1.下载ffmpeg
#!/bin/bash
source="ffmpeg-4.1"
if [ ! -r $source ]
then
curl http://ffmpeg.org/releases/${source}.tar.bz2 | tar xj || exit 1
fi
curl 表示下载,后边跟下载的地址。
tar表示解压或者压缩。 x表示解压,j表示是否需要解压bz2压缩包(压缩包格式类型有很多:zip、bz2等等)
2.查看ffmpeg编译配置项
cd 到ffmpeg目录下,
执行./configure --help
ffmpeg有很多配置项:
help options:帮助选项、
standard options:标准选项、
Licensing options:许可选项、
configuration options:配置选项、
external library support:外部库支持、
toolchain options:工具链选项、
advanced options:高级选项、
developer options:开发者选项、
3.编译ffmpeg
下边分析:
1.定义一些字符串
VERSION="4.1" //版本号
SOURCE="ffmpeg-$VERSION" // 需要编译的ffmpeg源文件
CACHEDIR="CACHEDIR" // 保存编译生成的.o文件。
STATICDIR=`pwd`/"weixiao-ffmpeg" // 编译生成的静态库.a的目录
pwd表示获取当前位置的命令。
2.添加ffmpeg的配置项
CONFIGURE_FLAGS="--enable-cross-compile --disable-debug \
--disable-programs --disable-doc --enable-pic"
if [ "$X264" ]
then
CONFIGURE_FLAGS="$CONFIGURE_FLAGS --enable-gpl --enable-libx264"
fi
if [ "$FDK_AAC" ]
then
CONFIGURE_FLAGS="$CONFIGURE_FLAGS --enable-libfdk-aac --enable-nonfree"
fi
FFmpeg一共九个库,常用的(默认)编译的是七个库。 而我们一般用到的是3到4个库,其中最重要的库是用于编解码的库avcodec。
可以根据我们的需要进行编译:
查看这些配置项使用上边第二条讲过的方式,./confifure --help
通过设置CONFIGURE_FLAGS 来配置这些库是否需要,类似下边:
CONFIGURE_FLAGS ="$CONFIGURE_FLAGS --enable-avdevice --enable-avcodec --enable-avformat --disable-postproc"
3.添加需要编译的cpu平台
ARCHS="arm64 armv7 x86_64 i386"
// 也可以动态的通过入参指定需要的cpu平台
if [ "$*" ]
then
#存入输入参数,也就说:外部指定需要编译CPU架构类型
ARCHS ="$*"
fi
4.安装汇编器yasm
if [ ! `which yasm` ]
then
echo "Yasm not found"
if [ ! `which brew` ]
then
echo "Homebrew not found"
ruby -e "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/master/install)" || exit 1
fi
brew install yasm || exit 1
fi
ffmpeg编译用到了汇编,所以需要汇编器yasm,注意不要写错。
5.gas-preprocessor.pl 脚本文件
if [ ! `which gas-preprocessor.pl` ]
then
echo "gas-preprocessor.pl not found"
(curl -L https://github.com/libav/gas-preprocessor/raw/master/gas-preprocessor.pl -o /usr/local/bin/gas-preprocessor.pl && chmod +x /usr/local/bin/gas-preprocessor.pl) || exit 1
fi
ffmpeg编译需要gas-preprocessor.pl 文件,它是一个perl的脚本。
6.根据需要的cpu平台循环编译
CWD=`pwd`
for ARCH in $ARCHS
do
编译代码
done
7.for循环中的代码一,首先设置platform平台(7和下边都是for遵循中执行的代码)
echo "build $ARCH"
mkdir -p "$CACHEDIR/$ARCH"
cd "$CACHEDIR/$ARCH"
CFLAGS="-arch $ARCH"
if [ "$ARCH" = "i386" -o "$ARCH" = "x86_64" ]
then
PLATFORM="iPhoneSimulator"
CFLAGS="$CFLAGS -mios-simulator-version-min=$TARGET"
else
PLATFORM="iPhoneOS"
CFLAGS="$CFLAGS -mios-version-min=$TARGET -fembed-bitcode"
if [ "$ARCH" = "arm64" ]
then
EXPORT="GASPP_FIX_XCODE5=1"
fi
fi
根据需要编译的cpu类型,设置 PLATFORM 是真机还是模拟器。
设置了编译参数 CFLAGS,支持的最低iOS版本。
是否支持x264 和 acc
LDFLAGS="$CFLAGS"
if [ "$X264" ]
then
CFLAGS="$CFLAGS -I$X264/include"
LDFLAGS="$LDFLAGS -L$X264/lib"
fi
if [ "$FDK_AAC" ]
then
CFLAGS="$CFLAGS -I$FDK_AAC/include"
LDFLAGS="$LDFLAGS -L$FDK_AAC/lib"
fi
根据是否支持x264 和acc 继续拼接编译参数。
设置汇编器
XCRUN_SDK=`echo $PLATFORM | tr '[:upper:]' '[:lower:]'`
CC="xcrun -sdk $XCRUN_SDK clang"
if [ "$ARCH" = "arm64" ]
then
AS="gas-preprocessor.pl -arch aarch64 -- $CC"
else
AS="gas-preprocessor.pl -- $CC"
fi
设置了汇编参数 cc 和as
10.设置参数,并编译
TMPDIR=${TMPDIR/%\/} $CWD/$SOURCE/configure \
--target-os=darwin \
--arch=$ARCH \
--cc="$CC" \
--as="$AS" \
$CONFIGURE_FLAGS \
--extra-cflags="$CFLAGS" \
--extra-ldflags="$LDFLAGS" \
--prefix="$STATICDIR/$ARCH" \
|| exit 1
make -j3 install $EXPORT || exit 1
cd $CWD
--prefix表示.a文件的输出文件目录
darwin 是Mac系统的意思
4.编译ffmpeg遇到的问题汇总:
1.shell脚本的语法错误,`` 写成了 '' ,斜着的两个点表示shell命令,竖直的两点表示字符串的意思。
`pwd` 表示获取当前位置的命令, 'pwd'表示pwd字符串
2.shell脚本错误,=号和if判断
name="jack" =号前不能有空格
if [ age == 18 ] []前后必须有空格
3.变量名和专有名词写错
ffmpeg 需要的汇编器是 yasm 而不是ysam。 因为变量名写的时候不报错,所以很难检查出来,要细心些。
4.编译错误,我编译的时候遇到了如下错误:
xcrun -sdk iphoneos clang is unable to create an executable file.
C compiler test failed.
解决方案是命令行执行如下语句:
sudo xcode-select --switch /Applications/Xcode.app
该问题的链接为:issues119 大意是说因为找不到Xcode的目录。
5.完整脚本如下:
VERSION="4.1"
SOURCE="ffmpeg-$VERSION"
CACHEDIR="CACHEDIR"
STATICDIR=`pwd`/"weixiao-ffmpeg"
CONFIGURE_FLAGS="--enable-cross-compile --disable-debug --disable-programs --disable-doc --enable-pic"
if [ "$X264" ]
then
CONFIGURE_FLAGS="$CONFIGURE_FLAGS --enable-gpl --enable-libx264"
fi
if [ "$FDK_AAC" ]
then
CONFIGURE_FLAGS="$CONFIGURE_FLAGS --enable-libfdk-aac --enable-nonfree"
fi
ARCHS="arm64 armv7 x86_64 i386"
TARGET="10.0"
if [ ! `which yasm` ]
then
echo "Yasm not found"
if [ ! `which brew` ]
then
echo "Homebrew not found"
ruby -e "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/master/install)" || exit 1
fi
brew install yasm || exit 1
fi
if [ ! `which gas-preprocessor.pl` ]
then
echo "gas-preprocessor.pl not found"
(curl -L https://github.com/libav/gas-preprocessor/raw/master/gas-preprocessor.pl -o /usr/local/bin/gas-preprocessor.pl && chmod +x /usr/local/bin/gas-preprocessor.pl) || exit 1
fi
CWD=`pwd`
for ARCH in $ARCHS
do
echo "build $ARCH"
mkdir -p "$CACHEDIR/$ARCH"
cd "$CACHEDIR/$ARCH"
CFLAGS="-arch $ARCH"
if [ "$ARCH" = "i386" -o "$ARCH" = "x86_64" ]
then
PLATFORM="iPhoneSimulator"
CFLAGS="$CFLAGS -mios-simulator-version-min=$TARGET"
else
PLATFORM="iPhoneOS"
CFLAGS="$CFLAGS -mios-version-min=$TARGET -fembed-bitcode"
if [ "$ARCH" = "arm64" ]
then
EXPORT="GASPP_FIX_XCODE5=1"
fi
fi
LDFLAGS="$CFLAGS"
if [ "$X264" ]
then
CFLAGS="$CFLAGS -I$X264/include"
LDFLAGS="$LDFLAGS -L$X264/lib"
fi
if [ "$FDK_AAC" ]
then
CFLAGS="$CFLAGS -I$FDK_AAC/include"
LDFLAGS="$LDFLAGS -L$FDK_AAC/lib"
fi
XCRUN_SDK=`echo $PLATFORM | tr '[:upper:]' '[:lower:]'`
CC="xcrun -sdk $XCRUN_SDK clang"
if [ "$ARCH" = "arm64" ]
then
AS="gas-preprocessor.pl -arch aarch64 -- $CC"
else
AS="gas-preprocessor.pl -- $CC"
fi
TMPDIR=${TMPDIR/%\/} $CWD/$SOURCE/configure \
--target-os=darwin \
--arch=$ARCH \
--cc="$CC" \
--as="$AS" \
$CONFIGURE_FLAGS \
--extra-cflags="$CFLAGS" \
--extra-ldflags="$LDFLAGS" \
--prefix="$STATICDIR/$ARCH" \
|| exit 1
make -j3 install $EXPORT || exit 1
cd $CWD
done
FFmpeg android 动态库 ffmpeg库编译
转载文章标签 FFmpeg android 动态库 ffmpeg库编译加文字 ios github 字符串 文章分类 Android 移动开发
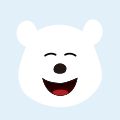