import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* 字符串工具类,提供常用的字符串处理方法。
*/
public class StringUtils {
/**
* 判断字符串是否为空(包括 null、空字符串、纯空白字符)。
*
* @param str 待判断的字符串
* @return 如果字符串为空,则返回 true,否则返回 false
*/
public static boolean isEmpty(String str) {
return str == null || str.trim().isEmpty();
}
/**
* 判断字符串是否不为空。
*
* @param str 待判断的字符串
* @return 如果字符串不为空,则返回 true,否则返回 false
*/
public static boolean isNotEmpty(String str) {
return !isEmpty(str);
}
/**
* 判断字符串是否为手机号格式。
*
* @param phoneNumber 待判断的手机号字符串
* @return 如果字符串符合手机号格式,则返回 true,否则返回 false
*/
public static boolean isPhoneNumber(String phoneNumber) {
String regex = "^1[3-9]\\d{9}$";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(phoneNumber);
return matcher.matches();
}
/**
* 将字符串转换为大写。
*
* @param str 待转换的字符串
* @return 转换为大写后的字符串
*/
public static String toUpperCase(String str) {
if (isEmpty(str)) {
return str;
}
return str.toUpperCase();
}
/**
* 将字符串转换为小写。
*
* @param str 待转换的字符串
* @return 转换为小写后的字符串
*/
public static String toLowerCase(String str) {
if (isEmpty(str)) {
return str;
}
return str.toLowerCase();
}
/**
* 移除字符串中的所有空白字符(包括空格、制表符、换行符等)。
*
* @param str 待处理的字符串
* @return 移除空白字符后的字符串
*/
public static String removeWhitespace(String str) {
if (isEmpty(str)) {
return str;
}
return str.replaceAll("\\s+", "");
}
/**
* 判断两个字符串是否相等(忽略大小写)。
*
* @param str1 第一个字符串
* @param str2 第二个字符串
* @return 如果两个字符串相等(忽略大小写),则返回 true,否则返回 false
*/
public static boolean equalsIgnoreCase(String str1, String str2) {
if (str1 == null && str2 == null) {
return true;
}
if (str1 == null || str2 == null) {
return false;
}
return str1.equalsIgnoreCase(str2);
}
/**
* 截取字符串的指定长度,超出部分省略并添加省略号。
*
* @param str 待截取的字符串
* @param maxSize 最大长度
* @return 截取后的字符串,如果原字符串长度不超过最大长度,则返回原字符串;否则返回截取后的字符串加上省略号
*/
public static String truncate(String str, int maxSize) {
if (isEmpty(str) || str.length() <= maxSize) {
return str;
}
return str.substring(0, maxSize) + "...";
}
/**
* 统计字符串中包含的字母个数。
*
* @param str 待统计的字符串
* @return 字符串中包含的字母个数
*/
public static int countLetters(String str) {
if (isEmpty(str)) {
return 0;
}
int count = 0;
for (char c : str.toCharArray()) {
if (Character.isLetter(c)) {
count++;
}
}
return count;
}
}
StringUtils工具类
原创
©著作权归作者所有:来自51CTO博客作者wx6620bd839b85a的原创作品,请联系作者获取转载授权,否则将追究法律责任
下一篇:服务空间设计
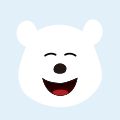
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
ES工具操作测试类
ES工具操作测试类
User System elasticsearch -
工具类-StringUtils
怀念二抱三抱
java 字符串 下划线 工具类 -
apache stringutils 工具类小结
在JAVA中我们用的最多的类应该就是String了。对于String的处理说简单也简单,但是有的
java System 字符串 数组