1. /************************************************
2. NAME : MMU.C
3. DESC :
4. Revision : 1.0
5. ************************************************/
6.
7. #include "def.h"
8. #include "option.h"
9. #include "2440addr.h"
10. #include "2440lib.h"
11. #include "2440slib.h"
12. #include "mmu.h"
13.
14. // 1) Only the section table is used.
15. // 2) The cachable/non-cachable area can be changed by MMT_DEFAULT value.
16. // The section size is 1MB.
17.
18.
19. extern char __ENTRY[];
20.
21. void MMU_Init(void)
22. {
23. int i,j;
24. /*
25. 这段程序不能实现当前堆栈和代码区域的重新映射,假如你
26. 想让存储区域自由映射,需要你自己编写一个细致入微的MMU初始化代码
27. */
28. //========================== IMPORTANT NOTE =========================
29. //The current stack and code area can't be re-mapped in this routine.
30. //If you want memory map mapped freely, your own sophiscated MMU
31. //initialization code is needed.
32. //===================================================================
33.
34. //禁止数据高速缓存
35. //禁止指令高速缓存
36.
37. //要使用回写操作,一定要对DCache进行清除
38. //If write-back is used,the DCache should be cleared.
39. for(i=0;i<64;i++)
40. for(j=0;j<8;j++)
41. //使整个DCache的数据无效
42. //使无效整个指令Cache
43.
44. if 0
45. //To complete MMU_Init() fast, Icache may be turned on here.
46. //为了快速完成MMU_Init(), Icache在这打开。
47. MMU_EnableICache();
48. endif
49.
50. //禁止MMU
51. //使快表无效
52.
53. //MMU_SetMTT(int vaddrStart,int vaddrEnd,int paddrStart,int attr)
54. //MMU_SetMTT(0x00000000,0x07f00000,0x00000000,RW_CNB); //bank0
55. //bank0
56. //bank0
57. //bank1
58. //bank2
59. //bank3
60. //MMU_SetMTT(0x20000000,0x27f00000,0x20000000,RW_CB); //bank4
61. //bank4 for STRATA Flash
62. //bank5
63. //30f00000->30100000, 31000000->30200000
64. //bank6-1
65. //bank6-2
66. //
67. //bank6-3
68. //bank7
69.
70. //SFR
71. //SFR
72. //SFR
73. //not used
74.
75.
76. //写转换表基地址到C2
77. MMU_SetDomain(0x55555550|DOMAIN1_ATTR|DOMAIN0_ATTR);
78. //DOMAIN1: no_access, DOMAIN0,2~15=client(AP is checked)
79. //写域访问控制位到C3
80. //快速上下文切换,禁止? MVA="VA"
81. //是否开启地址对齐检查功能
82.
83. //使能MMU
84. // 使能ICache
85.
86. //DCache 必须要打开,当MMU打开时.
87. //DCache should be turned on after MMU is turned on.
88. }
89.
90.
91. // attr=RW_CB,RW_CNB,RW_NCNB,RW_FAULT
92. void ChangeRomCacheStatus(int attr)
93. {
94. int i,j;
95. MMU_DisableDCache();
96. MMU_DisableICache();
97. //If write-back is used,the DCache should be cleared.
98. for(i=0;i<64;i++)
99. for(j=0;j<8;j++)
100. MMU_CleanInvalidateDCacheIndex((i<<26)|(j<<5));
101. MMU_InvalidateICache();
102. MMU_DisableMMU();
103. MMU_InvalidateTLB();
104. //bank0
105. //bank1
106. MMU_EnableMMU();
107. MMU_EnableICache();
108. MMU_EnableDCache();
109. }
110.
111.
112. void MMU_SetMTT(int vaddrStart,int vaddrEnd,int paddrStart,int attr)
113. {
114. //定义了页表的指针
115. volatile int i,nSec;
116. //由于内存块是1M的,写页表的基地址
117. // nSec:段大小
118.
119. //页表存储访问信息和存储块的基地址
120. //(((paddrStart>>20)+i)<<20) :对应的物理内存页的地址
121. // attr:访问权限和缓冲属性
122. for(i=0;i<=nSec;i++)*pTT++=attr |(((paddrStart>>20)+i)<<20);
123. }
124.
125. /************************************************
126. NAME : MMU.H
127. DESC :
128. Revision: 1.0
129. ************************************************/
130.
131. #include "2440slib.h"
132.
133. #ifndef __MMU_H__
134. #define __MMU_H__
135. #ifdef __cplusplus
136. extern "C" {
137. #endif
138.
139. #define DESC_SEC (0x2|(1<<4))
140. #define CB (3<<2) //cache_on, write_back
141. #define CNB (2<<2) //cache_on, write_through
142. #define NCB (1<<2) //cache_off,WR_BUF on
143. #define NCNB (0<<2) //cache_off,WR_BUF off
144. #define AP_RW (3<<10) //supervisor=RW, user=RW
145. #define AP_RO (2<<10) //supervisor=RW, user=RO
146.
147. #define DOMAIN_FAULT (0x0)
148. #define DOMAIN_CHK (0x1)
149. #define DOMAIN_NOTCHK (0x3)
150. #define DOMAIN0 (0x0<<5)
151. #define DOMAIN1 (0x1<<5)
152.
153. #define DOMAIN0_ATTR (DOMAIN_CHK<<0)
154. #define DOMAIN1_ATTR (DOMAIN_FAULT<<2)
155.
156. #define RW_CB (AP_RW|DOMAIN0|CB|DESC_SEC)
157. #define RW_CNB (AP_RW|DOMAIN0|CNB|DESC_SEC)
158. #define RW_NCNB (AP_RW|DOMAIN0|NCNB|DESC_SEC)
159. #define RW_FAULT (AP_RW|DOMAIN1|NCNB|DESC_SEC)
160.
161. void MMU_Init(void);
162. void MMU_SetMTT(int vaddrStart,int vaddrEnd,int paddrStart,int attr);
163. void ChangeRomCacheStatus(int attr);
164.
165. #ifdef __cplusplus
166. }
167. #endif
168.
169. #endif /*__MMU_H__*/
170.
171. //具体.h参数请翻看数据手册,及《ARM体系结构与编程+杜春雷》一书
172.
S3C2440裸机驱动--MMU源码分析
转载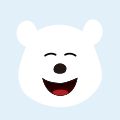
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
s3c2440 lcd驱动移植
一.lcd简介: LCD( Liquid Crystal Display的简称)液晶显示器。 LCD 的构造是在两片平行的成为主流,价格也已
ARM9-Linux-fl2440下的l 3c linux centos -
s3c2440栈分配情况(fl2440裸机 stack)
//2440INIT.S
f5 内存空间 库函数 -
S3C2440 RTC
概述:RTC时钟控制,晶振
S3C2440 RTC时钟 寄存器 实时时钟 晶振 -
S3C2440 gpio
WATCHDOG TIMER 原理图 手册 ...
linux f5 原理图 看门狗 引脚