import { promptAction } from '@kit.ArkUI'
class MyDataItem {
id: number = 0
provinceid: string = ""
provincename: string = ""
cityid: string = ""
cityname: string = ""
countyid: string = ""
countyname: string = ""
}
// 示例数据
const transformedData: MyDataItem[] =
[
{
"id": 1,
"provinceid": "110000",
"provincename": "北京市",
"cityid": "110100000000",
"cityname": "北京市",
"countyid": "110101000000",
"countyname": "东城区"
},
{
"id": 2,
"provinceid": "110000",
"provincename": "北京市",
"cityid": "110100000000",
"cityname": "北京市",
"countyid": "110102000000",
"countyname": "西城区"
},
{
"id": 3,
"provinceid": "110000",
"provincename": "北京市",
"cityid": "110100000000",
"cityname": "北京市",
"countyid": "110103000000",
"countyname": "朝阳区"
},
{
"id": 4,
"provinceid": "120000",
"provincename": "天津市",
"cityid": "120100000000",
"cityname": "天津市",
"countyid": "120101000000",
"countyname": "和平区"
},
{
"id": 5,
"provinceid": "120000",
"provincename": "天津市",
"cityid": "120100000000",
"cityname": "天津市",
"countyid": "120102000000",
"countyname": "河东区"
},
{
"id": 6,
"provinceid": "120000",
"provincename": "天津市",
"cityid": "120100000000",
"cityname": "天津市",
"countyid": "120103000000",
"countyname": "河西区"
},
{
"id": 7,
"provinceid": "130000",
"provincename": "河北省",
"cityid": "130100000000",
"cityname": "石家庄市",
"countyid": "130102000000",
"countyname": "桥西区"
},
{
"id": 8,
"provinceid": "130000",
"provincename": "河北省",
"cityid": "130100000000",
"cityname": "石家庄市",
"countyid": "130103000000",
"countyname": "新华区"
},
{
"id": 9,
"provinceid": "130000",
"provincename": "河北省",
"cityid": "130100000000",
"cityname": "石家庄市",
"countyid": "130104000000",
"countyname": "长安区"
}
]
export class MyTabBean {
name: string = ""
idType: string | null = null // 'province' | 'city' | null = null
idContent: string | null = null
x: number = -1 //当前位置
width: number = -1 //当前宽度
constructor(name: string) {
this.name = name
}
}
@Entry
@Component
struct Page11 {
scroller: Scroller = new Scroller()
@State titles: MyTabBean[] = []
scrollWidthOld: number = 0
@State searchValue: string = "" //搜索内容
@State errorInfo: string = "" //错误信息
@State contentList: contentItem[] = [] //要显示的列表
aboutToAppear(): void {
this.getDataByIdType("全部", null, null)
}
build() {
Column() {
//tab栏
Scroll(this.scroller) {
Row() {
ForEach(this.titles, (item: MyTabBean, index: number) => {
if (index == 0) {
Text(item.name)
.padding({ left: '30lpx', right: '16lpx' ,top:'5lpx',bottom:'5lpx'})
.fontColor(index == this.titles.length - 1 ? "#FF1919" : "#2E2E2E")
.fontSize('30lpx')
.transition(TransitionEffect.OPACITY.animation({ duration: 200 }))
.onAreaChange((previousArea: Area, currentArea: Area) => {
if (item.x == -1) {
item.x = currentArea.position.x as number
}
if (item.width == -1) {
item.width = currentArea.width as number
}
})
.onClick(() => {
this.getDataByIdType("全部", null, null)
})
} else {
Row() {
Text(">").width('44lpx').height('44lpx')
Text(item.name)
.fontColor(index == this.titles.length - 1 ? "#FF1919" : "#2E2E2E")
.fontSize('30lpx')
}.padding({ right: '16lpx' ,top:'5lpx',bottom:'5lpx'})
.transition(TransitionEffect.OPACITY.animation({ duration: 200 }))
.onAreaChange((previousArea: Area, currentArea: Area) => {
if (item.x == -1) {
item.x = currentArea.position.x as number
}
if (item.width == -1) {
item.width = currentArea.width as number
}
})
.onClick(() => {
this.getDataByIdType(item.name, item.idType, item.idContent, index)
})
}
})
}.height('95lpx')
.onAreaChange((previousArea: Area, currentArea: Area) => {
let scrollWidth = currentArea.width as number
console.info(`scrollWidth:${scrollWidth}`)
if (this.titles.length > 0 && this.scrollWidthOld != scrollWidth) {
this.scrollWidthOld = scrollWidth
let item = this.titles[this.titles.length-1]
this.scroller.scrollTo({
xOffset: (item.x - scrollWidth / 2 + item.width / 2),
yOffset: 0,
animation: true
})
}
})
}
.scrollable(ScrollDirection.Horizontal)
.scrollBar(BarState.Off)
.borderWidth({ bottom: 1 })
.borderColor("#e3e3e3")
.align(Alignment.Start)
.width('100%')
//内容
Scroll() {
Column() {
ForEach(this.contentList, (item: contentItem, index: number) => {
if (index != 0) {
Text()
.height(1)
.width('720lpx')
.margin({ left: '30lpx' })
.backgroundColor("#e3e3e3")
}
Row() {
Column() {
Text(item.name).fontSize('30lpx').fontColor("#4B4B4B")
}.layoutWeight(1).alignItems(HorizontalAlign.Start).justifyContent(FlexAlign.Center)
}
.width('100%')
.backgroundColor(Color.White)
.padding({ top: '20lpx', bottom: '20lpx' })
.onClick(() => {
this.getDataByIdType(item.name, item.idType, item.idContent)
// else{//是叶子节点了,点击需要调用加入部门接口
// // for(let i = 0 ;i<this.contentList.length;i++){
// // this.contentList[i].isSelect = false
// // }
//
// }
})
})
}
.width('100%')
.padding({ left: '30lpx', right: '30lpx' })
.margin({ top: '20lpx', bottom: '20lpx' })
.borderWidth({ top: 1, bottom: 1 })
.borderColor("#e3e3e3")
.backgroundColor(Color.White)
}
.align(Alignment.Top)
.width('100%')
.layoutWeight(1)
.scrollBar(BarState.Off)
.backgroundColor("#fafafa")
.visibility(this.contentList.length != 0 ? Visibility.Visible : Visibility.None)
}
.height('100%')
.width('100%');
}
// 封装一个方法来根据ID类型和具体内容返回相应的数据
//idType: 'province' | 'city' | null
getDataByIdType(name: string, idType: string | null, idContent: string | null, index?: number) {
console.info(`内容填充`)
let contentItems: contentItem[] = [];
if (idType === null) {
// 如果idType为空,返回所有省份的不重复数据
const seenProvinces: object = Object();
for (let i = 0; i < transformedData.length; i++) {
const item = transformedData[i];
const provinceKey = item.provincename + item.provinceid;
if (!seenProvinces[provinceKey]) {
seenProvinces[provinceKey] = true;
contentItems.push({
name: item.provincename,
idType: 'province',
idContent: item.provinceid
});
}
}
} else {
// 如果idType为省份或城市,筛选出相应级别的所有数据
for (let i = 0; i < transformedData.length; i++) {
const item = transformedData[i];
if (idType === 'province') {
// 如果idType为省份,筛选出指定省份下的所有数据
if (item.provinceid === idContent) {
contentItems.push({
name: item.cityname,
idType: 'city',
idContent: item.cityid
});
}
} else if (idType === 'city') {
// 如果idType为城市,筛选出指定城市下的所有数据
if (item.cityid === idContent) {
contentItems.push({
name: item.countyname,
idType: 'county',
idContent: item.countyid
});
}
}
}
}
if(contentItems.length == 0){
let str = ""
for(let i = 0 ;i<transformedData.length;i++){
if(idContent ==transformedData[i].countyid ){
str = `省:${transformedData[i].provincename},市:${transformedData[i].cityname},区:${transformedData[i].countyname}`
break;
}
}
promptAction.showDialog({
title: '当前选中的是',
message: `${str}`,
buttons: [
{
text: '我知道了',
color: '#000000'
}
],
})
return
}
this.contentList = [...contentItems]
//标题栏填充
console.info(`标题栏填充`)
if (!idType) {
console.info(`用户点了根,或者是首次启动的`)
this.titles = [new MyTabBean(name)]
} else {
//清空index之后的
console.info(`index:${index}`)
console.info(`this.titles:${JSON.stringify(this.titles)}`)
if (index) {
this.titles.splice(index)
}
let myTabBean = new MyTabBean(name)
myTabBean.idType = idType
myTabBean.idContent = idContent
this.titles.push(myTabBean)
console.info(`this.titles:${JSON.stringify(this.titles)}`)
}
}
}
class contentItem {
name: string = "";
idType: string | null = null; //'province' | 'city' | 'county' | null
idContent: string | null = null;
}
【HarmonyOS】鸿蒙面包屑导航实现示例
原创
©著作权归作者所有:来自51CTO博客作者zhongcx的原创作品,请联系作者获取转载授权,否则将追究法律责任
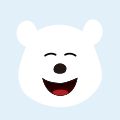
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
AmazeUI 面包屑导航
<!doctype html><html class="no-js"><ntent="IE=edge"><meta name=&
ui javascript ViewUI ico html