upload_ajax.html
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title>upload_ajax</title>
<link rel="stylesheet" type="text/css" href="css/base.css" />
<script type="text/javascript" src="upload.js" defer="defer"></script>
<style type="text/css">
body,div,form,p,legend{margin:0;padding:0;font-size:13px;font-family:"微软雅黑";}
body{text-align:center;padding:20px;}
#container{width:900px;text-align:left;margin:0 auto;}
.preview{width:406px;overflow:hidden;}
.preview img{width:400px;border:3px solid #eee;}
.headText{margin:10px 0;}
fieldset{padding:10px;}
#postBtn{width:80px;height:30px;line-height:30px;text-align:center;border:none;margin:10px 0;cursor:pointer;background:#67efef;font:13px "微软雅黑";-moz-box-shadow:0px 0px 3px rgba(0,0,0,0.4);-webkit-box-shadow:0px 0px 3px rgba(0,0,0,0.4);box-shadow:0px 0px 3px rgba(0,0,0,0.4);}
#postBtn:hover{background:#f90;}
iframe {display:none;}
</style>
</head>
<body>
<div id="container">
<fieldset>
<legend><strong>Ajax无刷新上传图片</strong></legend>
<form action="ajaxupload.php" method="post" name="myform" id="myform" enctype="multipart/form-data">
<p><input type="file" name="filename" /></p>
<button id="postBtn">Upload</button>
</form>
<div id="upload_area"></div>
</fieldset>
</div>
</body>
</html>
ajaxupload.php
<?php
/**
* upload_ajax.php
*
*/
header("Content-type:text/html;charset=utf-8");
$filename = 'filename';
$destination_file = 'upload/';
$max_file_size = '2000000';
//echo '<pre>'; print_r($_FILES); echo '</pre>';
if(!is_uploaded_file($_FILES[$filename]['tmp_name'])){
echo "请选择你想要上传的文件";
exit;
}
if($max_file_size < $files['size']){
echo "你上传的图片过大,本系统最大图片为2MB";
exit;
}
$imgType = array(
'image/gif'
,'image/png'
,'image/jpg'
,'image/x-png'
,'image/bmp'
);
if(!in_array($files['type'],$imgType)){
echo $files['type']."不符合文件类型";
exit;
}
if(!file_exists($destination_file)){
mkdir($destination_file);
}
$filename=$files['tmp_name'];
$destination = $destination_file.$files['name'];
if (file_exists($destination)){
echo "同名文件已经存在了";
exit;
}
if(!move_uploaded_file ($files['tmp_name'], $destination_file.$files['name'])){
echo "移动文件出错";
exit;
}
//打印出上传文件到页面
echo "<div class=\"preview\">"."<img src=\"{$destination}\" alt=\"{$files['name']}\" />"."</div>";
?>
upload.js
function addEvent(element, type, handler) { //事件加载函数
if (!handler.$$guid) handler.$$guid = addEvent.guid++;
if (!element.events) element.events = {};
var handlers = element.events[type];
if (!handlers) {
handlers = element.events[type] = {};
if (element["on" + type]) {
handlers[0] = element["on" + type];
}
}
handlers[handler.$$guid] = handler;
element["on" + type] = handleEvent;
}
addEvent.guid = 1;
function removeEvent(element, type, handler) {
if (element.events && element.events[type]) {
delete element.events[type][handler.$$guid];
}
}
function handleEvent(event) {
var returnValue = true;
event = event || fixEvent(window.event);
var handlers = this.events[event.type];
for (var i in handlers) {
this.$$handleEvent = handlers[i];
if (this.$$handleEvent(event) === false) {
returnValue = false;
}
}
return returnValue;
};
function fixEvent(event) {
event.preventDefault = fixEvent.preventDefault;
event.stopPropagation = fixEvent.stopPropagation;
return event;
};
fixEvent.preventDefault = function() {
this.returnValue = false;
};
fixEvent.stopPropagation = function() {
this.cancelBubble = true;
};
function $m(theVar){
return document.getElementById(theVar)
};
function remove(theVar){
var theParent = theVar.parentNode;
theParent.removeChild(theVar);
};
function isWebKit(){
return RegExp(" AppleWebKit/").test(navigator.userAgent);
};
function ajaxUpload(form,url_action,id_element,html_show_loading,html_error_http){
var detectWebKit = isWebKit();
form = typeof(form)=="string"?$m(form):form;
var erro="";
if(form==null || typeof(form)=="undefined"){
erro += "表单第一个参数错误.\n";
}else if(form.nodeName.toLowerCase()!="form"){
erro += "这不是一个表单.\n";
}
if($m(id_element)==null){
erro += "第三个参数不存在.\n";
}
if(erro.length>0){
alert("所有的上传错误:\n" + erro);
return;
}
var iframe = document.createElement("iframe");
iframe.setAttribute("id","ajax-temp");
iframe.setAttribute("name","ajax-temp");
iframe.setAttribute("width","0");
iframe.setAttribute("height","0");
iframe.setAttribute("border","0");
iframe.setAttribute("style","width: 0; height: 0; border: none;");
form.parentNode.appendChild(iframe);
window.frames['ajax-temp'].name="ajax-temp";
var doUpload = function(){
removeEvent($m('ajax-temp'),"load", doUpload);
var cross = "javascript: ";
cross += "window.parent.$m('"+id_element+"').innerHTML = document.body.innerHTML; void(0);";
$m(id_element).innerHTML = html_error_http;
$m('ajax-temp').src = cross;
if(detectWebKit){
remove($m('ajax-temp'));
}else{
setTimeout(function(){ remove($m('ajax-temp'))}, 250);
}
}
addEvent($m('ajax-temp'),"load", doUpload);
form.setAttribute("target","ajax-temp");
form.setAttribute("action",url_action);
form.setAttribute("method","post");
form.setAttribute("enctype","multipart/form-data");
form.setAttribute("encoding","multipart/form-data");
if(html_show_loading.length > 0){
$m(id_element).innerHTML = html_show_loading;
}
form.submit();
};
//初始化事件
function start(){
var postBtn = $m('postBtn');
addEvent(postBtn,'click',function(){
var getForm = document.forms['myform'];
var destination = 'upload_ajax.php';
var hold_id ='upload_area';
var load_wait ='文件上传中,请稍后...; <br/><img src="images/loader_light_blue.gif" />';
var load_error = '上传错误,请检查路径和文件名是否正确.';
ajaxUpload(getForm,destination,hold_id,load_wait,load_error);
return false;
});
};
addEvent(window,'load',start);
php ajax 单个文件上传案例解析
原创
©著作权归作者所有:来自51CTO博客作者ibmfashion的原创作品,请联系作者获取转载授权,否则将追究法律责任
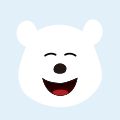
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
ajax结合文件上传类进行多文件的单个上传
通过ajax结合php文件上传类进行图片的异步上传,通过返回的图片的路径能清晰的判断错误原因,成功的时候生成图片的缩略图.通俗易懂
php文件上传 ajax结合php异步上传 php生成缩略图 -
PHP的单个文件上传、多个单文件上传、多文件上传
单文件上传upload1.php 单文件上传 请选择您要上传的文件: 我们提交到 do
php 单个文件上传 多个单文件上传 多文件上传 上传 -
单个文件上传
单个文件上传
文件上传 单个 -
php案例 文件上传并预览
php案例 文件上传并预览功能。
php 开发语言 上传 html