[size=large]1、发送图片+文字
要特别注意,图片的文件名要为 pic 才会被新浪接收[/size]。
Map map = new HashMap();
map.put("source", "appkey");//改成自己的key
map.put("status", txt);
postImg("http://api.t.sina.com.cn/statuses/upload.json",map,Environment.getExternalStorageDirectory()+ "/temp.jpg"
,"帐号名字","密码");
/**
* 直接通过HTTP协议提交数据到服务器,实现表单提交功能
* @param actionUrl 上传路径
* @param params 请求参数 key为参数名,value为参数值
* @param filename 上传文件
* @param username 用户名
* @param password 密码
*/
private void postImg(String actionUrl,Map<String, String> params, String filename,String username,String password) {
try {
String BOUNDARY = "--------------et567z"; //数据分隔线
String MULTIPART_FORM_DATA = "Multipart/form-data";
URL url = new URL(actionUrl);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setDoInput(true);//允许输入
conn.setDoOutput(true);//允许输出
conn.setUseCaches(false);//不使用Cache
conn.setRequestMethod("POST");
conn.setRequestProperty("Connection", "Keep-Alive");
conn.setRequestProperty("Charset", "UTF-8");
conn.setRequestProperty("Content-Type", MULTIPART_FORM_DATA + ";boundary=" + BOUNDARY);
String usernamePassword=username+":"+password;
conn.setRequestProperty("Authorization","Basic "+new String(SecBase64.encode(usernamePassword.getBytes())));
StringBuilder sb = new StringBuilder();
//上传的表单参数部分,格式请参考文章
for (Map.Entry<String, String> entry : params.entrySet()) {//构建表单字段内容
sb.append("--");
sb.append(BOUNDARY);
sb.append("\r\n");
sb.append("Content-Disposition: form-data; name=\""+ entry.getKey() + "\"\r\n\r\n");
sb.append(entry.getValue());
sb.append("\r\n");
}
// System.out.println(sb.toString());
DataOutputStream outStream = new DataOutputStream(conn.getOutputStream());
outStream.write(sb.toString().getBytes());//发送表单字段数据
byte[] content = readFileImage(filename);
//上传的文件部分,格式请参考文章
//System.out.println("content:"+content.toString());
StringBuilder split = new StringBuilder();
split.append("--");
split.append(BOUNDARY);
split.append("\r\n");
split.append("Content-Disposition: form-data;name=\"pic\";filename=\"temp.jpg\"\r\n");
split.append("Content-Type: image/jpg\r\n\r\n");
System.out.println(split.toString());
outStream.write(split.toString().getBytes());
outStream.write(content, 0, content.length);
outStream.write("\r\n".getBytes());
byte[] end_data = ("--" + BOUNDARY + "--\r\n").getBytes();//数据结束标志
outStream.write(end_data);
outStream.flush();
int cah = conn.getResponseCode();
// if (cah != 200) throw new RuntimeException("请求url失败:"+cah);
if(cah == 200)//如果发布成功则提示成功
{
/*读返回数据*/
//String strResult = EntityUtils.toString(httpResponse.getEntity());
new AlertDialog.Builder(Main.this)
// Main.this视情况而定,这个一般是指当前显示的Activity对应的XML视窗。
.setTitle("")// 设置对话框的标题
.setPositiveButton("确定",// 设置对话框的确认按钮
new DialogInterface.OnClickListener() {// 设置确认按钮的事件
public void onClick(DialogInterface dialog, int which) {
}})
.setMessage(" 发布成功 ")// 设置对话框的内容
.show();
}
else if(cah == 400)
{
new AlertDialog.Builder(Main.this)
// Main.this视情况而定,这个一般是指当前显示的Activity对应的XML视窗。
.setTitle("")// 设置对话框的标题
.setPositiveButton("确定",// 设置对话框的确认按钮
new DialogInterface.OnClickListener() {// 设置确认按钮的事件
public void onClick(DialogInterface dialog, int which) {
}})
.setMessage(" 发布失败 \n 不可连续发布相同内容 ")// 设置对话框的内容
.show();
}else{
throw new RuntimeException("请求url失败:"+cah);
}
// InputStream is = conn.getInputStream();
// int ch;
// StringBuilder b = new StringBuilder();
// while( (ch = is.read()) != -1 ){
// b.append((char)ch);
// }
outStream.close();
conn.disconnect();
}
catch (IOException e)
{
e.printStackTrace();
}
catch (Exception e)
{
e.printStackTrace();
}
}
public static byte[] readFileImage(String filename) throws IOException {
BufferedInputStream bufferedInputStream = new BufferedInputStream(
new FileInputStream(filename));
int len = bufferedInputStream.available();
byte[] bytes = new byte[len];
int r = bufferedInputStream.read(bytes);
if (len != r) {
bytes = null;
throw new IOException("读取文件不正确");
}
bufferedInputStream.close();
return bytes;
}
[size=large]2、只发文字[/size]
//POST发布文本信息
public void sendMsg(String status,String username,String password){
HttpClient httpclient = new DefaultHttpClient();
HttpPost httppost = new HttpPost("http://api.t.sina.com.cn/statuses/update.json");
//NameValuePair实现请求参数的封装
List params = new ArrayList ();
params.add(new BasicNameValuePair("source", "4016954419"));
params.add(new BasicNameValuePair("status", status));
try
{
//添加请求参数到请求对象
httppost.setEntity(new UrlEncodedFormEntity(params, HTTP.UTF_8));
httppost.getParams().setBooleanParameter(CoreProtocolPNames.USE_EXPECT_CONTINUE, false);
String data=username+":"+password;
httppost.addHeader("Authorization","Basic "+new String(SecBase64.encode(data.getBytes())));
httppost.addHeader("Content-Type", "application/x-www-form-urlencoded");
//发送请求并等待响应
HttpResponse httpResponse = new DefaultHttpClient().execute(httppost);
//若状态码为200 ok
if(httpResponse.getStatusLine().getStatusCode() == 200)
{
//读返回数据
//String strResult = EntityUtils.toString(httpResponse.getEntity());
new AlertDialog.Builder(Main.this)
// Main.this视情况而定,这个一般是指当前显示的Activity对应的XML视窗。
.setTitle("")// 设置对话框的标题
.setPositiveButton("确定",// 设置对话框的确认按钮
new DialogInterface.OnClickListener() {// 设置确认按钮的事件
public void onClick(DialogInterface dialog, int which) {
}})
.setMessage(" 发布成功 ")// 设置对话框的内容
.show();
}
else if(httpResponse.getStatusLine().getStatusCode() == 400)
{
new AlertDialog.Builder(Main.this)
// Main.this视情况而定,这个一般是指当前显示的Activity对应的XML视窗。
.setTitle("")// 设置对话框的标题
.setPositiveButton("确定",// 设置对话框的确认按钮
new DialogInterface.OnClickListener() {// 设置确认按钮的事件
public void onClick(DialogInterface dialog, int which) {
}})
.setMessage(" 发布失败 \n 不可连续发布相同内容 ")// 设置对话框的内容
.show();
}
}
catch (ClientProtocolException e)
{
e.printStackTrace();
et.setText(et.getText()+" Error1:"+e.getMessage());
}
catch (IOException e)
{
e.printStackTrace();
et.setText(et.getText()+" Error2:"+e.getMessage());
}
catch (Exception e)
{
e.printStackTrace();
et.setText(et.getText()+" Error3:"+e.getMessage());
}
}
[size=large]3、加密类 SecBase64.java[/size]
package wizzer.cn.app;
public class SecBase64 {
private static final byte[] encodingTable = { (byte) 'A', (byte) 'B',
(byte) 'C', (byte) 'D', (byte) 'E', (byte) 'F', (byte) 'G',
(byte) 'H', (byte) 'I', (byte) 'J', (byte) 'K', (byte) 'L',
(byte) 'M', (byte) 'N', (byte) 'O', (byte) 'P', (byte) 'Q',
(byte) 'R', (byte) 'S', (byte) 'T', (byte) 'U', (byte) 'V',
(byte) 'W', (byte) 'X', (byte) 'Y', (byte) 'Z', (byte) 'a',
(byte) 'b', (byte) 'c', (byte) 'd', (byte) 'e', (byte) 'f',
(byte) 'g', (byte) 'h', (byte) 'i', (byte) 'j', (byte) 'k',
(byte) 'l', (byte) 'm', (byte) 'n', (byte) 'o', (byte) 'p',
(byte) 'q', (byte) 'r', (byte) 's', (byte) 't', (byte) 'u',
(byte) 'v', (byte) 'w', (byte) 'x', (byte) 'y', (byte) 'z',
(byte) '0', (byte) '1', (byte) '2', (byte) '3', (byte) '4',
(byte) '5', (byte) '6', (byte) '7', (byte) '8', (byte) '9',
(byte) '+', (byte) '/' };
private static final byte[] decodingTable;
static {
decodingTable = new byte[128];
for (int i = 0; i < 128; i++) {
decodingTable[i] = (byte) -1;
}
for (int i = 'A'; i <= 'Z'; i++) {
decodingTable[i] = (byte) (i - 'A');
}
for (int i = 'a'; i <= 'z'; i++) {
decodingTable[i] = (byte) (i - 'a' + 26);
}
for (int i = '0'; i <= '9'; i++) {
decodingTable[i] = (byte) (i - '0' + 52);
}
decodingTable['+'] = 62;
decodingTable['/'] = 63;
}
//加密
public static byte[] encode(byte[] data) {
byte[] bytes;
int modulus = data.length % 3;
if (modulus == 0) {
bytes = new byte[(4 * data.length) / 3];
} else {
bytes = new byte[4 * ((data.length / 3) + 1)];
}
int dataLength = (data.length - modulus);
int a1;
int a2;
int a3;
for (int i = 0, j = 0; i < dataLength; i += 3, j += 4) {
a1 = data[i] & 0xff;
a2 = data[i + 1] & 0xff;
a3 = data[i + 2] & 0xff;
bytes[j] = encodingTable[(a1 >>> 2) & 0x3f];
bytes[j + 1] = encodingTable[((a1 << 4) | (a2 >>> 4)) & 0x3f];
bytes[j + 2] = encodingTable[((a2 << 2) | (a3 >>> 6)) & 0x3f];
bytes[j + 3] = encodingTable[a3 & 0x3f];
}
int b1;
int b2;
int b3;
int d1;
int d2;
switch (modulus) {
case 0:
break;
case 1:
d1 = data[data.length - 1] & 0xff;
b1 = (d1 >>> 2) & 0x3f;
b2 = (d1 << 4) & 0x3f;
bytes[bytes.length - 4] = encodingTable[b1];
bytes[bytes.length - 3] = encodingTable[b2];
bytes[bytes.length - 2] = (byte) '=';
bytes[bytes.length - 1] = (byte) '=';
break;
case 2:
d1 = data[data.length - 2] & 0xff;
d2 = data[data.length - 1] & 0xff;
b1 = (d1 >>> 2) & 0x3f;
b2 = ((d1 << 4) | (d2 >>> 4)) & 0x3f;
b3 = (d2 << 2) & 0x3f;
bytes[bytes.length - 4] = encodingTable[b1];
bytes[bytes.length - 3] = encodingTable[b2];
bytes[bytes.length - 2] = encodingTable[b3];
bytes[bytes.length - 1] = (byte) '=';
break;
}
return bytes;
}
//解密
public static byte[] decode(byte[] data) {
byte[] bytes;
byte b1;
byte b2;
byte b3;
byte b4;
data = discardNonBase64Bytes(data);
if (data[data.length - 2] == '=') {
bytes = new byte[(((data.length / 4) - 1) * 3) + 1];
} else if (data[data.length - 1] == '=') {
bytes = new byte[(((data.length / 4) - 1) * 3) + 2];
} else {
bytes = new byte[((data.length / 4) * 3)];
}
for (int i = 0, j = 0; i < (data.length - 4); i += 4, j += 3) {
b1 = decodingTable[data[i]];
b2 = decodingTable[data[i + 1]];
b3 = decodingTable[data[i + 2]];
b4 = decodingTable[data[i + 3]];
bytes[j] = (byte) ((b1 << 2) | (b2 >> 4));
bytes[j + 1] = (byte) ((b2 << 4) | (b3 >> 2));
bytes[j + 2] = (byte) ((b3 << 6) | b4);
}
if (data[data.length - 2] == '=') {
b1 = decodingTable[data[data.length - 4]];
b2 = decodingTable[data[data.length - 3]];
bytes[bytes.length - 1] = (byte) ((b1 << 2) | (b2 >> 4));
} else if (data[data.length - 1] == '=') {
b1 = decodingTable[data[data.length - 4]];
b2 = decodingTable[data[data.length - 3]];
b3 = decodingTable[data[data.length - 2]];
bytes[bytes.length - 2] = (byte) ((b1 << 2) | (b2 >> 4));
bytes[bytes.length - 1] = (byte) ((b2 << 4) | (b3 >> 2));
} else {
b1 = decodingTable[data[data.length - 4]];
b2 = decodingTable[data[data.length - 3]];
b3 = decodingTable[data[data.length - 2]];
b4 = decodingTable[data[data.length - 1]];
bytes[bytes.length - 3] = (byte) ((b1 << 2) | (b2 >> 4));
bytes[bytes.length - 2] = (byte) ((b2 << 4) | (b3 >> 2));
bytes[bytes.length - 1] = (byte) ((b3 << 6) | b4);
}
return bytes;
}
//解密
public static byte[] decode(String data) {
byte[] bytes;
byte b1;
byte b2;
byte b3;
byte b4;
data = discardNonBase64Chars(data);
if (data.charAt(data.length() - 2) == '=') {
bytes = new byte[(((data.length() / 4) - 1) * 3) + 1];
} else if (data.charAt(data.length() - 1) == '=') {
bytes = new byte[(((data.length() / 4) - 1) * 3) + 2];
} else {
bytes = new byte[((data.length() / 4) * 3)];
}
for (int i = 0, j = 0; i < (data.length() - 4); i += 4, j += 3) {
b1 = decodingTable[data.charAt(i)];
b2 = decodingTable[data.charAt(i + 1)];
b3 = decodingTable[data.charAt(i + 2)];
b4 = decodingTable[data.charAt(i + 3)];
bytes[j] = (byte) ((b1 << 2) | (b2 >> 4));
bytes[j + 1] = (byte) ((b2 << 4) | (b3 >> 2));
bytes[j + 2] = (byte) ((b3 << 6) | b4);
}
if (data.charAt(data.length() - 2) == '=') {
b1 = decodingTable[data.charAt(data.length() - 4)];
b2 = decodingTable[data.charAt(data.length() - 3)];
bytes[bytes.length - 1] = (byte) ((b1 << 2) | (b2 >> 4));
} else if (data.charAt(data.length() - 1) == '=') {
b1 = decodingTable[data.charAt(data.length() - 4)];
b2 = decodingTable[data.charAt(data.length() - 3)];
b3 = decodingTable[data.charAt(data.length() - 2)];
bytes[bytes.length - 2] = (byte) ((b1 << 2) | (b2 >> 4));
bytes[bytes.length - 1] = (byte) ((b2 << 4) | (b3 >> 2));
} else {
b1 = decodingTable[data.charAt(data.length() - 4)];
b2 = decodingTable[data.charAt(data.length() - 3)];
b3 = decodingTable[data.charAt(data.length() - 2)];
b4 = decodingTable[data.charAt(data.length() - 1)];
bytes[bytes.length - 3] = (byte) ((b1 << 2) | (b2 >> 4));
bytes[bytes.length - 2] = (byte) ((b2 << 4) | (b3 >> 2));
bytes[bytes.length - 1] = (byte) ((b3 << 6) | b4);
}
return bytes;
}
private static byte[] discardNonBase64Bytes(byte[] data) {
byte[] temp = new byte[data.length];
int bytesCopied = 0;
for (int i = 0; i < data.length; i++) {
if (isValidBase64Byte(data[i])) {
temp[bytesCopied++] = data[i];
}
}
byte[] newData = new byte[bytesCopied];
System.arraycopy(temp, 0, newData, 0, bytesCopied);
return newData;
}
private static String discardNonBase64Chars(String data) {
StringBuffer sb = new StringBuffer();
int length = data.length();
for (int i = 0; i < length; i++) {
if (isValidBase64Byte((byte) (data.charAt(i)))) {
sb.append(data.charAt(i));
}
}
return sb.toString();
}
private static boolean isValidBase64Byte(byte b) {
if (b == '=') {
return true;
} else if ((b < 0) || (b >= 128)) {
return false;
} else if (decodingTable[b] == -1) {
return false;
}
return true;
}
//测试类
public static void main(String[] args) {
String data = "wizzer@qq.com:etpass";
byte[] result = SecBase64.encode(data.getBytes());// 加密
System.out.println("Basic "+data);
System.out.println("Basic "+new String(result));
System.out.println(new String(SecBase64.decode(new String(result))));// 解密
}
}
android上传文件前端代码 android post上传文件
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
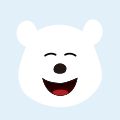
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
android post文件上传 android 上传文件夹
在局域网内,实现从android客户端把手机SD卡上的文件上传到PC服务器端,并保存在PC硬盘的指定文件夹下。同时把PC端硬盘文件的目录和对文件的描述信息保存在mysql数据库中。
android post文件上传 android 服务器 http协议 java -
Android 调接口上传文件 android post上传文件
Android 调接口上传文件 android post上传文件
android string buffer file byte -
android socket 接收文件上传 android post上传文件
最近在做向服务器提交文件,本来用的xutils,使用起来挺简单,代码超不过10行,但是想深入了解一下原理,所以就自己用HttpURLConnection实现文件的上传。无论是浏览器通过表单提交文件,还是APP通过post提交,其实原理都是一样的。APP只要仿照浏览器的表单提交数据的样式去提交文件,服务器就可以解析并处理文件。那就首先来看一下浏览器提交的数据样式和请求头的信息。HTML核心代码:&l
Stream ended unexpec HttpURLConnection上传文 content-typemultipar Content-Disposition 数据