/*
Copyright 2016 The Kubernetes Authors.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package kubelet
import (
"context"
"fmt"
v1 "k8s.io/api/core/v1"
runtimeapi "k8s.io/cri-api/pkg/apis/runtime/v1"
)
// providerRequiresNetworkingConfiguration returns whether the cloud provider
// requires special networking configuration.
func (kl *Kubelet) providerRequiresNetworkingConfiguration() bool {
// TODO: We should have a mechanism to say whether native cloud provider
// is used or whether we are using overlay networking. We should return
// true for cloud providers if they implement Routes() interface and
// we are not using overlay networking.
if kl.cloud == nil || kl.cloud.ProviderName() != "gce" {
return false
}
_, supported := kl.cloud.Routes()
return supported
}
//该函数的功能是判断云提供商是否需要特殊的网络配置。
//具体实现中,如果kl.cloud为nil或云提供商的名称不是"gce",则返回false。
//否则,判断云提供商是否实现了Routes()接口,如果实现了则返回true,否则返回false。
// updatePodCIDR updates the pod CIDR in the runtime state if it is different
// from the current CIDR. Return true if pod CIDR is actually changed.
func (kl *Kubelet) updatePodCIDR(ctx context.Context, cidr string) (bool, error) {
kl.updatePodCIDRMux.Lock()
defer kl.updatePodCIDRMux.Unlock()
podCIDR := kl.runtimeState.podCIDR()
if podCIDR == cidr {
return false, nil
}
// kubelet -> generic runtime -> runtime shim -> network plugin
// docker/non-cri implementations have a passthrough UpdatePodCIDR
if err := kl.getRuntime().UpdatePodCIDR(ctx, cidr); err != nil {
// If updatePodCIDR would fail, theoretically pod CIDR could not change.
// But it is better to be on the safe side to still return true here.
return true, fmt.Errorf("failed to update pod CIDR: %v", err)
}
klog.InfoS("Updating Pod CIDR", "originalPodCIDR", podCIDR, "newPodCIDR", cidr)
kl.runtimeState.setPodCIDR(cidr)
return true, nil
}
//该函数用于更新Kubelet的运行时状态中的Pod CIDR。
//如果当前的Pod CIDR与给定的CIDR不同,则会尝试更新CIDR,并返回一个标志位表示是否真正更改了CIDR。
//首先,函数使用互斥锁来确保更新操作的原子性。
//然后,它将当前的Pod CIDR与给定的CIDR进行比较,如果两者相等,则直接返回false。
//如果不相等,则调用kl.getRuntime().UpdatePodCIDR(ctx, cidr)方法来尝试更新Pod CIDR。
//如果更新失败,则返回错误信息,并返回true以指示Pod CIDR已尝试更改。
//如果更新成功,则将新的CIDR设置到运行时状态中,并返回true。
// GetPodDNS returns DNS settings for the pod.
// This function is defined in kubecontainer.RuntimeHelper interface so we
// have to implement it.
func (kl *Kubelet) GetPodDNS(pod *v1.Pod) (*runtimeapi.DNSConfig, error) {
return kl.dnsConfigurer.GetPodDNS(pod)
}
//该函数是Kubelet的一个方法,用于获取Pod的DNS设置。它通过调用Kubelet中的dnsConfigurer的GetPodDNS方法来实现。
K8S kubelet_network.go源码解读
原创wx65f7b35eca645 ©著作权
文章标签 Pod ide ci 文章分类 kubernetes 云计算
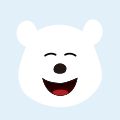