1、vue插件
v-bind:实现点击不同按钮切换不同的属性值,使用class属性中的特殊用法实现一个按钮切换北京颜色,例如<img src=" " height=" "/>,在其中src和height的值如果不想写死,而是想获取vue实例中的属性值的话,就可以通过v-bind实现,如<img v-bind:src="vue实例中的数据属性名" :height="vue实例中的数据属性名"/>。
实例:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>v-bind绑定</title>
</head>
<body>
<script src="../node_modules/vue/dist/vue.js"></script>
<div id="app">
<button @click="color='red'">红色</button>
<button @click="color='blue'">蓝色</button>
<div :class="color">
点击按钮改变背景颜色
</div>
<hr>
<br>
<button @click="bool=!bool">点我改变下面色块的颜色</button>
<div :class="{red:bool,blue:!bool}">
点击按钮改变背景颜色
</div>
</div>
<script type="text/javascript">
new Vue({
el:"#app",
data:{
color:"red",
bool:true
}
})
</script>
</body>
</html>
计算属性的使用
计算属性主要是实现将日期转换为yyyy-MM-dd格式字符串,主要是使用computed计算属性里面的方法进行处理.
实例:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>v-bind绑定</title>
</head>
<body>
<script src="../node_modules/vue/dist/vue.js"></script>
<div id="app">
<h2>
你的生日是:
{{new Date(birthday).getFullYear()}}-{{new Date(birthday).getMonth()+1}}-
{{new Date(birthday).getDay()}}
</h2>
<hr>
<h2>
你的生日是:
{{birth}}
</h2>
</div>
<script type="text/javascript">
new Vue({
el:"#app",
data:{
birthday:1429032123201
},
computed:{
birth(){
const data=new Date(this.birthday);
return data.getFullYear()+"-"+
(data.getMonth()+1)+"-"+data.getDay();
}
}
});
</script>
</body>
</html>
说明:computed计算属性的应用场景:可以应用在差值或者指令表达式复杂的时候,将一些属性数据经过方法处理之后返回.
watch基本和深度监控
watch的使用场景:在vue实例中数据属性在页面中修改产生了变化,就可以通过watch监控获取数据对象,如果是修改对象的数据属性,就可以开启深度监控获取修改之后最新的数据对象
实例:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>v-bind绑定</title>
</head>
<body>
<script src="../node_modules/vue/dist/vue.js"></script>
<div id="app">
<input type="text" v-model="message">
<hr><br>
<input type="text" v-model="person.name"><br>
<input type="text" v-model="person.age">
<button @click="person.age++">+</button>
<h2>
姓名为:{{person.name}},年龄为:{{person.age}}
</h2>
</div>
<script type="text/javascript">
new Vue({
el:"#app",
data:{
message:"olivia",
person:{"name":"haha","age":8}
},
watch:{
message(newValue,oldValue){
console.log("新值:"+newValue+";旧值"+oldValue);
},
person: {
// 开启深度监控,监控对象中属性变化
deep: true,
// 可以获取最新的对象属性数据
handler(obj){
console.log("name="+obj.name+"; age="+obj.age);
}
}
},
});
</script>
</body>
</html>
watch使用主要是可以监控视图中数据的变化而做出响应,比如下拉框列表中,当如果选择了对于下拉框选项之后,就要根据最新的值去加载一些其他数据.
2.组件使用
组件主要将通用或公用的页面模板抽取成为vue组件,并且在vue实例当中引用
实例:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>组件测试</title>
</head>
<body>
<script src="../node_modules/vue/dist/vue.js"></script>
<div id="app">
<!--使用组件-->
<counter></counter>
<counter></counter>
<counter></counter>
</div>
<script>
// 定义组件
const counter={
template:"<button @click='num++'>你点击了{{num}}次</button>,",
data(){
return {num:0}
}
};
// 全局注册组件:在所有的vue实例当中都可以使用组件
//参数1:组件名称,参数2:具体组件
//vue.component("counter", counter);
new Vue({
el:"#app",
components:{
counter: counter
}
})
</script>
</body>
</html>
组件使用场景:当项目中需要重用某个模块(头部,尾部,新闻...)等,可以将模块抽取为组件,在其他页面当中注册使用
1.全局注册:在任何vue实例当中都可以引用,比如一般的网站的头部导航菜单
2.局部注册:可以在有需要的页面上引入组件,比如商城网站首页页面中的各种活动模块
父组件向子组件通信
其主要是实现父子组件之间的数据交换,能够及时更新组件内容
父组件将简单的字符串更新传递到子组件当中
实例:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>父子组件之间的通信</title>
</head>
<body>
<script src="../node_modules/vue/dist/vue.js"></script>
<div id="app">
<!--使用组件-->
<introduce :title="msg"></introduce>
</div>
<script>
// 定义组件
const introduce={
template:"<h2>{{title}}</h2>",
//定义接收父组件的属性
props:["title"]
};
//全局祖册组件:在所有的vue实例中都可以使用组件
//参数1:组件名称,参数2:具体组件
Vue.component("introduce",introduce);
new Vue({
el:"#app",
data:{
msg:"父组件的msg属性数据内容"
}
})
</script>
</body>
</html>
父组件将数组更新传递到子组件中:
实例:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>组件通信</title>
</head>
<body>
<script src="../node_modules/vue/dist/vue.js"></script>
<div id="app">
<!--使用组件-->
<my-list :items="lessons"></my-list>
</div>
<script>
//定义组件
const myList={
template:
' <ul>\n' +
' <li v-for="item in items" :key="item.id">{{item.id}}--{{item.name}}</li>\n' +
'</ul>',
//定义接收父组件的属性
props:{
items:{
//数据类型,如果是数组则是Array,结果是对象则是object
type:Array,
// 默认值
default:[]
}
}
};
new Vue({
el:"#app",
data:{
msg:"父组件的msg属性数据内容",
lessons:[
{"id":1,"name":"java"},
{"id":2,"name":"php"},
{"id":3,"name":"js"},
]
},
components:{
myList
}
})
</script>
</body>
</html>
父组件将数组更新传递到子组件当中
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>父组件将数组更新传递到子组件当中</title>
</head>
<body>
<script src="../node_modules/vue/dist/vue.js"></script>
<div id="app">
<!--使用组件-->
<my-list v-bind:p="person" ></my-list>
</div>
<script>
// 定义组件
const myList={
template:`
<span>{{p.name}}:{{p.age}}</span>
`,
// 定义接收父组件的属性
props:{
p:{
//数据类型,如果是数组则是Array,对象则是object
type:Object,
//默认值
default(){
return {name: "",age: 0};
}
}
}
};
new Vue({
el:"#app",
data:{
msg:"父组件的msg属性数据内容",
person:{name:"zhangsan",age:12}
},
components:{
myList
}
})
</script>
</body>
</html>
子组件向父组件通信
主要实现在子组件中点击对应按钮实现父组件属性数据改变
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>子组件向父组件通信</title>
</head>
<body>
<script src="../node_modules/vue/dist/vue.js"></script>
<div id="app">
<h2>num = {{num}}</h2>
<!--使用组件-->
<counter @plus="numPlus" @reduce="numReduce" :snum="num"></counter>
</div>
<script>
// 定义组件
const counter={
template:`
<div>
<button @click='incrNum'>+</button>
<button @click='decrNum'>-</button>
</div>
`,
props:["snum"],
methods:{
//递增
incrNum(){
//调用到父组件中的方法
return this.$emit("plus");
},
decrNum(){
//调用到父组件中的方法
return this.$emit("reduce");
}
}
};
//全局注册组件,在所有的vue实例中都可以使用组件
//参数1:组件名称,参数2:具体的组件
//Vue.component("counter",counter);
var app=new Vue({
el:"#app",
components:{
counter:counter
},
data:{
num:0
},
methods: {
numPlus(){
this.num++;
},
numReduce(){
this.num--;
}
}
});
</script>
</body>
</html>
3.axios方法及get,post方法
通过使用axios方法获取数据并在页面上将数据遍历显示.通过get,post方法实现数据加载,同时获取对应服务器上的数据,如果不是同一个服务器则需要在对应的服务器上面配置跨域,解决该问题.
实例;
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>axios</title>
</head>
<body>
<script src="../node_modules/vue/dist/vue.js"></script>
<script src="js/axios.min.js"></script>
<div id="app">
<ul>
<li v-for="(user, index) in users" :key="index">
{{index}}--{{user.name}}--{{user.age}}--{{user.gender}}
</li>
</ul>
</div>
<script>
new Vue({
el:"#app",
data:{
users:[]
},
created(){
//初始化数据
axios.post("data.json").then(res=>{
console.log(res);
//将数据赋值到vue实例数据属性users;
//如果使用的是箭头函数就可以使用this,this表示new
//vue组件
this.users=res.data;
}).catch(err=>alert(err));
//初始化加载数据
axios.post("data.json").then(
function (res) {
console.log(res);
//将数据赋值到vue实例中的数据属性users;
//如果使用的是匿名函数,不能使用this,在axios回调
//表示函数本身,不是vue实例
app.users=res.data;
}
).catch(err=>alert(err))
}
})
</script>
</body>
</html>
vue 修改nprogress 颜色 vue改变背景颜色
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
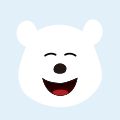
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
Python获取颜色RGB值
Python获取颜色RGB值
Python 图像处理 屏幕截图 -
javascript改变背景颜色 js改变背景颜色的代码
今天学习了使用JS调节背景色。结合HTML5的 input标签中的 range 类型。截图: 代码:<!DOCTYPE HTML> <html> <head> <meta charset="utf-8"> <title>JS调节背景色</title> <script src
javascript改变背景颜色 html5 css3 javascript 背景色