import java.awt.*;
import java.awt.event.*;
import java.io.*;
import java.util.ArrayList;
import javax.swing.*;public class MoneyManager {
public static void main(String[] args) {
LoginFrame lf=new LoginFrame();
lf.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}//登录界面
class LoginFrame extends JFrame implements ActionListener{
private JLabel l_user,l_pwd; //用户名标签,密码标签
private JTextField t_user;//用户名文本框
private JPasswordField t_pwd; //密码文本框
private JButton b_ok,b_cancel; //登录按钮,退出按钮 public LoginFrame(){
super("欢迎登录财务系统");
l_user=new JLabel("用户名:",JLabel.RIGHT);
l_pwd=new JLabel(" 密码:",JLabel.RIGHT);
t_user=new JTextField(31);
t_pwd=new JPasswordField(31);
b_ok=new JButton("登录");
b_cancel=new JButton("退出");
//布局方式FlowLayout,一行排满排下一行
Container c=this.getContentPane();
c.setLayout(new FlowLayout());
c.add(l_user);
c.add(t_user);
c.add(l_pwd);
c.add(t_pwd);
c.add(b_ok);
c.add(b_cancel);
//为按钮添加监听事件
b_ok.addActionListener(this);
b_cancel.addActionListener(this); t_user.addKeyListener(new KeyAdapter() {
@Override
public void keyTyped(KeyEvent e) {
if(e.getKeyChar() ==KeyEvent.VK_ENTER){
try{
FileReader in=new FileReader("D:\\作业相关\\Java课程设计\\src\\pwd.txt");
BufferedReader re=new BufferedReader(in);
String s1=re.readLine();//账号
String s2=re.readLine();//密码
String pwd=new String(t_pwd.getPassword());
boolean flag1= t_user.getText().trim().equals(s1);
boolean flag2= pwd.trim().equals(s2);
if(!flag1){
JOptionPane.showMessageDialog(null,"用户名出错", "警告", JOptionPane.ERROR_MESSAGE);
}
if(!flag2){
JOptionPane.showMessageDialog(null,"密码出错", "警告", JOptionPane.ERROR_MESSAGE);
}
if(flag1&&flag2){
new MainFrame(t_user.getText().trim());//密码正确,进入登录界面
}
}catch(IOException error1){
System.out.println(""+error1);
}
}
}
});
t_pwd.addKeyListener(new KeyAdapter() {
@Override
public void keyTyped(KeyEvent e) {
if(e.getKeyChar() ==KeyEvent.VK_ENTER){
try{
FileReader in=new FileReader("D:\\作业相关\\Java课程设计\\src\\pwd.txt");
BufferedReader re=new BufferedReader(in);
String s1=re.readLine();//账号
String s2=re.readLine();//密码
String pwd=new String(t_pwd.getPassword());
boolean flag1= t_user.getText().trim().equals(s1);
boolean flag2= pwd.trim().equals(s2);
if(!flag1){
JOptionPane.showMessageDialog(null,"用户名出错", "警告", JOptionPane.ERROR_MESSAGE);
}
if(!flag2){
JOptionPane.showMessageDialog(null,"密码出错", "警告", JOptionPane.ERROR_MESSAGE);
}
if(flag1&&flag2){
new MainFrame(t_user.getText().trim());//密码正确,进入登录界面
}
}catch(IOException error1){
System.out.println(""+error1);
}
}
}
}); //界面大小不可调整
this.setResizable(false);
this.setSize(455,150); //界面显示居中
Dimension screen = this.getToolkit().getScreenSize();
this.setLocation((screen.width-this.getSize().width)/2,(screen.height-this.getSize().height)/2);
//this.show();
this.setVisible(true);
}
/*登录和退出按钮功能设计,对接口方法的实现*/
public void actionPerformed(ActionEvent e) {
if(b_cancel==e.getSource()){
System.exit(0);
}else if(b_ok==e.getSource()){
try{
FileReader in=new FileReader("D:\\作业相关\\Java课程设计\\src\\pwd.txt");
BufferedReader re=new BufferedReader(in);
String s1=re.readLine();//账号
String s2=re.readLine();//密码
String pwd=new String(t_pwd.getPassword());
boolean flag1= t_user.getText().trim().equals(s1);
boolean flag2= pwd.trim().equals(s2);
if(!flag1){
JOptionPane.showMessageDialog(null,"用户名出错", "警告", JOptionPane.ERROR_MESSAGE);
}
if(!flag2){
JOptionPane.showMessageDialog(null,"密码出错", "警告", JOptionPane.ERROR_MESSAGE);
}
if(flag1&&flag2){
new MainFrame(t_user.getText().trim());//密码正确,进入登录界面
}
}catch(IOException error1){
System.out.println(""+error1);
}
}
}
}
//主界面
class MainFrame extends JFrame implements ActionListener{
private JMenuBar mb=new JMenuBar();
private JMenu m_system=new JMenu("系统管理");
private JMenu m_fm=new JMenu("收支管理");
private JMenuItem mI[]={new JMenuItem("密码重置"),new JMenuItem("退出系统")};
private JMenuItem m_FMEdit=new JMenuItem("收支编辑");
private JLabel l_type,l_fromdate,l_todate,l_bal,l_ps;
private JTextField t_fromdate,t_todate;
private JButton b_select1,b_select2;
private JComboBox c_type;
private JPanel p_condition,p_detail;
private String s1[]={"收入","支出"};
private double bal1;
private JTable table;
private String username; public MainFrame(String username){
super(username+",欢迎使用个人理财账本!");
this.username=username;
Container c=this.getContentPane();
c.setLayout(new BorderLayout());
c.add(mb,"North");
mb.add(m_system);
mb.add(m_fm);
m_system.add(mI[0]);
m_system.add(mI[1]);
m_fm.add(m_FMEdit);
m_FMEdit.addActionListener(this);
mI[0].addActionListener(this);
mI[1].addActionListener(this); l_type=new JLabel("收支类型:");
c_type=new JComboBox(s1);
b_select1=new JButton("查询");
l_fromdate=new JLabel("起始时间");
t_fromdate=new JTextField(8);
l_todate=new JLabel("终止时间");
t_todate=new JTextField(8);
b_select2=new JButton("查询");
l_ps = new JLabel("注意:时间格式为YYYYMMDD,例如:20150901");
p_condition=new JPanel();
p_condition.setLayout(new GridLayout(3,1));
p_condition.setBorder(BorderFactory.createCompoundBorder(
BorderFactory.createTitledBorder("输入查询条件"),
BorderFactory.createEmptyBorder(5,5,5,5)));
JPanel p1 = new JPanel();
JPanel p2 = new JPanel();
JPanel p3 = new JPanel();
p1.add(l_type);
p1.add(c_type);
p1.add(b_select1);
p2.add(l_fromdate);
p2.add(t_fromdate);
p2.add(l_todate);
p2.add(t_todate);
p2.add(b_select2);
p3.add(l_ps);
p_condition.add(p1);
p_condition.add(p2);
p_condition.add(p3);
c.add(p_condition,"Center");
b_select1.addActionListener(this);
b_select2.addActionListener(this);
p_detail=new JPanel();
p_detail.setBorder(BorderFactory.createCompoundBorder(
BorderFactory.createTitledBorder("收支明细信息"),
BorderFactory.createEmptyBorder(5,5,5,5)));
l_bal=new JLabel();
String[] cloum = {"编号", "日期", "类型","内容","金额",};
Object[][] row = new Object[50][5];
table = new JTable(row, cloum);
JScrollPane scrollpane = new JScrollPane(table);
scrollpane.setPreferredSize(new Dimension(580,350));
scrollpane.setVerticalScrollBarPolicy(JScrollPane.VERTICAL_SCROLLBAR_ALWAYS);
scrollpane.setViewportView(table);
p_detail.add(l_bal);
p_detail.add(scrollpane);
c.add(p_detail,"South"); method m=new method();
m.showDetail(row);
bal1=0;
double b;
ArrayList<MyAccount> a1= new ArrayList<>();
a1=m.loadList(a1);
for(int i=0;i<a1.size();i++){
b=Double.parseDouble(a1.get(i).getAmount());
if(a1.get(i).getType().equals("收入")){
bal1+=b;
}
else{
bal1-=b;
}
}
if(bal1<0)
l_bal.setText("个人总收支余额为"+bal1+"元。您已超支,请适度消费!");
else
l_bal.setText("个人总收支余额为"+bal1+"元。"); this.setResizable(false);
this.setSize(600,580);
Dimension screen = this.getToolkit().getScreenSize();
this.setLocation((screen.width-this.getSize().width)/2,(screen.height-this.getSize().height)/2);
this.setVisible(true);
}
public MainFrame(String username,ArrayList<MyAccount> a1){
super(username+",欢迎使用个人理财账本!");
this.username=username;
Container c=this.getContentPane();
c.setLayout(new BorderLayout());
c.add(mb,"North");
mb.add(m_system);
mb.add(m_fm);
m_system.add(mI[0]);
m_system.add(mI[1]);
m_fm.add(m_FMEdit);
m_FMEdit.addActionListener(this);
mI[0].addActionListener(this);
mI[1].addActionListener(this); l_type=new JLabel("收支类型:");
c_type=new JComboBox(s1);
b_select1=new JButton("查询");
l_fromdate=new JLabel("起始时间");
t_fromdate=new JTextField(8);
l_todate=new JLabel("终止时间");
t_todate=new JTextField(8);
b_select2=new JButton("查询");
l_ps = new JLabel("注意:时间格式为YYYYMMDD,例如:20150901");
p_condition=new JPanel();
p_condition.setLayout(new GridLayout(3,1));
p_condition.setBorder(BorderFactory.createCompoundBorder(
BorderFactory.createTitledBorder("输入查询条件"),
BorderFactory.createEmptyBorder(5,5,5,5)));
JPanel p1 = new JPanel();
JPanel p2 = new JPanel();
JPanel p3 = new JPanel();
p1.add(l_type);
p1.add(c_type);
p1.add(b_select1);
p2.add(l_fromdate);
p2.add(t_fromdate);
p2.add(l_todate);
p2.add(t_todate);
p2.add(b_select2);
p3.add(l_ps);
p_condition.add(p1);
p_condition.add(p2);
p_condition.add(p3);
c.add(p_condition,"Center");
b_select1.addActionListener(this);
b_select2.addActionListener(this);
p_detail=new JPanel();
p_detail.setBorder(BorderFactory.createCompoundBorder(
BorderFactory.createTitledBorder("收支明细信息"),
BorderFactory.createEmptyBorder(5,5,5,5)));
l_bal=new JLabel();
String[] cloum = {"编号", "日期", "类型","内容","金额",};
Object[][] row = new Object[50][5];
table = new JTable(row, cloum);
JScrollPane scrollpane = new JScrollPane(table);
scrollpane.setPreferredSize(new Dimension(580,350));
scrollpane.setVerticalScrollBarPolicy(JScrollPane.VERTICAL_SCROLLBAR_ALWAYS);
scrollpane.setViewportView(table);
p_detail.add(l_bal);
p_detail.add(scrollpane);
c.add(p_detail,"South"); method m=new method();
m.showDetail(row,a1);
bal1=0;
double b;
for(int i=0;i<a1.size();i++){
b=Double.parseDouble(a1.get(i).getAmount());
if(a1.get(i).getType().equals("收入")){
bal1+=b;
}
else{
bal1-=b;
}
}
if(bal1<0)
l_bal.setText("个人总支出为"+bal1+"元");
else
l_bal.setText("个人总收入为"+bal1+"元"); this.setResizable(false);
this.setSize(600,580);
Dimension screen = this.getToolkit().getScreenSize();
this.setLocation((screen.width-this.getSize().width)/2,(screen.height-this.getSize().height)/2);
this.setVisible(true);
} public void actionPerformed(ActionEvent e) {
Object temp=e.getSource();
if(temp==mI[0]){
new ModifyPwdFrame(username);//重置密码
}else if(temp==mI[1]){
System.exit(0);//退出系统
}else if(temp==m_FMEdit){
new BalEditFrame(); //收支编辑
}else if(temp==b_select1){ //根据收支类型查询
this.dispatchEvent(new WindowEvent(this,WindowEvent.WINDOW_CLOSING));//关闭当前窗口
method m=new method();//对文件数据读取等操作
ArrayList<MyAccount> a1=new ArrayList<>();
a1=m.loadList(a1);//全部数据
ArrayList<MyAccount> a2=new ArrayList<>();//收入
ArrayList<MyAccount> a3=new ArrayList<>();//支出
for(int i=0;i<a1.size();i++){
if(a1.get(i).getType().equals("收入")){
a2.add(a1.get(i));
}else{
a3.add(a1.get(i));
}
}
if(c_type.getSelectedItem().toString().equals("收入")){
new MainFrame(username,a2);
}else{
new MainFrame(username,a3);
}
}else if(temp==b_select2){ //根据时间范围查询
this.dispatchEvent(new WindowEvent(this,WindowEvent.WINDOW_CLOSING));
method m=new method();
ArrayList<MyAccount> a1=new ArrayList<>();
a1=m.loadList(a1);
String start=t_fromdate.getText();
String end=t_todate.getText();
ArrayList<MyAccount> a2=new ArrayList<>();
for(int i=0;i<a1.size();i++){
if(a1.get(i).getDate().compareTo(start)>=0&&a1.get(i).getDate().compareTo(end)<=0){
a2.add(a1.get(i));
}
}
new MainFrame(username,a2);
}
}
}
//修改密码界面
class ModifyPwdFrame extends JFrame implements ActionListener{
private JLabel l_oldPWD,l_newPWD,l_newPWDAgain;
private JPasswordField t_oldPWD,t_newPWD,t_newPWDAgain;
private JButton b_ok,b_cancel;
private String username; public ModifyPwdFrame(String username){
super("修改密码");
this.username=username;
l_oldPWD=new JLabel("旧密码");
l_newPWD=new JLabel("新密码:");
l_newPWDAgain=new JLabel("确认新密码:");
t_oldPWD=new JPasswordField(15);
t_newPWD=new JPasswordField(15);
t_newPWDAgain=new JPasswordField(15);
b_ok=new JButton("确定");
b_cancel=new JButton("取消");
Container c=this.getContentPane();
c.setLayout(new FlowLayout());
c.add(l_oldPWD);
c.add(t_oldPWD);
c.add(l_newPWD);
c.add(t_newPWD);
c.add(l_newPWDAgain);
c.add(t_newPWDAgain);
c.add(b_ok);
c.add(b_cancel);
b_ok.addActionListener(this);
b_cancel.addActionListener(this);
this.setResizable(false);
this.setSize(280,160);
Dimension screen = this.getToolkit().getScreenSize();
this.setLocation((screen.width-this.getSize().width)/2,(screen.height-this.getSize().height)/2);
this.setVisible(true);
} public void actionPerformed(ActionEvent e) {
if(b_cancel==e.getSource()){
this.dispatchEvent(new WindowEvent(this,WindowEvent.WINDOW_CLOSING));
}else if(b_ok==e.getSource()){ //修改密码
boolean flag=true;
try{
BufferedReader re=new BufferedReader(new FileReader("D:\\作业相关\\Java课程设计\\src\\pwd.txt"));
String s1=re.readLine();
String s2=re.readLine();
if(!t_oldPWD.getText().trim().equals(s2)){
JOptionPane.showMessageDialog(null,"旧密码错误!!", "警告", JOptionPane.ERROR_MESSAGE);
flag=false;
}
if(t_newPWD.getText().trim().equals(s2)){
JOptionPane.showMessageDialog(null,"新旧一样,重新修改", "警告", JOptionPane.ERROR_MESSAGE);
flag=false;
}
if(t_newPWDAgain.getText().trim().equals(t_newPWD.getText().trim())&&flag){
PrintWriter p=new PrintWriter("D:\\作业相关\\Java课程设计\\src\\pwd.txt");
p.println(s1);
p.println(t_newPWD.getPassword());
p.close();
JOptionPane.showMessageDialog(null,"修改成功", "提醒", JOptionPane.ERROR_MESSAGE);
this.dispatchEvent(new WindowEvent(this, WindowEvent.WINDOW_CLOSING));
System.exit(0);
}else if(flag&& !t_newPWDAgain.getText().trim().equals(t_newPWD.getText())){
JOptionPane.showMessageDialog(null,"两次新密码不同,重改!", "警告", JOptionPane.ERROR_MESSAGE);
}
}catch(IOException ERROR){
System.out.println(""+ERROR);
} }
}
}
//收支编辑界面
class BalEditFrame extends JFrame implements ActionListener{
private JLabel l_id,l_date,l_bal,l_type,l_item;
private JTextField t_id,t_date,t_bal;
private JComboBox c_type,c_item;
private JButton b_update,b_delete,b_select,b_new,b_clear;
private JPanel p1,p2,p3;
private JScrollPane scrollpane;
private JTable table; public BalEditFrame(){
super("收支编辑" );
l_id=new JLabel("编号:");
l_date=new JLabel("日期:");
l_bal=new JLabel("金额:");
l_type=new JLabel("类型:");
l_item=new JLabel("内容:");
t_id=new JTextField(8);
t_date=new JTextField(8);
t_bal=new JTextField(8); String s1[]={"收入","支出"};
String s2[]={"购物","餐饮","居家","交通","娱乐","人情","工资","奖金","其他"};
c_type=new JComboBox(s1);
c_item=new JComboBox(s2); b_select=new JButton("查询");
b_update=new JButton("修改");
b_delete=new JButton("删除");
b_new=new JButton("录入");
b_clear=new JButton("清空"); Container c=this.getContentPane();
c.setLayout(new BorderLayout()); p1=new JPanel();
p1.setLayout(new GridLayout(5,2,10,10));
p1.setBorder(BorderFactory.createCompoundBorder(
BorderFactory.createTitledBorder("编辑收支信息"),
BorderFactory.createEmptyBorder(5,5,5,5)));
p1.add(l_id);
p1.add(t_id);
p1.add(l_date);
p1.add(t_date);
p1.add(l_type);
p1.add(c_type);
p1.add(l_item);
p1.add(c_item);
p1.add(l_bal);
p1.add(t_bal);
c.add(p1, BorderLayout.WEST); p2=new JPanel();
p2.setLayout(new GridLayout(5,1,10,10));
p2.add(b_new);
p2.add(b_update);
p2.add(b_delete);
p2.add(b_select);
p2.add(b_clear); c.add(p2,BorderLayout.CENTER);
p3=new JPanel();
p3.setBorder(BorderFactory.createCompoundBorder(
BorderFactory.createTitledBorder("显示收支信息"),
BorderFactory.createEmptyBorder(5,5,5,5))); String[] cloum = { "编号", "日期", "类型","内容", "金额"};
Object[][] row = new Object[50][5];
/*此处添加代码会造成查询功能失去作用,新建构造方法去实现编辑表中的查询功能,这个方法保证打开的时候为空*/
//method m=new method();
//m.showDetail(row); table = new JTable(row, cloum);
scrollpane = new JScrollPane(table);
scrollpane.setViewportView(table);
scrollpane.setVerticalScrollBarPolicy(JScrollPane.VERTICAL_SCROLLBAR_ALWAYS);
p3.add(scrollpane);
c.add(p3,BorderLayout.EAST); b_update.addActionListener(this);
b_delete.addActionListener(this);
b_select.addActionListener(this);
b_new.addActionListener(this);
b_clear.addActionListener(this);
/*table 添加鼠标点击响应*/
table.addMouseListener(new MouseAdapter() {
@Override
public void mouseClicked(MouseEvent e) {
int a=table.getSelectedRow();
t_id.setText(row[a][0].toString());
t_date.setText(row[a][1].toString());
c_type.setSelectedItem(row[a][2].toString());
c_item.setSelectedItem(row[a][3].toString());
t_bal.setText(row[a][4].toString());
}
}
);
this.setResizable(false);
this.setSize(800,300);
Dimension screen = this.getToolkit().getScreenSize();
this.setLocation((screen.width-this.getSize().width)/2,(screen.height-this.getSize().height)/2);
this.setVisible(true);
}
public BalEditFrame(ArrayList<MyAccount> a1){
super("收支编辑" );
l_id=new JLabel("编号:");
l_date=new JLabel("日期:");
l_bal=new JLabel("金额:");
l_type=new JLabel("类型:");
l_item=new JLabel("内容:");
t_id=new JTextField(8);
t_date=new JTextField(8);
t_bal=new JTextField(8); String s1[]={"收入","支出"};
String s2[]={"购物","餐饮","居家","交通","娱乐","人情","工资","奖金","其他"};
c_type=new JComboBox(s1);
c_item=new JComboBox(s2); b_select=new JButton("查询");
b_update=new JButton("修改");
b_delete=new JButton("删除");
b_new=new JButton("录入");
b_clear=new JButton("清空"); Container c=this.getContentPane();
c.setLayout(new BorderLayout()); p1=new JPanel();
p1.setLayout(new GridLayout(5,2,10,10));
p1.setBorder(BorderFactory.createCompoundBorder(
BorderFactory.createTitledBorder("编辑收支信息"),
BorderFactory.createEmptyBorder(5,5,5,5)));
p1.add(l_id);
p1.add(t_id);
p1.add(l_date);
p1.add(t_date);
p1.add(l_type);
p1.add(c_type);
p1.add(l_item);
p1.add(c_item);
p1.add(l_bal);
p1.add(t_bal);
c.add(p1, BorderLayout.WEST); p2=new JPanel();
p2.setLayout(new GridLayout(5,1,10,10));
p2.add(b_new);
p2.add(b_update);
p2.add(b_delete);
p2.add(b_select);
p2.add(b_clear);
c.add(p2,BorderLayout.CENTER);
p3=new JPanel();
p3.setBorder(BorderFactory.createCompoundBorder(
BorderFactory.createTitledBorder("显示收支信息"),
BorderFactory.createEmptyBorder(5,5,5,5)));
String[] cloum = { "编号", "日期", "类型","内容", "金额"};
Object[][] row = new Object[50][5];
method m=new method();
m.showDetail(row,a1);
table = new JTable(row, cloum);
scrollpane = new JScrollPane(table);
scrollpane.setViewportView(table);
scrollpane.setVerticalScrollBarPolicy(JScrollPane.VERTICAL_SCROLLBAR_ALWAYS);
p3.add(scrollpane);
c.add(p3,BorderLayout.EAST); b_update.addActionListener(this);
b_delete.addActionListener(this);
b_select.addActionListener(this);
b_new.addActionListener(this);
b_clear.addActionListener(this);
/*table 添加鼠标点击响应*/
table.addMouseListener(new MouseAdapter() {
@Override
public void mouseClicked(MouseEvent e) {
int a=table.getSelectedRow();
t_id.setText(row[a][0].toString());
t_date.setText(row[a][1].toString());
c_type.setSelectedItem(row[a][2].toString());
c_item.setSelectedItem(row[a][3].toString());
t_bal.setText(row[a][4].toString());
}
}
);
this.setResizable(false);
this.setSize(800,300);
Dimension screen = this.getToolkit().getScreenSize();
this.setLocation((screen.width-this.getSize().width)/2,(screen.height-this.getSize().height)/2);
this.setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if(b_select==e.getSource()){ //查询所有收支信息
this.dispatchEvent(new WindowEvent(this,WindowEvent.WINDOW_CLOSING));
method m= new method();
ArrayList<MyAccount> a1=new ArrayList<>();
a1=m.loadList(a1);
new BalEditFrame(a1);
}else if(b_update==e.getSource()){ // 修改某条收支信息
int op=0;
if(op==JOptionPane.showConfirmDialog(null,"确定修改?", "提示", JOptionPane.ERROR_MESSAGE)){
method m=new method();
ArrayList<MyAccount> a1=new ArrayList<>();
a1=m.loadList(a1);
int b=Integer.parseInt(t_id.getText());
for(int i=0;i<a1.size();i++){
if(a1.get(i).getId().equals(t_id.getText())){
b=i;
break;
}
}
a1.remove(b);
a1.add(b,new MyAccount(t_id.getText(),t_date.getText(),c_type.getSelectedItem().toString(),c_item.getSelectedItem().toString(),t_bal.getText()));
this.dispatchEvent(new WindowEvent(this,WindowEvent.WINDOW_CLOSING));
m.update(a1);
m.delete();
a1=m.loadList(a1);
new BalEditFrame(a1);
}
}else if(b_delete==e.getSource()){ //删除某条收支信息
method m=new method();
ArrayList<MyAccount> a1=new ArrayList<>();
a1=m.loadList(a1);
int b=0;
for(int i=0;i<a1.size();i++){
if(a1.get(i).getId().equals(t_id.getText())){
b=i;
break;
}
}
a1.remove(b);
this.dispatchEvent(new WindowEvent(this,WindowEvent.WINDOW_CLOSING));
m.delete();
m.update(a1);
new BalEditFrame(a1);
}else if(b_new==e.getSource()){ //新增某条收支信息
method m=new method();
ArrayList<MyAccount> a1=new ArrayList<>();
a1=m.loadList(a1);
int flag=1;
for(int i=0;i<a1.size();i++) {
if(t_id.getText().equals(a1.get(i).getId())){
flag=0;
break;
}
}
String s1=t_date.getText();
String regex="[^0-9]+";
if(s1.matches(regex)){
flag=2;
}else{
if(s1.length()!=8){
flag=2;
}
int year=Integer.parseInt(s1.substring(0,4));
int month=Integer.parseInt(s1.substring(4,6));
int day=Integer.parseInt(s1.substring(6,8));
if(year<1970||month<=0||month>12||year>=2022){
flag=2;
}
if(month==1||month==3||month==5||month==7||month==8||month==10||month==12){
if(day<=0||day>31){
flag=2;
}
}
if(month==2&&(year%4==0&&year%100!=0||year%400==0)){
if(day<=0||day>29){
flag=2;
}
}
if(month==2&&!(year%4==0&&year%100!=0||year%400==0)){
if(day<=0||day>28){
flag=2;
}
}
if(month==4||month==6||month==9||month==11){
if(day<=0||day>30){
flag=2;
}
}
}
if(flag==1) {
a1.add(new MyAccount(t_id.getText(),t_date.getText(),c_type.getSelectedItem().toString(),c_item.getSelectedItem().toString(),t_bal.getText()));
}else if(flag==0){
JOptionPane.showMessageDialog(null,"id重复,重新编辑", "警告", JOptionPane.ERROR_MESSAGE);
}
else {
JOptionPane.showMessageDialog(null,"日期错误,重新编辑", "警告", JOptionPane.ERROR_MESSAGE);
}
this.dispatchEvent(new WindowEvent(this,WindowEvent.WINDOW_CLOSING));
m.delete();
m.update(a1);
new BalEditFrame(a1);
}else if(b_clear==e.getSource()){ //清空输入框
method m=new method();
ArrayList<MyAccount> a1=new ArrayList<>();
a1=m.loadList(a1);
this.dispatchEvent(new WindowEvent(this,WindowEvent.WINDOW_CLOSING));
new BalEditFrame(a1);
}
}
}//主界面中的row数组赋值
class method{
public Object showDetail(Object row[][]) {
ArrayList<MyAccount> a1= new ArrayList<>();
try {
FileInputStream fis=new FileInputStream("D:\\作业相关\\Java课程设计\\src\\data.txt");
ObjectInputStream ois=new ObjectInputStream(fis);
a1=(ArrayList<MyAccount>)(ois.readObject());
ois.close();
fis.close();
} catch (Exception e) {
System.out.println(""+e);
}
for(int i=0;i<a1.size();i++) {
row[i][0]=a1.get(i).getId();
row[i][1]=a1.get(i).getDate();
row[i][2]=a1.get(i).getType();
row[i][3]=a1.get(i).getContent();
row[i][4]=a1.get(i).getAmount();
}
return row;
} //对主界面构造函数数组赋值
public Object showDetail(Object row[][],ArrayList<MyAccount> al) {
for(int i=0;i<al.size();i++) {
row[i][0]=al.get(i).getId();
row[i][1]=al.get(i).getDate();
row[i][2]=al.get(i).getType();
row[i][3]=al.get(i).getContent();
row[i][4]=al.get(i).getAmount();
}
return row;
}
//加载目标文件信息,并以ArrayList<MyAccount>形式返回
public ArrayList<MyAccount> loadList(ArrayList<MyAccount> a1) {
try {
FileInputStream fis= new FileInputStream("D:\\作业相关\\Java课程设计\\src\\data.txt");
ObjectInputStream ois=new ObjectInputStream(fis);
a1=(ArrayList<MyAccount>)(ois.readObject());
ois.close();
fis.close();
} catch (Exception e) {
System.out.println(""+e);
}
return a1;
}
public void delete(){
File f=new File("D:\\作业相关\\Java课程设计\\src\\data.txt");
f.delete();
}
//创建新文件并写入信息
public void update(ArrayList<MyAccount> a1){
try {
File f=new File("D:\\作业相关\\Java课程设计\\src\\data.txt");
FileOutputStream fos=new FileOutputStream(f);
ObjectOutputStream oos=new ObjectOutputStream(fos);
oos.writeObject(a1);
oos.close();
fos.close();
} catch (Exception e) {
System.out.println(""+e);
}
}
}
//序列化数据,便于适用线性表
class MyAccount implements Serializable{
private String id;
private String date;
private String type;
private String content;
private String amount;
public MyAccount(String id, String date, String type, String content, String amount) {
super();
this.id = id;
this.date = date;
this.type = type;
this.content = content;
this.amount = amount;
}
public String getId() {
return id;
}
public String getDate() {
return date;
}
public String getType() {
return type;
}
public String getContent() {
return content;
}
public String getAmount() {
return amount;
}
}
Java项目财务统计报表系统 基于java的财务管理系统
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
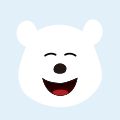
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
【java】学生管理系统
简单实现学生管理系统
学生管理系统 增删改查 登录注册 -
基于ElementUI+html和VUE实现的java后台管理系统
基于Html+Elementui实现的java后台管理框架
Vue ELementUI+html Springboot Jfinal Shiro登录验证 -
财务管理系统|基于Springboot开发实现公司财务管理系统
本系统是基于SpringBoot的公司财务系统,初步分为四个部分。管理员,财务,部门领导以及普通
spring boot java 公司财务管理系统 springboot财务管理 企业财务管理 -
财务管理系统java 高级 最新的财务管理系统财务管理系统java 高级 课程设计 spring boot vue.js 功能模块