package com.hupun.crm.test.thread.pattern;
import java.util.concurrent.BlockingQueue;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.LinkedBlockingQueue;
/**
* 阻塞队列BlockingQueue
*
* 下面是用BlockingQueue来实现Producer和Consumer的例子
*/
public class BlockingQueueTest2 {
/**
* 定义装苹果的篮子
*/
public static class Basket {
// 篮子,能够容纳3个苹果
// BlockingQueue<String> basket = new ArrayBlockingQueue<String>(3);
BlockingQueue<String> basket = new LinkedBlockingQueue<String>(3);
// 生产苹果,放入篮子
public void produce() throws InterruptedException {
// put方法放入一个苹果,若basket满了,等到basket有位置
basket.put("An apple");
}
// 消费苹果,从篮子中取走
public String consume() throws InterruptedException {
// get方法取出一个苹果,若basket为空,等到basket有苹果为止
return basket.take();
}
}
// 测试方法
public static void testBasket() {
// 建立一个装苹果的篮子
final Basket basket = new Basket();
// 定义苹果生产者
class Producer implements Runnable {
public String instance = "";
public Producer(String a) {
instance = a;
}
public void run() {
try {
while (true) {
// 生产苹果
System.out.println("生产者准备生产苹果:" + instance);
basket.produce();
System.out.println("! 生产者生产苹果完毕:" + instance);
// 休眠300ms
Thread.sleep(300);
}
} catch (InterruptedException ex) {
}
}
}
// 定义苹果消费者
class Consumer implements Runnable {
public String instance = "";
public Consumer(String a) {
instance = a;
}
public void run() {
try {
while (true) {
// 消费苹果
System.out.println("消费者准备消费苹果:" + instance);
basket.consume();
System.out.println("! 消费者消费苹果完毕:" + instance);
// 休眠1000ms
Thread.sleep(1000);
}
} catch (InterruptedException ex) {
}
}
}
ExecutorService service = Executors.newCachedThreadPool();
Producer producer = new Producer("P1");
Producer producer2 = new Producer("P2");
Producer producer3 = new Producer("P3");
Consumer consumer = new Consumer("C1");
service.submit(producer);
service.submit(producer2);
service.submit(producer3);
service.submit(consumer);
// 程序运行3s后,所有任务停止
try {
Thread.sleep(3000);
} catch (InterruptedException e) {
}
service.shutdownNow();
}
public static void main(String[] args) {
BlockingQueueTest2.testBasket();
}
}
转自:javascript:void(0)
----------------------------------------
简单的队列缓存区实现:
public class Queue <E>{
private List<E> queue = new ArrayList<E>();
private int max;
public Queue() {
super();
max=100;
}
public Queue(int max) {
super();
this.max = max;
}
public synchronized void produce(E e) {
while(true){
if(queue.size()>=max){
try {
wait();
} catch (InterruptedException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
}else{
break;
}
}
queue.add(e);
notifyAll();
}
public synchronized E consume() {
E result = null;
while(true){
if(queue.size()<=0){
try {
wait();
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}else{
result = queue.remove(0);
notifyAll();
break;
}
}
return result;
}
}
====================================
环形缓冲区实现:
public class CircularBuf {
int NMAX = 1000;
int iput = 0; // 环形缓冲区的当前放人位置
int iget = 0; // 缓冲区的当前取出位置
int n = 0; // 环形缓冲区中的元素总数量
Object buffer[];
public CircularBuf() {
super();
buffer = new Object[NMAX];
}
public CircularBuf(int nmax) {
super();
NMAX = nmax;
buffer = new Object[NMAX];
}
/*
* 环形缓冲区的地址编号计算函数,,如果到达唤醒缓冲区的尾部,将绕回到头部。
*
* 环形缓冲区的有效地址编号为:0到(NMAX-1)
*
*/
public int addring(int i) {
return (i + 1) == NMAX ? 0 : i + 1;
}
/* 从环形缓冲区中取一个元素 */
public synchronized Object get() {
int pos;
while (true) {
if (n > 0) {
pos = iget;
iget = addring(iget);
n--;
// System.out.println("get-->" + buffer[pos]);
notifyAll();
return buffer[pos];
} else {
// System.out.println("Buffer is Empty");
try {
wait();
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
}
/* 向环形缓冲区中放人一个元素 */
public synchronized void put(Object z) {
while (true) {
if (n < NMAX) {
buffer[iput] = z;
// System.out.println("put<--" + buffer[iput]);
iput = addring(iput);
n++;
notifyAll();
} else {
// System.out.println("Buffer is full");
try {
wait();
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
}
}
多线程 生产者和消费者模式
原创mb648972af0d702 ©著作权
文章标签 多线程 生产者和消费者模式 System 环形缓冲 java 文章分类 JavaScript 前端开发
下一篇:经常上的网站
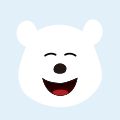
-
chan实现生产者消费者模型
一个简单的例子让你更好的理解golang chan的使用
斐波拉契数列 斐波那契数列 Group -
多线程之生产者消费者模式
多的产品,店员会叫生产者停一下, *如果店中有空位放产品了在通知生产者继续生产,如果店中没有产品了,店员
多线程 生产者 消费者 System ide -
多线程“生产者,消费者”
public class Clerk { /** * 生产者 */ private int pro=0; &n
消费者 private public