import java.io.BufferedReader;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStreamReader;
import sun.net.TelnetOutputStream;
import sun.net.TelnetInputStream;
import sun.net.ftp.FtpClient;
public class download {
String localfilename;
String remotefilename;
FtpClient ftpClient;
// server:服务器名字
// user:用户名
// password:密码
// path:服务器上的路径
public void connectServer(String ip, int port,String user
, String password,String path) {
try {
ftpClient = new FtpClient();
ftpClient.openServer(ip,port);
ftpClient.login(user, password);
System.out.println("login success!");
if (path.length() != 0) ftpClient.cd(path);
ftpClient.binary();
} catch (IOException ex) {
System.out.println("not login");
System.out.println(ex);
}
}
public void closeConnect() {
try {
ftpClient.closeServer();
System.out.println("disconnect success");
} catch (IOException ex) {
System.out.println("not disconnect");
System.out.println(ex);
}
}
public void upload() {
// 本地要长传文件名
this.localfilename = "D://test2//test.txt";
// 在ftp服务器上,要生成的文件名字。
this.remotefilename = "test.txt";
try {
TelnetOutputStream os = ftpClient.put(this.remotefilename);
java.io.File file_in = new java.io.File(this.localfilename);
FileInputStream is = new FileInputStream(file_in);
byte[] bytes = new byte[1024];
int c;
while ((c = is.read(bytes)) != -1) {
os.write(bytes, 0, c);
}
System.out.println("upload success");
is.close();
os.close();
} catch (IOException ex) {
System.out.println("not upload");
System.out.println(ex);
}
}
public void download() {
try {
TelnetInputStream is = ftpClient.get(this.remotefilename);
java.io.File file_in = new java.io.File(this.localfilename);
FileOutputStream os = new FileOutputStream(file_in);
byte[] bytes = new byte[1024];
int c;
while ((c = is.read(bytes)) != -1) {
// System.out.println((char)is.read());
// System.out.println(file_in);
os.write(bytes, 0, c);
}
System.out.println("download success");
os.close();
is.close();
} catch (IOException ex) {
System.out.println("not download");
System.out.println(ex);
}
}
public void download(String remotePath,String remoteFile,String localFile) {
try {
if (remotePath.length() != 0) ftpClient.cd(remotePath);
TelnetInputStream is = ftpClient.get(remoteFile);
java.io.File file_in = new java.io.File(localFile);
FileOutputStream os = new FileOutputStream(file_in);
byte[] bytes = new byte[1024];
int c;
while ((c = is.read(bytes)) != -1) {
// System.out.println((char)is.read());
// System.out.println(file_in);
os.write(bytes, 0, c);
}
System.out.println("download success");
os.close();
is.close();
} catch (IOException ex) {
System.out.println("not download");
System.out.println(ex);
}
}
public void download(String remoteFile,String localFile) {
// remoteFile是ftp服务器上的的文件路径文件。
// localFile 是 从ftp服务器上下载下来的文件,放在本机上的文件(名字随意定义)。
try {
TelnetInputStream is = ftpClient.get(remoteFile);
java.io.File file_in = new java.io.File(localFile);
FileOutputStream os = new FileOutputStream(file_in);
byte[] bytes = new byte[1024];
int c;
while ((c = is.read(bytes)) != -1) {
// System.out.println((char)is.read());
// System.out.println(file_in);
os.write(bytes, 0, c);
}
System.out.println("download success");
os.close();
is.close();
} catch (IOException ex) {
System.out.println("not download");
System.out.println(ex);
}
}
public static void main(String agrs[]) {
String filepath[] = { "/callcenter/index.jsp", "/callcenter/ip.txt",
"/callcenter/mainframe/image/processing_bar_2.gif",
"/callcenter/mainframe/image/logo_01.jpg" };
String localfilepath[] = { "C:\\FTP_Test\\index.jsp",
"C:\\FTP_Test\\ip.txt", "C:\\FTP_Test\\processing_bar_2.gif",
"C:\\FTP_Test\\logo_01.jpg" };
download fu = new download();
// fu.connectServer("59.151.113.85",22, "root", "cdel4321$#@!","/root/gklwb");
// 必须加上 "/"。
fu.connectServer("127.0.0.1",21, "jack", "123456","/ftp_test");
// 下载用的。
//for(int i=0;i<filepath.length;i++){
//fu.download(filepath[i],localfilepath[i]);
//}
// 上传方法。
// fu.upload();
// 下载方法1
// fu.download();
// 下载方法2。
fu.download("/ftp_test/test.txt","D:\\test2\\test.txt");
fu.closeConnect();
}
}
// 附件中是: WinFtp Server2.0.1特别汉化版
java ftp 上传下载,代码。
原创
©著作权归作者所有:来自51CTO博客作者mb64a401e9f23d4的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:投资学
下一篇:如何获得客户端的IP及MAC地址
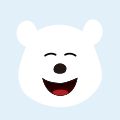
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
SpringBoot项目整合MinIO实现文件的上传下载
SpringBoot项目整合MinIO实现文件的上传下载
spring java 文件路径