string
strHostName = Dns.GetHostName();
//得到本机的主机名
IPHostEntry ipEntry = Dns.GetHostByName(strHostName);
//取得本机IP
string
strAddr = ipEntry.AddressList[0].ToString();
//假设本地主机为单网卡
在这段代码中使用了两个类,一个是Dns类,另一个为IPHostEntry类,二者都存在于命名空间System.Net中.
Dns类主要是从域名系统(DNS)中检索关于特定主机的信息,上面的代码第一行就从本地的DNS中检索出本地主机名.
IPHostEntry类则将一个域名系统或主机名与一组IP地址相关联,它与DNS类一起使用,用于获取主机的IP地址组.
要获取远程主机的IP地址,其方法也是大同小异.
在获取了IP地址后,如果还需要取得网卡的MAC地址,就需要进一步探究了.
这里又分两种情况,一是本机MAC地址,二是远程主机MAC地址.二者的获取是完全不同的.
在获取本机的MAC地址时,可以使用WMI规范,通过SELECT语句提取MAC地址.在.NET框架中,WMI规范的实现定义在System.Management命名空间中.
ManagementObjectSearcher类用于根据指定的查询检索管理对象的集合
ManagementObjectCollection类为管理对象的集合,下例中由检索对象返回管理对象集合赋值给它.
示例:
ManagementObjectSearcher query =
new
ManagementObjectSearcher(
"SELECT * FROM Win32_NetworkAdapterConfiguration"
) ;
ManagementObjectCollection queryCollection = query.Get();
foreach
( ManagementObject mo
in
queryCollection )
{
if
(mo[
"IPEnabled"
].ToString() ==
"True"
)
mac = mo[
"MacAddress"
].ToString();
}
获取远程主机的MAC地址时,需要借用API函数SendARP.该函数使用ARP协议,向目的主机发送ARP包,利用返回并存储在高速缓存中的IP和MAC地址对,从而获取远程主机的MAC地址.
示例:
Int32 ldest= inet_addr(remoteIP);
//目的ip
Int32 lhost= inet_addr(localIP);
//本地ip
try
{
Int64 macinfo =
new
Int64();
Int32 len = 6;
int
res = SendARP(ldest,0,
ref
macinfo,
ref
len);
//发送ARP包
return
Convert.ToString(macinfo,16);
}
catch
(Exception err)
{
Console.WriteLine(
"Error:{0}"
,err.Message);
}
return
0.ToString();
但使用该方式获取MAC时有一个很大的限制,就是只能获取同网段的远程主机MAC地址.因为在标准网络协议下,ARP包是不能跨网段传输的,故想通过ARP协议是无法查询跨网段设备MAC地址的。
示例程序:
using
System.Net;
using
System;
using
System.Management;
using
System.Runtime.InteropServices;
public
class
getIP
{
[DllImport(
"Iphlpapi.dll"
)]
private
static
extern
int
SendARP(Int32 dest,Int32 host,
ref
Int64 mac,
ref
Int32 length);
[DllImport(
"Ws2_32.dll"
)]
private
static
extern
Int32 inet_addr(
string
ip);
//获取本机的IP
public
string
getLocalIP()
{
string
strHostName = Dns.GetHostName();
//得到本机的主机名
IPHostEntry ipEntry = Dns.GetHostByName(strHostName);
//取得本机IP
string
strAddr = ipEntry.AddressList[0].ToString();
return
(strAddr);
}
//获取本机的MAC
public
string
getLocalMac()
{
string
mac =
null
;
ManagementObjectSearcher query =
new
ManagementObjectSearcher(
"SELECT * FROM Win32_NetworkAdapterConfiguration"
) ;
ManagementObjectCollection queryCollection = query.Get();
foreach
( ManagementObject mo
in
queryCollection )
{
if
(mo[
"IPEnabled"
].ToString() ==
"True"
)
mac = mo[
"MacAddress"
].ToString();
}
return
(mac);
}
//获取远程主机IP
public
string
[] getRemoteIP(
string
RemoteHostName)
{
IPHostEntry ipEntry = Dns.GetHostByName(RemoteHostName);
IPAddress[] IpAddr = ipEntry.AddressList;
string
[] strAddr =
new
string
[IpAddr.Length];
for
(
int
i=0;i<IPADDR.LENGTH;I++)
{
strAddr[i] = IpAddr[i].ToString();
}
return
(strAddr);
}
//获取远程主机MAC
public
string
getRemoteMac(
string
localIP,
string
remoteIP)
{
Int32 ldest= inet_addr(remoteIP);
//目的ip
Int32 lhost= inet_addr(localIP);
//本地ip
try
{
Int64 macinfo =
new
Int64();
Int32 len = 6;
int
res = SendARP(ldest,0,
ref
macinfo,
ref
len);
return
Convert.ToString(macinfo,16);
}
catch
(Exception err)
{
Console.WriteLine(
"Error:{0}"
,err.Message);
}
return
0.ToString();
}
public
static
void
Main(
string
[] args)
{
getIP gi =
new
getIP();
Console.WriteLine(
"本地网卡信息:"
);
Console.WriteLine(gi.getLocalIP() +
" - "
+ gi.getLocalMac());
Console.WriteLine(
"\n\r远程网卡信息:"
);
string
[] temp = gi.getRemoteIP(
"scmobile-tj2"
);
for
(
int
i=0;i<TEMP.LENGTH;I++)
{
Console.WriteLine(temp[i]);
}
Console.WriteLine(gi.getRemoteMac(
"192.168.0.3"
,
"192.168.0.1"
));
}
}
能根据MAC地址分配不通VLAN的IP吗 根据mac地址获取ip地址
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
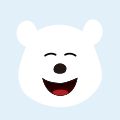
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
获取IP地址
端点(API接口)访问获取IP地址!
IP Java SpringBoot\ -
java根据本地Ip获取mac地址
下面这个方法是获取客户端请求地址
javaWeb学习笔记 .net java i++ 数组长度