package com.cn.file;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStreamWriter;
import java.io.PrintStream;
import java.io.PrintWriter;
import java.io.Reader;
import java.io.UnsupportedEncodingException;
import java.io.Writer;/**
* 文件及文件夹操作工具类
* 文件中\r\n为换行
* @author Administrator
*
*/
public class FileUtils {
/**
* FileOutputStream 文件写入内容
* @param filePath 文件路径
* @param content 文件内容
*/
public void fileOutput_FileOutputStream(String filePath,String content){
try {
File file=new File(filePath); //创建file
if(!file.exists()){ //判断文件是否存在
file.createNewFile(); //创建文件
}
//创建文件输出流,参数true为追加内容,若无则是覆盖内容
FileOutputStream out = new FileOutputStream(file,true);
byte[] contentInBytes = content.getBytes("utf-8"); //内容转化
out.write(contentInBytes); //写入内容
//关闭流
out.flush();
out.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
/**
* FileWriter 文件写入内容
* @param filePath 文件路径
* @param content 文件内容
*/
public void fileOutput_FileWriter(String filePath,String content){
try {
File file=new File(filePath); //创建file
if(!file.exists()){ //判断文件是否存在
file.createNewFile(); //创建文件
}
//参数true为追加内容,若无则是覆盖内容
FileWriter fw = new FileWriter(file,true);
fw.write(content);
//关闭流
fw.flush();
fw.close();
} catch (IOException e) {
e.printStackTrace();
}
}
/**
* BufferedWriter 文件写入内容
* @param filePath 文件路径
* @param content 文件内容
*/
public void fileOutput_BufferedWriter(String filePath,String content){
try {
File file=new File(filePath); //创建file
if(!file.exists()){ //判断文件是否存在
file.createNewFile(); //创建文件
}
//参数true为追加内容,若无则是覆盖内容
BufferedWriter bw = new BufferedWriter(new FileWriter(file,true));
bw.write(content);
//关闭流
bw.flush();
bw.close();
} catch (IOException e) {
e.printStackTrace();
}
}
/**
* PrintWriter 文件写入内容
* @param filePath 文件路径
* @param content 文件内容
*/
public void fileOutput_PrintWriter(String filePath,String content){
try {
File file=new File(filePath); //创建file
if(!file.exists()){ //判断文件是否存在
file.createNewFile(); //创建文件
}
//参数true为追加内容,若无则是覆盖内容
PrintWriter pw = new PrintWriter(new FileWriter(file,true));
pw.println(content); // 换行
//pw.print(content); // 不换行
//关闭流
pw.flush();
pw.close();
} catch (IOException e) {
e.printStackTrace();
}
}
/**
* PrintStream 文件写入内容
* @param filePath 文件路径
* @param content 文件内容
*/
public void fileOutput_PrintStream(String filePath,String content){
try {
File file=new File(filePath); //创建file
if(!file.exists()){ //判断文件是否存在
file.createNewFile(); //创建文件
}
//参数true为追加内容,若无则是覆盖内容
PrintStream pw=new PrintStream(new FileOutputStream(file,true));
pw.println(content);
//关闭流
pw.flush();
pw.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
// System.out.write()方法是字符流,System.out.println()方法是字节流
/**
* FileInputStream 读取文件内容并写入指定路径文件中
* 以字节为单位读取文件,常用于读二进制文件,如图片、声音、影像等文件
* @param filePath 文件路径
* @param newFilePath 要写入的路径
*/
public void fileInput_FileInputStream(String filePath,String newFilePath){
try {
File file=new File(filePath); //创建file
if(!file.exists() || file.isDirectory()){ //判断文件是否存在或者是目录
throw new FileNotFoundException(); //抛出异常
}
InputStream in = new FileInputStream(file); // 读取的文件
FileOutputStream out = new FileOutputStream(newFilePath,true); // 指定要写入的文件 /*// 一次读一个字节
int tempbyte;
while ((tempbyte = in.read()) != -1) {
out.write(tempbyte);
//System.out.write(tempbyte);
} */
// 一次读多个字节
int byteread = 0;
byte[] tempbytes = new byte[1024];
while ((byteread = in.read(tempbytes)) != -1) {
out.write(tempbytes, 0, byteread);
//System.out.write(tempbytes, 0, byteread);
}
//关闭流
out.flush();
out.close();
in.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} }
/**
* InputStreamReader 读取文件内容并写入指定路径文件中
* 以字符为单位读取文件,常用于读文本,数字等类型的文件
* @param filePath 文件路径
* @param newFilePath 要写入的路径
*/
public void fileInput_InputStreamReader(String filePath,String newFilePath){
try {
File file=new File(filePath); //创建file
if(!file.exists() || file.isDirectory()){ //判断文件是否存在或者是目录
throw new FileNotFoundException(); //抛出异常
}
Reader reader = new InputStreamReader(new FileInputStream(file));
// 指定要写入的文件
Writer writer = new OutputStreamWriter(new FileOutputStream(newFilePath,true));
// 一次读一个字节
int tempchar;
while ((tempchar = reader.read()) != -1) {
//System.out.print(tempchar);
writer.write(tempchar);
}
/*// 一次读多个字节
char[] tempchars = new char[1024];
int charread = 0;
// 读入多个字符到字符数组中,charread为一次读取字符数
while ((charread = reader.read(tempchars)) != -1) {
//System.out.print(tempchar);
writer.write(tempchars);
}*/
//关闭流
writer.flush();
writer.close();
reader.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
/**
* BufferedReader 读取文件内容并写入指定路径文件中
* 以行为单位读取文件,常用于读面向行的格式化文件
* @param filePath 文件路径
* @param newFilePath 要写入的路径
*/
public void fileInput_BufferedReader(String filePath,String newFilePath){
try {
File file=new File(filePath); //创建file
if(!file.exists() || file.isDirectory()){ //判断文件是否存在或者是目录
throw new FileNotFoundException(); //抛出异常
}
BufferedReader reader = new BufferedReader(new FileReader(file));
BufferedWriter writer = new BufferedWriter(new FileWriter(newFilePath,true));
String tempString = null;
int line = 1;
// 一次读入一行,直到读入null为文件结束
while ((tempString = reader.readLine()) != null) {
//System.out.println(tempString);
writer.write(tempString);
writer.newLine();
}
//关闭流
writer.flush();
writer.close();
reader.close();
} catch (Exception e) {
e.printStackTrace();
}
}
/**
* 文件复制
* @param targetFile 目标文件
* @param newFile 新文件
* @throws IOException 文件流异常
*/
public void copyFile(String targetFile,String newFile) throws IOException{
FileInputStream in=new FileInputStream(targetFile); //创建输入流(目标文件)
File file=new File(newFile); //新文件
if(!file.exists()){ //判断新文件是否存在
file.createNewFile(); //创建新文件
}
FileOutputStream out=new FileOutputStream(file); //创建输出流
int c; //标识符
byte buffer[]=new byte[1024]; //创建byte数组
while((c=in.read(buffer))!=-1){ //循环读取输入流
for(int i=0;i<c;i++) //读取到的内容写入输出流
out.write(buffer[i]);
}
out.close(); //关闭输入流
in.close(); //关闭输出流
}
/**
* 文件重命名
* @param path 路径
* @param oldname 原文件名
* @param newname 新文件名
*/
public void renameFile(String path,String oldName,String newName){
if(!oldName.equals(newName)){ //新的文件名和以前文件名不同时,才有必要进行重命名
File oldfile=new File(path+"/"+oldName);//原文件
File newfile=new File(path+"/"+newName);//新文件
if(newfile.exists()) //若在该目录下已经有一个文件和新文件名相同,则不允许重命名
System.out.println(newName+"已经存在!");
else{
oldfile.renameTo(newfile); //文件重命名
}
}
} /**
* 文件移动
* @param filename 文件名
* @param oldpath 原路径
* @param newpath 新路径
* @param cover 是否覆盖重名文件
*/
public void changeDirectory(String filename,String oldpath,String newpath,boolean cover){
if(!oldpath.equals(newpath)){ //新的路径和以前路径不同时,才有必要进行移动
File oldfile=new File(oldpath+"/"+filename);//原文件
File newfile=new File(newpath+"/"+filename);//新文件
if(newfile.exists()){ //若在待转移目录下,已经存在待转移文件
if(cover){ //覆盖
oldfile.renameTo(newfile); //移动
}else{
System.out.println("在新目录下已经存在:"+filename);
}
}
else{ //不存在同名文件
oldfile.renameTo(newfile); //移动
}
}
}
/**
* 创建文件
* @param path 路径
* @param fileName 文件名
* @throws IOException 文件流异常
*/
public void createFile(String path,String fileName) throws IOException{
File file=new File(path+"/"+fileName);
if(!file.exists())
file.createNewFile();
}
/**
* 创建文件夹
* @param path 路径
*/
public void createDir(String path){
File dir=new File(path);
if(!dir.exists())
dir.mkdir();
}
/**
* 删除文件
* @param path 路径
* @param fileName 文件名
*/
public void delFile(String path,String fileName){
File file=new File(path+"/"+fileName);
if(file.exists()&&file.isFile())
file.delete();
}
/**
* 删除文件夹
* @param path 路径
*/
public void delDir(String path){
File dir=new File(path);
if(dir.exists()){
File[] tmp=dir.listFiles();
for(int i=0;i<tmp.length;i++){
if(tmp[i].isDirectory()){
delDir(path+"/"+tmp[i].getName());
}
else{
tmp[i].delete();
}
}
dir.delete();
}
}
}
java中 file 显示关闭file java 关闭文件流
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
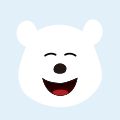
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章