展开全部
package mainWindow;
import java.awt.*;
import javax.swing.*;
import java.util.*;
import java.io.IOException;
import java.awt.event.*;
import javax.swing.event.*;
/*程序文件:ShutDownWindow.java
*程序功能:程序的定时关机窗体
*更新e68a843231313335323631343130323136353331333239306530时间:2009.10.26
*/
public class ShutDownWindow extends JFrame implements ActionListener,DocumentListener{
/**
*
*/
private static final long serialVersionUID = 2084958924713854452L;
JTextField 时,分,秒;
JLabel 现在时间,设置时,设置分,设置秒;
JButton 关机,重启,注销,清空,取消;
Box 左,中,右,中部; //Box是使用 BoxLayout 对象作为其布局管理器的一个轻量级容器
JPanel 底部;
Container 容器;
int 标志,nh,nm,ns,wh,wm,ws;
ShutDownWindow(String s){
super(s);
setVisible(true);
setResizable(false); //设置窗体是否允许用户调整窗体大小
setSize(300,200);
Dimension 屏幕=Toolkit.getDefaultToolkit().getScreenSize(); //获得屏幕大小
setLocation(屏幕.width-300,0);
现在时间=new JLabel();
现在时间.setForeground(Color.RED);
现在时间.setText(nowTime());
时=new JTextField(4);
时.setForeground(Color.BLUE);
时.setText(null);
分=new JTextField(4);
分.setForeground(Color.BLUE);
分.setText(null);
秒=new JTextField(4);
秒.setForeground(Color.BLUE);
秒.setText(null);
设置时=new JLabel("设定小时");
设置分=new JLabel("设定分钟");
设置秒=new JLabel("设定秒钟");
关机=new JButton("关机");
重启=new JButton("重启");
注销=new JButton("注销");
取消=new JButton("取消");
清空=new JButton("清空");
关机.setEnabled(false);
重启.setEnabled(false);
取消.setEnabled(false);
注销.setEnabled(false);
清空.setEnabled(false);//按钮不可用
中部=Box.createHorizontalBox(); //创建一个从左到右显示其组件的 Box
validate();
容器=getContentPane();
容器.setLayout(new BorderLayout());
左=Box.createVerticalBox(); //创建一个从左到右显示其组件的 Box
中=Box.createVerticalBox();
右=Box.createVerticalBox();
左.add(设置时);
左.add(Box.createVerticalStrut(20)); //创建一个不可见的、固定高度的组件
左.add(设置分);
左.add(Box.createVerticalStrut(20));
左.add(设置秒);
中.add(时);
中.add(Box.createVerticalStrut(9));
中.add(分);
中.add(Box.createVerticalStrut(9));
中.add(秒);
右.add(关机);
右.add(Box.createVerticalStrut(10));
右.add(重启);
右.add(Box.createVerticalStrut(10));
右.add(注销);
validate(); //使用 validate 方法会使容器再次布置其子组件
中部.add(左);
中部.add(Box.createHorizontalStrut(15));
中部.add(中);
中部.add(Box.createHorizontalStrut(15));
中部.add(右);
中部.validate();
底部=new JPanel();
底部.setBackground(Color.GREEN);
底部.add(清空);
底部.add(取消);
底部.validate();
容器.add(现在时间,BorderLayout.NORTH);
容器.add(中部,BorderLayout.CENTER);
容器.add(底部,BorderLayout.SOUTH);
容器.validate();
容器.setBackground(Color.GRAY);
关机.addActionListener(this); //为各按钮标签添加监听对象
重启.addActionListener(this);
注销.addActionListener(this);
取消.addActionListener(this);
清空.addActionListener(this);
时.getDocument().addDocumentListener(this);
分.getDocument().addDocumentListener(this);
秒.getDocument().addDocumentListener(this);
//******************* 匿名线程,实现时间动态显示 *******************//
new Thread(new Runnable() {
public void run()
{
while(true) //为了实时监听所以得用死循环
{
try
{
Thread.sleep(1000); //条件合适后线程休眠1秒钟
}
catch(Exception e)
{
e.printStackTrace(); //输入异常到指定地方
}
nowTime(); //调用nowTime()方法获得当前时间
}
}
}).start(); //调用start()后线程处于就绪状态
}
//******************** 得到当前系统时间并显示 *********************//
public String nowTime(){
Calendar 日历=Calendar.getInstance(); //使用默认时区和语言环境获得一个日历
int ny=日历.get(Calendar.YEAR);
int nmo=日历.get(Calendar.MONTH)+1; //因为月是从0开始算起的,所以得加上1
int nd=日历.get(Calendar.DAY_OF_MONTH);
nh=日历.get(Calendar.HOUR_OF_DAY);
nm=日历.get(Calendar.MINUTE);
ns=日历.get(Calendar.SECOND);
String ss=new String(" 现在是"+ny+"年"+nmo+"月"+nd+"日 "+nh+"时"+nm+"分"+ns+"秒");
现在时间.setText(ss); //把获得的时间赋给“现在时间”标签
return ss;
}
//************** 响应Document事件,需重写三个方法 *************//
public void changedUpdate(DocumentEvent e){
if(时.getText().equals("")||分.getText().equals("")||秒.getText().equals(""))
{
关机.setEnabled(false);
重启.setEnabled(false);
清空.setEnabled(false);
注销.setEnabled(false);
取消.setEnabled(false);
}
else
{
关机.setEnabled(true);
重启.setEnabled(true);
清空.setEnabled(true);
注销.setEnabled(true);
}
}
public void removeUpdate(DocumentEvent e){
changedUpdate(e);
}
public void insertUpdate(DocumentEvent e){
changedUpdate(e);
}
//*********** 响应事件要执行的方法 *****************//
public void 关机程序(){ //关机
try{Runtime.getRuntime().exec("shutdown.exe -s -t 5");}
catch(IOException e){
JOptionPane.showMessageDialog(this,"执行失败!");
}
}
public void 重启程序(){ //重启
try{Runtime.getRuntime().exec("shutdown.exe -r -t 5");}
catch(IOException e){
JOptionPane.showMessageDialog(this,"执行失败!");
}
}
public void 注销程序(){ //注销
try{Runtime.getRuntime().exec("shutdown.exe -l");}
catch(IOException e){
JOptionPane.showMessageDialog(this,"执行失败!");
}
}
public void 取消程序(){ //取消
try{Runtime.getRuntime().exec("shutdown.exe -a");}
catch(IOException e){
JOptionPane.showMessageDialog(this,"执行失败!");
}
}
//*****************时间到就执行的方法**************//
public void 到时执行(){
if(nh==wh && nm==wm && ns==ws){ //如果设定的时间和现在时间相同就执行
if(标志==1){
关机程序();
}
if(标志==2){
重启程序();
}
if(标志==3){
注销程序();
}
}
}
//****************** 响应各按钮的单击事件 ****************//
public void actionPerformed(ActionEvent e)
{
wh=Integer.parseInt(时.getText());
wm=Integer.parseInt(分.getText());
ws=Integer.parseInt(秒.getText());
if(e.getSource()==关机){
关机.setEnabled(false);
重启.setEnabled(false);
注销.setEnabled(false);
标志=1;
if(wh>=0&&wh<=23&&wm>=0&&wm<=59&&ws>=0&&ws<=59){
动作();
}
else{
JOptionPane.showMessageDialog(this,"时间设置满足:0=
时.requestFocus(); //获得焦点
}
}
if(e.getSource()==重启){
关机.setEnabled(false);
重启.setEnabled(false);
注销.setEnabled(false);
标志=2;
if(wh>=0&&wh<=23&&wm>=0&&wm<=59&&ws>=0&&ws<=59){
动作();
}
else
{
JOptionPane.showMessageDialog(this,"时间设置满足:0=
时.requestFocus(); //获得焦点
}
}
if(e.getSource()==注销){
关机.setEnabled(false);
重启.setEnabled(false);
注销.setEnabled(false);
标志=3;
if(wh>=0&&wh<=23&&wm>=0&&wm<=59&&ws>=0&&ws<=59){
动作();
}
else
{
JOptionPane.showMessageDialog(this,"时间设置满足:0=
时.requestFocus(); //获得焦点
}
}
if(e.getSource()==取消){
取消程序();
取消.setEnabled(false);
关机.setEnabled(true);
重启.setEnabled(true);
注销.setEnabled(true);
}
if(e.getSource()==清空){
时.setText("");
分.setText("");
秒.setText("");
时.requestFocus();
清空.setEnabled(false);
}
}
public void 监听()//时间一到就执行动作
{
new Thread(new Runnable()
{
public void run()
{
while(true)
{
try
{
Thread.sleep(1000);
}
catch(Exception e)
{
e.printStackTrace();
}
到时执行();//关机或重启或注销
}
}
}).start();
}
public void 动作() //检查用户输入是否正确,在正确情况下时间到便执行
{
if(Test()==false)
{
JOptionPane.showMessageDialog(this,"时间设定错误,应设定在现在之后!");
时.requestFocus();
}
else{
JOptionPane.showMessageDialog(this,"时间设定成功!");
取消.setEnabled(true); //定义时间成功后取消按钮可点击
监听();
}
}
public boolean Test(){
boolean b=true;
if(wh
b=false;
else if(wh==nh)
{
if(wm
b=false;
else if(wm==nm)
{
if(ws
b=false;
}
}
return b;
}
}
java自动关闭流 java自动关闭窗口
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
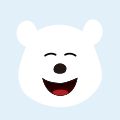
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
TCP关闭连接的方式tcp