python bytes对于刚接触python的小伙伴来讲,可能还是有点陌生!bytes是字节序列,值得注意的是它有取值范围:0 <= bytes <= 255;
一.bytes函数简介
python bytes字节序列有以下几种使用方式:
""" bytes(iterable_of_ints) ->bytes
bytes(string, encoding[, errors]) ->bytes
bytes(bytes_or_buffer)->immutable copy of bytes_or_buffer
bytes(int) -> bytes object of size given by the parameter initialized with nullbytes
bytes()-> empty bytes objectConstruct an immutable of bytesfrom:- an iterable yielding integers in range(256)- a text string encoded usingthe specified encoding- any objectimplementing the buffer API.-an integer
# (copiedfrom classdoc)"""#1.定义空的字节序列bytes
bytes()->empty bytes
#2.定义指定个数的字节序列bytes,默认以0填充,不能是浮点数
bytes(int) -> bytes of size given by the parameter initialized with nullbytes
#3.定义指定内容的字节序列bytes
bytes(iterable_of_ints)
#4.定义字节序列bytes,如果包含中文的时候必须设置编码格式
bytes(string, encoding[, errors]) -> immutable copy of bytes_or_buffer
返回值 : 返回一个新的字节序列,字节序列bytes有一个明显的特征,输出的时候最前面会有一个字符b标识,举个例子:
b'\x64\x65\x66'b'i love you'b'shuopython.com'
凡是输出前面带有字符b标识的都是字节序列bytes;
二.bytes函数使用
1.定义空的字节序列bytes
# !usr/bin/env python
#-*- coding:utf-8 _*-
"""@Author:何以解忧
@Blog(个人博客地址): shuopython.com
@WeChat Official Account(微信公众号):猿说python
@Github:www.github.com
@File:python_bytes.py
@Time:2020/2/25 21:25@Motto:不积跬步无以至千里,不积小流无以成江海,程序人生的精彩需要坚持不懈地积累!"""
if __name__ == "__main__":
a=bytes()
print(a)
print(type(a))
输出结果:
b''
2.定义指定个数的字节序列bytes,默认以0填充,不能是浮点数
if __name__ == "__main__":
b1= bytes(10)
print(b1)
print(type(b1))
# bytes 通过 decode函数转为 str类型
s1=b1.decode()
print("s1:",s1)
print(type(s1))
输出结果:
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'
s1:
3.定义指定内容的字节序列bytes,只能是整数类型的序列,否则异常
if __name__ == "__main__":
# 正常输出
b1= bytes([1, 2, 3, 4])>>> b'\x01\x02\x03\x04'# bytes字节序列必须是0 ~ 255之间的整数,不能含有float类型
b1= bytes([1.1, 2.2, 3, 4])>>> TypeError: 'float' object cannot be interpreted asan integer
# bytes字节序列必须是0 ~ 255之间的整数,不能含有str类型
b1= bytes([1, 'a', 2, 3])>>> TypeError: 'str' object cannot be interpreted asan integer
# bytes字节序列必须是0 ~ 255之间的整数,不能大于或者等于256
b1= bytes([1, 257])>>> ValueError: bytes must be in range(0, 256)
4.定义个字节序列bytes
if __name__ == "__main__":
b1= bytes('abc', 'utf-8') # 如果包含中文必须设置编码格式
print(b1)
print("***"*20)
b2= bytes(b'def')
print(b2)
print(type(b2))
print(id(b2))
print("***" * 20)
b3= b'\x64\x65\x66'print(b3)
print(type(b3))
print(id(b3))
print("***" * 20)
# result= True if b2 == b3 elseFalse
print("b == bb 的结果是",(b2 ==b3))
print("b is bb 的结果是", (b2 is b3))
输出:
b'abc'
************************************************************b'def'
2563018794448
************************************************************b'def'
2563018794448
************************************************************b==bb 的结果是 True
bis bb 的结果是 True
注意:
1.python is和==的区别文章中有详细介绍:==是python标准操作符中的比较操作符,用来比较判断两个对象的value(值)是否相等,例如下面两个字符串间的比较;
2.is也被叫做同一性运算符,这个运算符比较判断的是对象间的唯一身份标识,也就是id是否相同;
3.如果bytes初始化含有中文的字符串必须设置编码格式,否则报错:TypeError: string argument without an encoding,如下:
b = bytes("猿说python")>>> b = bytes("猿说python")>>> TypeError: string argument without an encoding
三.重点提醒
1.bytes字节序列的取值范围:必须是0~255之间的整数;
2.bytes字节序列是不可变序列:bytes是不可变序列,即和str类型一样不可修改,如果通过find()、replace()、islower()等函数修改,其实是创建了新的bytes、str对象,可以通过内置函数id()查看值 是否发生变化,示例如下:
if __name__ == "__main__":
#1.通过 replace 生成新的bytes字节序列
b1= bytes(b"abcdefg")
print(b1)
print(type(b1))
print(id(b1))
print("***" * 20)
b2= bytes.replace(b1,b"cd",b"XY")
print(b2)
print(type(b2))
print(id(b2))
print("***" * 20)
#2.bytes 是不可变序列,不能直接修改bytes的内容
b1[0] = b"ss"
>>> TypeError: 'bytes' object does not support item assignment
输出结果:
b'abcdefg'
2264724270976
************************************************************b'abXYefg'
2264707281104
************************************************************
bytes python 计算校验和 python中bytes函数怎么用
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
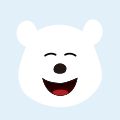
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
Python bytes() 函数
Python内置函数是Python编程语言中预先定义的函数。嵌入到主调函数中的函数称为内置函
内置函数 python python编程