一、MD5帮助类
/**
* 功能简介:MD5加密工具类
* 密码等安全信息存入数据库时,转换成MD5加密形式
* @author
*/
public class MD5Util {
public static String getMd5(String inStr) {
String outStr = null;
if (inStr == null) {
outStr = null;
} else if ("".equals(inStr)) {
outStr = "";
} else {
try {
MessageDigest md = MessageDigest.getInstance("MD5");
md.update(inStr.getBytes());
byte b[] = md.digest();
StringBuffer buf = new StringBuffer();
for (int i = 1; i < b.length; i++) {
int c = b[i] >>> 4 & 0xf;
buf.append(Integer.toHexString(c));
c = b[i] & 0xf;
buf.append(Integer.toHexString(c));
}
outStr = buf.toString();
} catch (NoSuchAlgorithmException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
return outStr;
}
public static void main(String[] args) {
String ss = MD5Util.getMd5("111");
System.err.println(ss);
}
}
二、AES加解密工具类
public class AESUtil {
private static final String ENCODE_RULES = "ml";
/**
* 加密
* 1.构造密钥生成器
* 2.根据ecnodeRules规则初始化密钥生成器
* 3.产生密钥
* 4.创建和初始化密码器
* 5.内容加密
* 6.返回字符串
*/
public static String aesEncode(String content) {
try {
//1.构造密钥生成器,指定为AES算法,不区分大小写
KeyGenerator keyGenerator = KeyGenerator.getInstance("AES");
//2.根据ecnodeRules规则初始化密钥生成器
//生成一个128位的随机源,根据传入的字节数组
SecureRandom random = SecureRandom.getInstance("SHA1PRNG");
random.setSeed(ENCODE_RULES.getBytes());
keyGenerator.init(128, random);
//3.产生原始对称密钥
SecretKey originalKey = keyGenerator.generateKey();
//4.获得原始对称密钥的字节数组
byte[] raw = originalKey.getEncoded();
//5.根据字节数组生成AES密钥
SecretKey key = new SecretKeySpec(raw, "AES");
//6.根据指定算法AES自成密码器
Cipher cipher = Cipher.getInstance("AES");
//7.初始化密码器,第一个参数为加密(Encrypt_mode)或者解密解密(Decrypt_mode)操作,第二个参数为使用的KEY
cipher.init(Cipher.ENCRYPT_MODE, key);
//8.获取加密内容的字节数组(这里要设置为utf-8)不然内容中如果有中文和英文混合中文就会解密为乱码
byte[] byteEncode = content.getBytes("utf-8");
//9.根据密码器的初始化方式--加密:将数据加密
byte[] byteAES = cipher.doFinal(byteEncode);
//10.将加密后的数据转换为字符串
//这里用Base64Encoder中会找不到包
//解决办法:
//在项目的Build path中先移除JRE System Library,再添加库JRE System Library,重新编译后就一切正常了。
String aesEncode = new String(new BASE64Encoder().encode(byteAES));
//11.将字符串返回
return aesEncode;
} catch (NoSuchAlgorithmException e) {
e.printStackTrace();
} catch (NoSuchPaddingException e) {
e.printStackTrace();
} catch (InvalidKeyException e) {
e.printStackTrace();
} catch (IllegalBlockSizeException e) {
e.printStackTrace();
} catch (BadPaddingException e) {
e.printStackTrace();
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
//如果有错就返加nulll
return null;
}
/**
* 解密
* 解密过程:
* 1.同加密1-4步
* 2.将加密后的字符串反纺成byte[]数组
* 3.将加密内容解密
*/
public static String aesDecode(String content) {
try {
//1.构造密钥生成器,指定为AES算法,不区分大小写
KeyGenerator keygen = KeyGenerator.getInstance("AES");
//2.根据ecnodeRules规则初始化密钥生成器
//生成一个128位的随机源,根据传入的字节数组
SecureRandom random = SecureRandom.getInstance("SHA1PRNG");
random.setSeed(ENCODE_RULES.getBytes());
keygen.init(128, random);
//3.产生原始对称密钥
SecretKey originalKey = keygen.generateKey();
//4.获得原始对称密钥的字节数组
byte[] raw = originalKey.getEncoded();
//5.根据字节数组生成AES密钥
SecretKey key = new SecretKeySpec(raw, "AES");
//6.根据指定算法AES自成密码器
Cipher cipher = Cipher.getInstance("AES");
//7.初始化密码器,第一个参数为加密(Encrypt_mode)或者解密(Decrypt_mode)操作,第二个参数为使用的KEY
cipher.init(Cipher.DECRYPT_MODE, key);
//8.将加密并编码后的内容解码成字节数组
byte[] byteContent = new BASE64Decoder().decodeBuffer(content);
/*
* 解密
*/
byte[] byteDecode = cipher.doFinal(byteContent);
String aesDecode = new String(byteDecode, "utf-8");
return aesDecode;
} catch (NoSuchAlgorithmException e) {
e.printStackTrace();
} catch (NoSuchPaddingException e) {
e.printStackTrace();
} catch (InvalidKeyException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} catch (IllegalBlockSizeException e) {
throw new RuntimeException("兄弟,配置文件中的密码需要使用AES加密,请使用com.zheng.common.util.AESUtil工具类修改这些值!");
//e.printStackTrace();
} catch (BadPaddingException e) {
e.printStackTrace();
}
//如果有错就返加nulll
return null;
}
public static void main(String[] args) {
String[] keys = {
"", "123456"
};
System.out.println("key | AESEncode | AESDecode");
for (String key : keys) {
System.out.print(key + " | ");
String encryptString = aesEncode(key);
System.out.print(encryptString + " | ");
String decryptString = aesDecode(encryptString);
System.out.println(decryptString);
}
String aa="你好";
String jimi = aesEncode(aa);
String jiemmi=aesDecode(jimi);
System.out.println("加密:"+jimi);
System.out.println("解密:"+jiemmi);
}
}
三、RSA加密解密类
public class RSASecurityCoder {
// 非对称加密密钥算法
private static final String Algorithm = "RSA";
// 密钥长度,用来初始化
private static final int Key_Size = 1024;
// 公钥
private final byte[] publicKey;
// 私钥
private final byte[] privateKey;
/**
* 构造函数,在其中生成公钥和私钥
*
* @throws Exception
*/
public RSASecurityCoder() throws Exception {
// 得到密钥对生成器
KeyPairGenerator kpg = KeyPairGenerator.getInstance(Algorithm);
kpg.initialize(Key_Size);
// 得到密钥对
KeyPair kp = kpg.generateKeyPair();
// 得到公钥
RSAPublicKey keyPublic = (RSAPublicKey) kp.getPublic();
publicKey = keyPublic.getEncoded();
// 得到私钥
RSAPrivateKey keyPrivate = (RSAPrivateKey) kp.getPrivate();
privateKey = keyPrivate.getEncoded();
}
/**
* 用公钥对字符串进行加密
*
* 说明:
*
* @param originalString
* @param publicKeyArray
* @return
* @throws Exception
* 创建时间:2010-12-1 下午06:29:51
*/
public byte[] getEncryptArray(String originalString, byte[] publicKeyArray) throws Exception {
// 得到公钥
X509EncodedKeySpec keySpec = new X509EncodedKeySpec(publicKeyArray);
KeyFactory kf = KeyFactory.getInstance(Algorithm);
PublicKey keyPublic = kf.generatePublic(keySpec);
// 加密数据
Cipher cp = Cipher.getInstance(Algorithm);
cp.init(Cipher.ENCRYPT_MODE, keyPublic);
return cp.doFinal(originalString.getBytes());
}
/**
* 使用私钥进行解密
*
* 说明:
*
* @param encryptedDataArray
* @return
* @throws Exception
* 创建时间:2010-12-1 下午06:35:28
*/
public String getDecryptString(byte[] encryptedDataArray) throws Exception {
// 得到私钥
PKCS8EncodedKeySpec keySpec = new PKCS8EncodedKeySpec(privateKey);
KeyFactory kf = KeyFactory.getInstance(Algorithm);
PrivateKey keyPrivate = kf.generatePrivate(keySpec);
// 解密数据
Cipher cp = Cipher.getInstance(Algorithm);
cp.init(Cipher.DECRYPT_MODE, keyPrivate);
byte[] arr = cp.doFinal(encryptedDataArray);
// 得到解密后的字符串
return new String(arr);
}
public byte[] getPublicKey() {
return publicKey;
}
public static void main(String[] arr) throws Exception {
String str = "你好,世界! Hello,world!";
System.out.println("准备用公钥加密的字符串为:" + str);
// 用公钥加密
RSASecurityCoder rsaCoder = new RSASecurityCoder();
byte[] publicKey = rsaCoder.getPublicKey();
//私钥
byte[] encryptArray = rsaCoder.getEncryptArray(str, publicKey);
System.out.print("用公钥加密后的结果为:");
StringBuffer sBuffer=new StringBuffer();
for (byte b : encryptArray) {
sBuffer.append(b);
// System.out.print(b);
}
System.out.println(sBuffer.toString());
// 用私钥解密
String str1 = rsaCoder.getDecryptString(encryptArray);
System.out.println("用私钥解密后的字符串为:" + str1);
}
}
JAVA设计一个加密器和解密器 java密码加密解密工具类
转载文章标签 JAVA设计一个加密器和解密器 java 加密解密类 System 字符串 初始化 文章分类 Java 后端开发
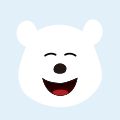