import core.reflect.reflect;
import core.reflect.transitiveVisitor;
// import core.reflect.nodeToString();
int func(int a, int b)
{
return a + b;
}
static assert(!hasUnusedParameters(nodeFromName("func")));
int func2(int a, int b, int c)
{
return func(a, b);
}
static assert(hasUnusedParameters(nodeFromName("func2")));
bool hasUnusedParameters(const Node n)
{
bool result = false;
if (auto fd = cast(FunctionDeclaration) n)
{
result = hasUnusedParameters(fd);
}
return result;
}
bool hasUnusedParameters(const FunctionDeclaration fd)
{
bool[const(VariableDeclaration)] parameterUsageList;
foreach(p;fd.parameters)
{
parameterUsageList[p] = false;
}
class Marker : TransitiveVisitor
{
bool[const(VariableDeclaration)]* parameterUsageList;
this(bool[const(VariableDeclaration)] *parameterUsageList)
{
this.parameterUsageList = parameterUsageList;
}
alias visit = TransitiveVisitor.visit;
override void visit(VariableDeclaration vd)
{
if (auto b = vd in *parameterUsageList)
{
*b = true;
}
}
}
scope marker = new Marker(¶meterUsageList);
(cast()fd.fbody).accept(marker);
foreach(v, unused; parameterUsageList)
{
if (!unused) return true;
}
return false;
}
d的core.反射示例
原创
©著作权归作者所有:来自51CTO博客作者fqbqrr的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:亚当嵌入d代码
下一篇:d的无效内存操作解决方式
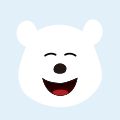
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
d的反射示例
d的反射示例
d 反射 示例 迭代 运行时库 -
java反射代码示例
一段java反射的代码示例
java 职场 示例 休闲 反射 -
Java反射机制示例
Java反射机制示例
Java开发 Java教程 -
d切片示例
d切片示例
切片 示例 d -
如何批量搜索 sql server 数据库
平时我们使用的数据库,看到的通常是一个整体,比如我们执行一条查询SQL,返回一个结果集,却不知道这条语句在MySQL内部是如何执行的,接下来我们就来简单的拆解一下MySQL,看看MySQL是由哪些“零件”组成的,在这个过程中逐步的揭开MySQL的面纱,对MySQL有个深入的理解。这样在我们以后遇到MySQL的一些异常或者问题的时候,就可以快速定位问题并解决问题。下边通过一张图来看一下SQL的执行流
mysql 拼接sql批量执行 sql server 如何设置字符串长度 sql server 客户端 sql server 查询分析器 sql server 触发器