import os, sys, time
users = dict()
articals = dict()
def regist():
while True:
os.system("cls")
print("* ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ *")
print("* ~ * ~ * ~ *\t\t\t个人博客注册界面\t\t\t* ~ * ~ * ~ *")
print("* ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ *")
uaerName = input("\t\t\t请输入账号(R/r键返回): ")
if uaerName == 'R' or uaerName == 'r':
break
if uaerName in users:
input("该用户已存在,重新设置...")
continue
passWord = input("\t\t\t请输入密码: ")
confirm = input("\t\t\t请确认密码: ")
if passWord != confirm:
input("\t\t\t两次输入密码不同,任意键继续...")
continue
user = {"username": uaerName,"password": passWord,"nickname": "None"}
users[uaerName] = user
input("\t\t\t注册成功,任意键返回...")
break
def login():
while True:
os.system("cls")
print("* ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ *")
print("* ~ * ~ * ~ *\t\t\t个人博客登录界面\t\t\t* ~ * ~ * ~ *")
print("* ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ *")
uaerName = input("\t\t\t账号(R/r键返回): ")
if uaerName == 'R' or uaerName == 'r':
break
passWord = input("\t\t\t密码: ")
if uaerName in users and users[uaerName]['password'] == passWord:
print("\t\t\t登录中...")
time.sleep(1)
main(uaerName)
else:
input("\t\t\t账号或密码错误,请重新输入...")
continue
def modify(userName):
result = False
while True:
os.system("cls")
print("* ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ *")
print("* ~ * ~ * ~ *\t\t\t 修改登录密码 \t\t\t* ~ * ~ * ~ *")
print("* ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ *")
oldpass = input("\t\t\t原密码(R/r键返回):")
if oldpass == 'R' or oldpass == 'r':
break
if users[userName]['password'] != oldpass:
input("\t\t\t原密码输入有误,任意键继续...")
continue
newpass = input("\t\t\t新密码:")
confirm = input("\t\t\t确认密码:")
if newpass != confirm:
input("\t\t\t两次输入新密码不一致,任意键继续...")
continue
users[userName]['password'] = newpass
input("\t\t\t修改密码成功,请重新登录...")
result = True
break
return result
def update(userName):
while True:
os.system("cls")
print("* ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ *")
print("* ~ * ~ * ~ *\t\t\t 完善个人信息 \t\t\t* ~ * ~ * ~ *")
print("* ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ *")
username = userName
print("------------》\t\t\t用户名:{}".format(username))
userpass = users[username]['password']
nickname = input("------------》\t\t\t昵 称(R/r键退出):")
if nickname == 'R' or nickname == 'r':
input("------------》\t\t\t信息尚未完善,任意键退出...")
break
usersex = input("------------》\t\t\t性 别(R/r键退出):")
if usersex == 'R' or usersex == 'r':
input("------------》\t\t\t信息尚未完善,任意键退出...")
break
userage = input("------------》\t\t\t年 龄(R/r键退出):")
if userage == 'R' or userage == 'r':
input("------------》\t\t\t信息尚未完善,任意键退出...")
break
userhobby = input("------------》\t\t\t爱 好(R/r键退出):")
if userhobby == 'R' or userhobby == 'r':
input("------------》\t\t\t信息尚未完善,任意键退出...")
break
is_save = input("------------》\t\t\t是否保存(y/n)?")
if is_save == 'y':
user = {"username": username, "password": userpass, "nickname": nickname, "usersex": usersex, "userage": userage, "userhobby": userhobby}
users.pop(username)
users[username] = user
print("* ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ *")
input("------------》\t\t\t信息完善成功,任意键继续...")
else:
input("------------》\t\t\t信息尚未完善,任意键继续...")
break
def find(userName):
os.system("cls")
print("* ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ *")
print("* ~ * ~ * ~ *\t\t\t 个人信息 \t\t\t* ~ * ~ * ~ *")
print("* ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ *")
print("------------》\t\t\t用户名:{}".format(userName))
print("------------》\t\t\t昵 称:{}".format(users[userName]['nickname']))
if 'usersex' in users[userName]:
print("------------》\t\t\t性 别:{}".format(users[userName]['usersex']))
if 'userage' in users[userName]:
print("------------》\t\t\t年 龄:{}".format(users[userName]['userage']))
if 'userhobby' in users[userName]:
print("------------》\t\t\t爱 好:{}".format(users[userName]['userhobby']))
print("* ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ *")
input("------------》\t\t\t任意键继续...")
def main(userName):
while True:
os.system("cls")
print("* ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ *")
print("* ~ * ~ * ~ *\t\t\t 个人博客主页 \t\t\t* ~ * ~ * ~ *")
print("* ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ *")
print("* ~ * ~ * ~ *\t\t\t1. 个人资料维护\t\t\t* ~ * ~ * ~ *")
print("* ~ * ~ * ~ *\t\t\t2. 文章数据维护\t\t\t* ~ * ~ * ~ *")
print("* ~ * ~ * ~ *\t\t\t3. 注销登录 \t\t\t* ~ * ~ * ~ *")
print("* ~ * ~ * ~ *\t\t\t4. 退出系统 \t\t\t* ~ * ~ * ~ *")
print("* ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ *")
choices = input("\t\t\t请输入您的选择: ")
choice = int(choices)
if choice == 1:
if info(userName):
break
elif choice == 2:
artical(userName)
elif choice == 3:
input("\t\t\t系统注销中...")
break
elif choice == 4:
input("\t\t\t欢迎下次光临...")
sys.exit(1)
else:
input("\t\t\t选项有误,按任意键重新输入...")
os.system("cls")
continue
def info(userName):
result = False
while True:
os.system("cls")
print("* ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ *")
print("* ~ * ~ * ~ *\t\t\t 个人资料维护 \t\t\t* ~ * ~ * ~ *")
print("* ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ *")
print("* ~ * ~ * ~ *\t\t\t1. 查看个人信息\t\t\t* ~ * ~ * ~ *")
print("* ~ * ~ * ~ *\t\t\t2. 修改登录密码\t\t\t* ~ * ~ * ~ *")
print("* ~ * ~ * ~ *\t\t\t3. 完善个人资料\t\t\t* ~ * ~ * ~ *")
print("* ~ * ~ * ~ *\t\t\t4. 返回上一级 \t\t\t* ~ * ~ * ~ *")
print("* ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ *")
choices = input("\t\t\t请输入您的选择: ")
choice = int(choices)
if choice == 1:
find(userName)
elif choice == 2:
res = modify(userName)
if res:
result = res
break
elif choice == 3:
update(userName)
elif choice == 4:
break
else:
input("\t\t\t选项有误,按任意键重新输入...")
os.system("cls")
return result
def artical(userName):
while True:
os.system("cls")
print("* ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ *")
print("* ~ * ~ * ~ *\t\t\t 文章数据维护 \t\t\t* ~ * ~ * ~ *")
print("* ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ *")
print("* ~ * ~ * ~ *\t\t\t1. 发表文章 \t\t\t* ~ * ~ * ~ *")
print("* ~ * ~ * ~ *\t\t\t2. 查看个人文章\t\t\t* ~ * ~ * ~ *")
print("* ~ * ~ * ~ *\t\t\t3. 查看所有文章\t\t\t* ~ * ~ * ~ *")
print("* ~ * ~ * ~ *\t\t\t4. 返回上一级 \t\t\t* ~ * ~ * ~ *")
print("* ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ *")
choices = input("\t\t\t请输入您的选择: ")
choice = int(choices)
if choice == 1:
publish_artical(userName)
elif choice == 2:
personal_artical(userName)
elif choice == 3:
all_artical()
elif choice == 4:
break
else:
input("\t\t\t选项有误,按任意键重新输入...")
os.system("cls")
def publish_artical(userName):
result = False
while True:
os.system("cls")
print("* ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ *")
print("* ~ * ~ * ~ *\t\t\t 发表博客文章 \t\t\t* ~ * ~ * ~ *")
print("* ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ *")
print("------------》\t\t\t作者名:{}".format(userName))
sum = ''
artical_title = input("------------》\t\t\t文章标题(R/r键返回):")
if artical_title == 'R' or artical_title == 'r':
break
sum += artical_title + "\n"
for i in range(1, 50):
i = input("\t\t文章内容(R/r键结束):")
if i == 'R' or i == 'r':
result = True
break
else:
sum += '-----》' + i + '\n'
if result:
if userName in articals:
articals[userName].append(sum)
else:
group = list()
group.append(sum)
articals[userName] = group
input("\t\t博客发表成功,任意键继续...")
break
def personal_artical(userName):
os.system("cls")
print("* ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ *")
print("* ~ * ~ * ~ *\t\t\t 个人博客文章 \t\t\t* ~ * ~ * ~ *")
print("* ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ *")
if userName in articals:
for a in articals[userName]:
print("-----》", a)
input("-----》任意键返回...")
else:
input("------------》\t\t\t用户尚未发表博客,任意键继续...")
def all_artical():
os.system("cls")
print("* ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ *")
print("* ~ * ~ * ~ *\t\t\t 所有博客文章 \t\t\t* ~ * ~ * ~ *")
print("* ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ *")
if len(articals.values()):
for k, v in articals.items():
for i in v:
print("-----》\t\t\t作者:{}".format(k))
print("-----》", i)
input("-----》 任意键返回...")
else:
input("------------》\t\t\t未发现任何博客,任意键继续...")
def index():
while True:
os.system("cls")
print("* ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ *")
print("* ~ * ~ * ~ *\t\t\t欢迎进入个人博客\t\t\t* ~ * ~ * ~ *")
print("* ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ *")
print("* ~ * ~ * ~ *\t\t\t1. 登录个人博客\t\t\t* ~ * ~ * ~ *")
print("* ~ * ~ * ~ *\t\t\t2. 注册个人账号\t\t\t* ~ * ~ * ~ *")
print("* ~ * ~ * ~ *\t\t\t3. 退出系统 \t\t\t* ~ * ~ * ~ *")
print("* ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ *")
choices = input("\t\t\t请输入您的选择: ")
choice = int(choices)
if choice == 1:
login()
elif choice == 2:
regist()
elif choice == 3:
input("\t\t\t客观走好...")
sys.exit(1)
else:
input("\t\t\t选项有误,按任意键重新输入...")
os.system("cls")
index()
python编程博客 python个人博客网站
转载文章标签 python编程博客 python博客项目 个人博客 用户名 数据 文章分类 Python 后端开发
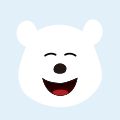
-
个人博客网站搭建
搭建步骤: 1、注册博客域名 2、购买服务器
服务器 域名解析