/**
* 删除记录
* @access public
* @param mixed $data 主键列表 支持闭包查询条件
* @return integer 成功删除的记录数
*/
public static function destroy($data)// 删除记录
{
$model = new static();// 获取继承者ID
$query = $model->db();// 获取 数据库 操作
if (is_array($data) && key($data) !== 0) {// 如果是数组 并且 key 不等于0
$query->where($data);// where 条件进行设置
$data = null;// 赋值为空
} elseif ($data instanceof \Closure) {//如果是个匿名函数
call_user_func_array($data, [ & $query]);// 调用 $data 这样的匿名函数,传入 $query参数
$data = null;// 清空传入的参数
} elseif (is_null($data)) {// 如果传入参数 为空
return 0;//直接返回0
}
$resultSet = $query->select($data);// 获得结果集
$count = 0;// 清空条目
if ($resultSet) {// 获取结果集【对象】
foreach ($resultSet as $data) {// 遍历 新函数
$result = $data->delete();// 删除
$count += $result;// 累加
}
}
return $count;// 返回结果
}
/**
* 命名范围
* @access public
* @param string|array|Closure $name 命名范围名称 逗号分隔
* @param mixed ...$params 参数调用
* @return Model
*/
public static function scope($name)
{
if ($name instanceof Query) {// 如果是查询 实例化
return $name;
}
$model = new static();// 实例自己 的 model
$params = func_get_args();//获取传入的参数
$params[0] = $model->db();// 初始化 数据库连接
if ($name instanceof \Closure) {//闭包
call_user_func_array($name, $params);//调用函数 传递参数
} elseif (is_string($name)) {// 字符串
$name = explode(',', $name);
}
if (is_array($name)) {
foreach ($name as $scope) {// 多个函数调用
$method = 'scope' . trim($scope);
if (method_exists($model, $method)) {
call_user_func_array([$model, $method], $params);// 类 函数 参数
}
}
}
return $model;// 返回 model
}
/**
* 设置是否使用全局查询范围
* @param bool $use 是否启用全局查询范围
* @access public
* @return Model
*/
public static function useGlobalScope($use)
{
$model = new static();// 好处是 静态的
static::$useGlobalScope = $use;// 是否
return $model;// 返回设置
}
/**
* 根据关联条件查询当前模型
* @access public
* @param string $relation 关联方法名
* @param string $operator 比较操作符
* @param integer $count 个数
* @param string $id 关联表的统计字段
* @return Model
*/
public static function has($relation, $operator = '>=', $count = 1, $id = '*')
{
$model = new static();// 静态实例化
$info = $model->$relation()->getRelationInfo();// 获取信息
$table = $info['model']::getTable();// 表名
switch ($info['type']) {// 不同的类型
case Relation::HAS_MANY://生成普通的 join 语句
return $model->db()->alias('a')
->join($table . ' b', 'a.' . $info['localKey'] . '=b.' . $info['foreignKey'], $info['joinType'])
->group('b.' . $info['foreignKey'])
->having('count(' . $id . ')' . $operator . $count);
case Relation::HAS_MANY_THROUGH:// 居然还有未完成函数,牛叉
// TODO
}
}
/**
* 根据关联条件查询当前模型
* @access public
* @param string $relation 关联方法名
* @param mixed $where 查询条件(数组或者闭包)
* @return Model
*/
public static function hasWhere($relation, $where = [])
{
$model = new static();
$info = $model->$relation()->getRelationInfo();// 关联信息
switch ($info['type']) {
case Relation::HAS_ONE:
case Relation::HAS_MANY:
$table = $info['model']::getTable();
if (is_array($where)) {
foreach ($where as $key => $val) {
if (false === strpos($key, '.')) {
$where['b.' . $key] = $val;
unset($where[$key]);
}
}
}
return $model->db()->alias('a')
->field('a.*')
->join($table . ' b', 'a.' . $info['localKey'] . '=b.' . $info['foreignKey'], $info['joinType'])
->where($where);
case Relation::HAS_MANY_THROUGH:
// TODO
}
}// 同上 生成使用 has where 的关联查询
/**
* 解析模型的完整命名空间
* @access public
* @param string $model 模型名(或者完整类名)
* @return string
*/
protected function parseModel($model)
{
if (false === strpos($model, '\\')) {
$path = explode('\\', get_called_class());// 应该是有一些特殊的用法
array_pop($path);
array_push($path, Loader::parseName($model, 1));
$model = implode('\\', $path);
}// 获取完整的 命名空间
return $model;
}
/**
* 查询当前模型的关联数据
* @access public
* @param string|array $relations 关联名
* @return $this
*/
public function relationQuery($relations)
{
if (is_string($relations)) {// 关系型查询
$relations = explode(',', $relations);// 查询 explode
}
$this->relation();// relation 关系
foreach ($relations as $relation) {// relations as
$this->data[$relation] = $this->relation->getRelation($relation);
}// 关系 导入
return $this;
}
/**
* 预载入关联查询 返回数据集
* @access public
* @param array $resultSet 数据集
* @param string $relation 关联名
* @return array
*/
public function eagerlyResultSet($resultSet, $relation)
{
return $this->relation()->eagerlyResultSet($resultSet, $relation);
}// 预载入关联查询 返回数据集合
// 返回关联 结果集
[李景山php]每天TP5-20170114|thinkphp5-Model.php-7
原创
©著作权归作者所有:来自51CTO博客作者lijingshan34的原创作品,请联系作者获取转载授权,否则将追究法律责任
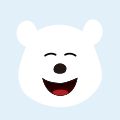
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章