#隐式继承(子类完全继承父类):父类有函数,但是子类没有时,
#就会发生子类的动作完全等同于父类上的动作
class parent(object):#父类
def implicit(self):#父类里的函数
print("parent:父辈,implicit:隐式")
class child(parent):#子类
pass#子类留空
dad = parent()
son = child()
dad.implicit()
son.implicit()#子类直接调用父类的函数
parent().implicit()#不用变量也可以,用变量是为了美观和简便
child().implicit()
#显示覆盖(子类完全覆盖父类):在子类中定义一个相同名称的函数,
#可以让子类的函数在继承父类时有一个不同的行为
class parent(object):
def override(self):
print("parent override:父类的重写")
class child(parent):
def override(self):#子类的函数名称和父类里的函数名字要一样
print("child override:子类的重写")
dad = parent()
son = child()
dad.override()
son.override()#子类的函数覆盖了父类的函数,实现自己独有的动作
#(子类部分覆盖父类):在父类的函数运行之前或者运行之后,
#再覆盖子类的函数,达到子类部分覆盖父类函数的效果
class parent(object):
def altered(self):
print("parent altered:父类的改变")
class child(parent):
def altered(self):
print("子类覆盖父类的函数之前")#子类自己的动作
super(child,self).altered()
#super(子类名,self).父类函数名(),调用父类函数动作
#完整解释:用“子类名”和“self”这两个参数
#调用super函数,然后参数返回到父类函数中
print("子类覆盖父类函数之后")#回到子类自己的动作
dad = parent()
son = child()
dad.altered()
son.altered()
#最终版本:
print("................")
class parent(object):
def override(self):
print("父类的重写")
def implicit(self):
print("父类的隐式")
def altered(self):
print("父类的改变")
class child(parent):
def override(self):
print("子类的重写")
def altered(self):
print("子类覆盖父类的函数之前")
super(child,self).altered()
print("子类覆盖父类函数之后")
dad = parent()
son = child()
dad.implicit()
son.implicit()
print("-"*20)
dad.override()
son.override()
print("-"*20)
dad.altered()
print("-"*20)
son.altered()
print("-"*20)
print("1、(完全覆盖)子类有和父类同名的函数时,用子类自己的函数内容")
print("2、(继承父类)子类用的函数名在子类中没有,而在父类中有时,用父类的函数内容")
print("3、(部分覆盖)子类和父类有同名的函数,但是子类函数里有super函数时,"
"super这一行的函数用父类里的内容。非super同行的函数继续用子类自己的内容")
class child(parent):
def __init__(self,stuff):
self.stuff = stuff
super(child,self).__init__()
#super常和__init__函数配合使用,对子类做一些事情,然后再对父类进行初始化
#合成模式,如下所示,child直接使用Other这个类,或者别的模块也可以,不是继承Other
#这里不是父子类child是Other的关系,而是child里面有Other的关系。
class Other(object):#一个父类
def override(self):
print("Other的重写")
def implicit(self):
print("Other的隐式")
def altered(self):
print("Other的改变")
class child(object):#也是一个独立的父类
def __init__(self):
self.other=Other()
#对child这个大类做一个初始化,然后在child这个类里设置一个self.other代表Other()的变量
def implicit(self):
self.other.implicit()
def override(self):
print("子类的重写")
def altered(self):
print("子类覆盖之前")
self.other.altered()
print("子类覆盖之后")
son = child()
son.implicit()
son.override()
son.altered()
python执行父类方法吗 python 父类
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
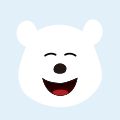
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
python继承父类方法吗 python 继承父类init
使用Python写过面向对象的代码的同学,可能对 __init__ 方法已经非常熟悉了,__init__方法在类的一个对象被建立时,马上运行。这个方法可以用来对你的对象做一些你希望的 初始化 。
python继承父类方法吗 什么是python中子类父类 父类 python 子类 -
python 调用父类 python调用父类静态方法
python 调用父类 python调用父类静态方法
python 调用父类 python 父类 类名 -
python调用父类方法 调用父类的方法
调用父类方法的三种方式:
python调用父类方法 父类 类继承