package com.nasc.base.util;
import java.util.*;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import java.util.regex.PatternSyntaxException;
/**
* 1. 功能:字符串工具类
* 2. 作者:SYSTEM
* 3. 创建日期:2016-5-20 15:42:54
*/
public class StringUtil {
/**
* 截取两个字符间的内容
*
* @param str
* @param strStart
* @param strEnd
* @return
* @since 2022-03-12
*/
public static String subStringStr(String str, String strStart, String strEnd) {
String result = "";
try {
/* 找出指定的2个字符在 该字符串里面的 位置 */
int strStartIndex = str.indexOf(strStart);
int strEndIndex = str.indexOf(strEnd);
/* index 为负数 即表示该字符串中 没有该字符 */
if (strStartIndex < 0) {
return "";
}
if (strEndIndex < 0) {
return "";
}
/* 开始截取 */
result = str.substring(strStartIndex, strEndIndex).substring(strStart.length());
} catch (Exception e) {
LogUtil.error("StringUtil.subStringStr", e);
}
return result;
}
/**
* 过滤特殊字符
*/
public static boolean filterSpecialChar(String c) {
String regEx = "[`~!@#$%^&*()+=|{}':;',\\[\\].<>/?~!@#¥%……&*()——+|{}‘;:”“’。,、?]";
Pattern pattern = Pattern.compile(regEx);
Matcher matcher = pattern.matcher(c);
return matcher.find();
}
/**
* 字符串的过滤特殊字符
*
* @param str
* @return
* @throws PatternSyntaxException
*/
public static String StringFilter(String str) throws PatternSyntaxException {
// 只允许字母和数字
// String regEx = "[^a-zA-Z0-9]";
// 清除掉所有特殊字符
String regEx = "[`~!@#$%^&*()+=|{}':;',//[//].<>/?~!@#¥%……&*()——+|{}‘;:”“’。,、?]";
Pattern p = Pattern.compile(regEx);
Matcher m = p.matcher(str);
String trim = m.replaceAll("").trim();
return trim.replaceAll(" ", "");
}
/**
* 将以逗号分隔的字符串转换成字符串数组
*
* @param valStr
* @return String[]
*/
public static String[] StrList(String valStr) {
int i = 0;
String TempStr = valStr;
String[] returnStr = new String[valStr.length() + 1 - TempStr.replace(",", "").length()];
valStr = valStr + ",";
while (valStr.indexOf(',') > 0) {
returnStr[i] = valStr.substring(0, valStr.indexOf(','));
valStr = valStr.substring(valStr.indexOf(',') + 1, valStr.length());
i++;
}
return returnStr;
}
/**
* 验证String是否为空
*
* @param str
* @return
*/
private static boolean isEmpty(String str) {
return (null == str) || (str.length() == 0) || ("".equals(str.trim())) || ("null".equalsIgnoreCase(str));
}
public static String isEmptyRetString(String str) {
return isEmpty(str) ? "" : str;
}
public static String isEmptyRetString(String str, String retString) {
return isEmpty(str) ? retString : str;
}
/**
* 为String创建连接
*
* @param params
* @return
*/
@SuppressWarnings({"rawtypes", "unchecked"})
public static String CreateLinkString(Map<String, String> params) {
List keys = new ArrayList(params.keySet());
Collections.sort(keys);
String prestr = "";
for (int i = 0; i < keys.size(); i++) {
String key = (String) keys.get(i);
String value = params.get(key);
if (i == keys.size() - 1)
prestr = prestr + key + "=" + value;
else {
prestr = prestr + key + "=" + value + "&";
}
}
return prestr;
}
/**
* 移除html标签
*
* @param str
* @return
*/
public static String removeHtmlTag(String str) {
try {
str = str.replaceAll("<br>", "\n");
str = str.replaceAll(" ", " ");
Pattern p = Pattern.compile("</?[A-Z]+\\b[^>]*>", 2);
Matcher m = p.matcher(str);
str = m.replaceAll("");
} catch (PatternSyntaxException pe) {
pe.printStackTrace();
}
return str;
}
@SuppressWarnings({"unchecked", "rawtypes"})
public static List getMatches(List list, String prefix) {
String prefix_upper = prefix.toUpperCase();
List matches = new ArrayList();
Iterator iter = list.iterator();
while (iter.hasNext()) {
String name = (String) iter.next();
String name_upper = name.toUpperCase();
if (name_upper.startsWith(prefix_upper)) {
matches.add(name);
}
}
return matches;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public static Map<Character, Integer> getStatistics(String s) {
Map map = new HashMap();
int size = s.length();
for (int i = 0; i < size; i++) {
char c = s.charAt(i);
map.put(Character.valueOf(c), Integer.valueOf(map.containsKey(Character.valueOf(c)) ? ((Integer) map.get(Character.valueOf(c))).intValue() + 1 : 1));
}
return map;
}
public static String addSplit(String[] array, String split) {
StringBuffer sb = new StringBuffer();
for (int i = 0; i < array.length - 1; i++) {
sb.append(array[i] + split);
}
sb.append(array[(array.length - 1)]);
return sb.toString();
}
public static String HexEncode(String str) {
String hexString = null;
if ((str != null) && (str.length() > 0)) {
char[] digital = "comd4f16b4ec3d7aaf082bab4159cfcn".toCharArray();
StringBuffer sb = new StringBuffer("");
try {
byte[] bs = str.getBytes("utf-8");
for (int i = 0; i < bs.length; i++) {
int bit = (bs[i] & 0xF0) >> 4;
sb.append(digital[bit]);
bit = bs[i] & 0xF;
sb.append(digital[bit]);
}
} catch (Exception e) {
}
hexString = sb.toString();
}
return hexString;
}
public static String HexDecode(String hexString) {
String str = null;
if ((hexString != null) && (hexString.length() > 0)) {
String digital = "comd4f16b4ec3d7aaf082bab4159cfcn";
char[] hex2char = hexString.toCharArray();
byte[] bytes = new byte[hexString.length() / 2];
for (int i = 0; i < bytes.length; i++) {
int temp = digital.indexOf(hex2char[(2 * i)]) * 16;
temp += digital.indexOf(hex2char[(2 * i + 1)]);
bytes[i] = (byte) (temp & 0xFF);
}
try {
str = new String(bytes, "utf-8");
} catch (Exception e) {
}
}
return str;
}
public static String getLimitLengthString(String str, int length) {
String view = null;
int counterOfDoubleByte = 0;
if (str == null)
return "";
try {
byte[] b = str.getBytes("GBK");
if (b.length <= length)
return str;
for (int i = 0; i < length; i++) {
if (b[i] > 0)
counterOfDoubleByte++;
}
if (counterOfDoubleByte % 2 == 0)
view = new String(b, 0, length, "GBK") + "...";
else
view = new String(b, 0, length - 1, "GBK") + "...";
} catch (Exception e) {
e.printStackTrace();
}
return view;
}
/**
* 去除String 类型的所有空格
*
* @param value
* @return
*/
public static String replaceEmpty(String value) {
if (value != null) {
value = value.replaceAll("\\s*", "");
}
return value;
}
/**
* 字符插入字符
*
* @param oldStr 原字符
* @param insertStr 插入字符
* @param position 插入位置
* @return
*/
public static String insertStr(String oldStr, String insertStr, int position) {
String rc = "";
try {
StringBuilder sb = new StringBuilder(oldStr);
sb.insert(position, insertStr);
rc = sb.toString();
} catch (Exception e) {
rc = oldStr;
}
return rc;
}
/**
* 判断字母或数字
*
* @return boolean 判断结果
* @author lx
*/
private static boolean zMOrSZ(String str) {
Pattern pattern = Pattern.compile("[0-9]|[a-z]");
Matcher ms = pattern.matcher(str);
if (!ms.matches()) {
return false;
}
return true;
}
/**
* 驼峰转下划线
*
* @param str 转换前的SQL语句
* @return string 转换后的SQL语句
*/
public static String camelToUnderline(String str) {
if (str == null || "".equals(str.trim())) {
return "";
}
int len = str.length();
StringBuilder sb = new StringBuilder(len);
for (int i = 0; i < len; i++) {
char c = str.charAt(i);
if (Character.isUpperCase(c) && (i > 0 && zMOrSZ(String.valueOf(str.charAt(i - 1))))) {
sb.append("_");
sb.append(Character.toLowerCase(c));
} else {
sb.append(c);
}
}
return sb.toString();
}
//获取指定位数的随机字符串(包含小写字母、大写字母、数字,0<length)
public static String getRandomString(int length) {
//随机字符串的随机字符库
String KeyString = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789";
StringBuffer sb = new StringBuffer();
int len = KeyString.length();
for (int i = 0; i < length; i++) {
sb.append(KeyString.charAt((int) Math.round(Math.random() * (len - 1))));
}
return sb.toString();
}
public static void main(String[] args) {
// String line="i_have_an_ipang3_pig_y";
// String camel=underline2Camel(line,true);
// System.out.println(camel);
// System.out.println(camel2Underline(camel));
String sql = "Select * FROM demo WHERE 1=1 and dLoginName ='dLoginName' or ID =2"; // "aEd,aWdEw";
System.out.println(camelToUnderline(sql));
System.out.println(zMOrSZ("a"));
// System.out.println(zMOrSZ(sql));
}
}
字符串工具类
原创
©著作权归作者所有:来自51CTO博客作者你的阿冷丶的原创作品,请联系作者获取转载授权,否则将追究法律责任
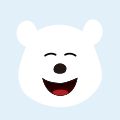
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
Java-长字符串加密
加密:为你的长字符串提供最高级别的保护!!!
加密算法 JAVA -
字符串工具类总结(全)
现在是:2022年7月9日18:53:37最近在项目过程中整理了一些常用的方法,于是将其抽出来形成一个
java 大数据 String 工具类 字符串 -
字符串处理工具类
【代码】字符串处理工具类。
java 前端 算法 字符串 字符串转换 -
字符串随机生成工具类
package com.gblfy.util;import org.springframework.stereoty
字符串生成 java 随机数 i++ -
vue 判断是否存在sessionStorage
一个典型的stackoverflow风格的问题作为文章标题,以此避免挖空心思想名字的纠结。问题描述回到这个问题,常写模板的同学应该能心领神会需求的来源。举个简单例子,在三维数据处理中,经常会和空间点point打交道,point中会有很多种信息需要存储,比如空间坐标 x/y/z,比如法向信息 nx/ny/nz,比如颜色信息 R/G/B等,这些信息并非都是必须,在不同应用中会有不同的取舍
vue 判断文件是否存在 vue 判断是否存在 vue 判断服务器图片是否存在 vue判断图片是否存在 vue的change事件判断是否存在