?"字符串相同":"字符串不相同"); //replaceAll()方法将字符串中指定内容替换成其它内容 System.out.print(str.replaceAll("h","H")); } }
java中String的常用方法
原创
©著作权归作者所有:来自51CTO博客作者xiaohongyangok的原创作品,请联系作者获取转载授权,否则将追究法律责任
下一篇:java 单例设计模式
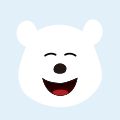
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
java中判断String类型为空和null的方法
java中判断String类型为空和null的方法
System 字符串 Apache -
STL-常用容器-string
string 类内部封装了很多成员方法例如:查找find,拷贝copy,删除delete 替换replace,插入insertstring管理char*所分配的内存,不用担心复制越界和取值越界等,由类内部进行负责(RAII)
C++STL STL常用容器 string类 string类的常用接口 -
Java中 String的常用方法总结
一、String中常用的方法,我以代码的形式,来说明这些常用的方法。 @Test public void test1(){ //1.返回字符串的
java 字符串 正则表达式 string 子字符串