1.Java的基本数据类型
Java的基本数据类型有8种
整数类型:
byte 字节型 8bit -128~127 (负2的8次方到2的8次方-1)
short 短整型 16bit
int 整型 32bit
long 长整型 64bit
浮点型:
float 单精度 32bit
double 双精度 64bit
布尔
boolean 布尔类型 true和false
字符
char 字符型 16bit
2.String类的使用
1.String在Java中是类。
String 类包括的方法可用于检查序列的单个字符、比较字
符串、搜索字符串、提取子字符串、创建字符串副本并将
所有字符全部转换为大写或小写。
2.String表示的是字符串
Java 程序中的所有字符串字面值(如 "abc" )都作为此类的实例实现。
字符串是常量;它们的值在创建之后不能更改。字符串缓冲区支持可变的
字符串。因为 String 对象是不可变的,所以可以共享。例如:
String str = "abc";
等效于:
char data[] = {'a', 'b', 'c'};
String str = new String(data);
使用字符串的基本方法,eg:
System.out.println("abc");
String cde = "cde";
System.out.println("abc" + cde);
String c = "abc".substring(2,3);
String d = cde.substring(1, 2);
[color=blue][size=medium]练习:统计字符串中每一个字符出现的次数[/size][/color]
我的代码:
package ss;
public class string {
/**
* 主函数
*/
public static void main(String[] args) {
//定义一个字符串变量
String str="euriyui3743289^%^&*&DJHK2312";
//第一个循环:返回第i个引索处的char值
for(int i=0;i<str.length();i++){
char a=str.charAt(i);
int index=str.indexOf(a);
[color=red]int n=str.lastIndexOf(a);[/color]//第i个字符所在的最后位置
int k=0;
//第二个循环:在给定字符串中找出与第一个循环中相同的字符
for(int j=index;j<str.length();j++){
char b=str.charAt(j);
[color=red]if(a==b&&b!=' '){
k++;
if(j>=n)[/color]//当且仅当找到最终出现的相同字符才输出个数
System.out.println(a+"字符出现的次数为"+k);
}
}
//第三个循环:用空格替换找出的相同字符
[color=red]for(int x=0;x<str.length();x++){
char c=str.charAt(x);
if(c==a)
str=str.replace(c,' ');}[/color]
}
}
}
解题思路:
在该代码中使用了三个循环,其中第一个和第二个循环构成一组内外循环;用于寻找字符串中相同的字符及计算其个数。第三个循环和第一个并列,用于将计算过的字符转换成空格。
使用到的String类中的方法有charAt()、indexOf()、lastIndexOf()、length()、replace()。
cherAt()在该代码中的作用是将字符串中的字符一个个拆分,易于比较。
indexOf()和lastIndexOf()分别用来找到字符在字符串中出现的最前和最后位置。
length()计算字符串的长度,使循环有一个终点。
replace()是string中字符与字符之间的替换。
练习中遇到的问题及解答方法:
1.首先想到用内外循环来完成对每个字符出现个数的计算。但因为开始时将计算个数的变量初始化k=0放在第一个循环之前,导致从第二个字符开始,每个字符出现次数都是前面字符与该字符总数之和,于是将初始化k=0放在第一个循环之内,这样,每计算一个字符时都会初始化k值。
2.在循环时发现每次第二个循环中找出与第一个循环中相同的字符时就会立即输出个数,当遇到第二个时又会输出,同时个数+1,而且即使后面的字符与前面的相同,也会重复输出。解决前者,用到了lastIndexOf(),直到找到了最后一个才输出。解决后者,用到了replace(),将找过的字符用空格替换,只有当找出的字符不是空格时才输出。但其实这样再用一个循环比较繁琐。
看看其他人的代码:
同组组员代码:
package stringcount;
public class ctest0705 {
/**
* @param args
*/
public static void main(String[] args) {
// TODO Auto-generated method stub
String str = "hdjsakhdjksahkdjhasyrtr";
int n;
//外循环:从字符串第一个开始与后面的比较
for(int i = 0;i < str.length();){
char a = str.charAt(i);
int k = 0;
//内循环:从被比较的开始进行比较,相同k+1
for([color=red]n = 0[/color];n < str.length();n++){
char b = str.charAt(n);
if(a == b)
k++;
}
System.out.println(a+"的个数为"+k);
[color=red]String str1 = str.valueOf(a);
str = str.replace(str1, "");[/color] }
}
}
老师的代码:
package cn.netjava.lesson01;
public class StringPractise {
/**
* @param args
*/
public static void main(String[] args) {
// 实例化一个接受命令行输入信息的对象
java.util.Scanner sc = new java.util.Scanner(System.in);
// 实例化StringPractise类的对象
StringPractise sp = new StringPractise();
System.out.println("请输入要统计的字符串:");
// 获取输入的一行字符串
String temp = sc.nextLine();
System.out.println("开始使用第一种方式统计.");
//调用第一种统计的方式
sp.wayOne(temp);
System.out.println("请输入要统计的字符串:");
// 获取输入的一行字符串
temp = sc.nextLine();
System.out.println("开始使用第二种方式统计.");
// 调用第二种统计的方式
sp.wayTwo(temp);
}
/**
* 第一种统计方式 根据ascii和数组来实现
*/
public void wayOne(String temp) {
// 定义一个存储统计次数的数组
int[] array = new int[256];
// 循环遍历字符串
for (int i = 0; i < temp.length(); i++) {
// 获取指定索引位置的字符
char c = temp.charAt(i);
// 将字符转换为对应的ascii
int ascii = c;
// 将对应的ascii位置的数组元素加1
array[ascii]++;
}
// 输出
for (int i = 0; i < array.length; i++) {
// 如果统计个数部位0则输出
if (array[i] != 0) {
char c = (char) i;
System.out.println("字符" + c + "出现的次数是" + array[i]);
}
}
}
/**
* 第二种统计方式 根据replace方法来实现
*/
public void wayTwo(String temp) {
// 循环遍历字符串
for (int i = 0; i < temp.length();) {
int count = 1;// 计数器
// 循环遍历字符串,从i+1的基础上开始,让i位置的每一个字符都与后边的字符进行比较
for (int j = i + 1; j < temp.length(); j++) {
// 如果相等
if (temp.charAt(i) == temp.charAt(j)) {
// 计数器加1
count++;
}
}
System.out.println("字符" + temp.charAt(i) + "出现的次数是" + count);
// 将相同的字符全部都替换为空
temp = temp.replace(temp.charAt(i) + "", "");
}
}
}
structs 数据类型 java java基本数据类型 string
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
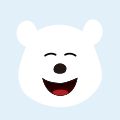
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
JAVA的基本数据类型例子 java 的基本数据类型
基本数据类型也称为简单数据类型,Java语言中有八种基本数据类型,分别为 boolean、byte、short、char、int、long、float、double,这八种基本数据类型通常分为四大类型;逻辑类型(布尔类型):boolean;整数类型 :byte、short、int、long;浮点类型 :float、double;字符类型 :char;以下进行逐一介绍这八种基本数据类型:一、逻辑类型
JAVA的基本数据类型例子 八种基础数据类型 Java基础数据类型的取值范围 java基本数据类型的使用 Java基本数据类型的定义