class SurfaceHolderCallback implements SurfaceHolder.Callback {
private static final int IMAGE_WIDTH = 512;
private static final int IMAGE_HEIGHT = 384;
private static final String ORIENTATION = "orientation";
private static final String ROTATION = "rotation";
private static final String PORTRAIT = "portrait";
private static final String LANDSCAPE = "landscape";
public void surfaceCreated(SurfaceHolder holder) {
try {
// This case can actually happen if the user opens and closes the camera too frequently.
// The problem is that we cannot really prevent this from happening as the user can easily
// get into a chain of activites and tries to escape using the back button.
// The most sensible solution would be to quit the entire EPostcard flow once the picture is sent.
camera = Camera.open();
} catch(Exception e) {
finish();
return;
}
//Surface.setOrientation(Display.DEFAULT_DISPLAY,Surface.ROTATION_90);
Parameters p = camera.getParameters();
p.setPictureSize(IMAGE_WIDTH, IMAGE_HEIGHT);
camera.getParameters().setRotation(90);
Camera.Size s = p.getSupportedPreviewSizes().get(0);
p.setPreviewSize( s.width, s.height );
p.setPictureFormat(PixelFormat.JPEG);
p.set("flash-mode", "auto");
camera.setParameters(p);
try {
camera.setPreviewDisplay(surfaceHolder);
} catch (Throwable ignored) {
Log.e(APP, "set preview error.", ignored);
}
}
public void surfaceChanged(SurfaceHolder holder, int format, int width,
int height) {
if (isPreviewRunning) {
camera.stopPreview();
}
try {
camera.startPreview();
} catch(Exception e) {
Log.d(APP, "Cannot start preview", e);
}
isPreviewRunning = true;
}
public void surfaceDestroyed(SurfaceHolder arg0) {
if(isPreviewRunning && camera != null) {
if(camera!=null) {
camera.stopPreview();
camera.release();
camera = null;
}
isPreviewRunning = false;
}
}
}
camera 旋转问题
原创mb649166f4c151e 博主文章分类:android ©著作权
©著作权归作者所有:来自51CTO博客作者mb649166f4c151e的原创作品,请联系作者获取转载授权,否则将追究法律责任
下一篇:箭头的使用
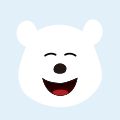
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
有趣的CSS - 旋转的金币
今天分享的是一个旋转的金币,适用于游戏网站,会员币等场景。
css 旋转的金币 动效 ux 用户体验 -
有趣的CSS - 旋转的太极图
用css画一个旋转的太极图。
css html 伪元素 -
有趣的css - 旋转的正方体
用css打造一个好看的透明感的旋转正方晶体。
动效设计 交互设计 css 正方体 -
使用Camera类获取图片被旋转90度问题
问题描述:因需要自动采集图像,故不能调用系统照相界面来获取图像。采用Camera获取图像时,出现了一个问题,图像被旋转了90度。解决方案:1.方式
横屏 解决方案 安卓开发技术 javascript -
camera 捕捉图片后存放问题
在android中camera捕捉相片后 相片的存放是手机程序执行的他会自己捕捉与存取并放到一个
Android 应用程序 ide android -
ios 图片旋转问题
问题 在移动端拍照并上传,在某些ios上会
移动端 js img ios android -
关于phoneGap camera照片放置的位置问题
现在我说下phoneGap camera照片放置的位置问题 在js中有这个一句话 // 使用设备上的摄像头拍照,并获得Base64编
phonegap 照片 function cache file