<!DOCTYPE html>
<html>
<head>
<title>vue</title>
<!-- <script src="./vue.js"></script> -->
<script src="http://cdn.staticfile.org/vue/2.6.10/vue.common.dev.js"></script>
</head>
<body>
<div id="root">
{{msg}} {{num}}
<div v-html="wocao"></div>
<div v-text="wocao"></div>
<div v-on:click="handleClick">{{content}}</div>
<div v-bind:title="title">title</div>
<input type="text" :value="neirong"> <!-- v-bind:可简写为: -->
<input type="text" v-model:value="neirong">
<div>{{neirong}}</div>
<br>
姓:<input v-model="firstName">
名:<input v-model="lastName">
<div>{{firstName}} {{lastName}}</div>
<div>{{fullName}}</div>
<div>{{count}}</div>
<!-- v-show和v-if:前者是隐藏/显示,后者是销毁/创建,显然v-show更省资源(浏览器资源也是资源!) -->
<div v-if="show">show-hidden</div>
<div v-show="show">show-hidden</div>
<button v-on:click="toggleFunc">toggle</button>
<button @click="toggleFunc">toggle</button>
<!-- 最好有个:key来标记,以增加性能(:key不能重复) -->
<ul>
<li v-for="(item, index) of list1" :key="index">{{item}}</li>
</ul>
<input v-model="inputValue"/>
<button @click="addTextFunc">提交</button>
<ul>
<li v-for="(item, index) of list2" :key="index">{{item}}</li>
</ul>
<div v-html="doChange"></div>
</div>
<script>
var app = new Vue({
el: "#root",
data: {
msg: "hello world",
num: "1",
wocao: "<b>wo cao a</b>",
content: "content: hello",
title: "啊哈哈title",
neirong: "这里是内容",
firstName: "",
lastName: "",
count: 0,
show: true,
list1: [1, 2, 3],
list2: [],
doChange: '哈哈哈'
},
computed: {
fullName: function() {
return this.firstName + " " + this.lastName
}
},
watch: {
fullName: function() {
this.count++
}
},
methods: {
handleClick: function() {
alert("转换为大写");
this.content = "CONTENT: HELLO"
},
toggleFunc: function() {
/*if (this.show == false) {
this.show = true
}else {
this.show = false
}*/
this.show = !this.show
},
addTextFunc: function() {
this.list2.push(this.inputValue)
this.inputValue = ""
}
}
});
setTimeout(function() {
app.$data.doChange = "<span style='color:red'>嘿嘿嘿</span>"
}, 1000)
</script>
</body>
</html>
vue2.5+基础
原创文章标签 vue2.5+基础 文章分类 JavaScript 前端开发
©著作权归作者所有:来自51CTO博客作者梁十八的原创作品,请联系作者获取转载授权,否则将追究法律责任
下一篇:vue组件化
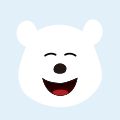
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
JavaScript基础
前端基础
Math 数组 字符串 -
Vue.js 2.5新特性介绍
TypeScriptTypeScript是一种由微软开发的自由和开源的编程语言。它是JavaScript的
javascript w3c 编程语言 typescript 修饰符 -
Vue - Vue组件基础
推荐:Vue学习汇总Vue学习(七)- Vue组件基础为了介绍Vue的组件,先用Vue
javascript vue.js html 前端 复用