package demo;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.Enumeration;
import org.apache.tools.zip.ZipEntry;
import org.apache.tools.zip.ZipFile;
public class test01 {
public static void main(String[] args) throws IOException {
//zip文件路径
String fileAddress = "D:\\Android\\workspace\\zip0322\\files\\demo01.zip";
//zip文件解压地址
String unZipAddress = "D:\\Android\\workspace\\zip0322\\files\\";
File file = new File(fileAddress);
ZipFile zipFile = null;
try {
zipFile = new ZipFile(file,"GBK");//设置编码格式
} catch (IOException exception) {
exception.printStackTrace();
System.out.println("解压文件不存在!");
}
Enumeration e = zipFile.getEntries();
while(e.hasMoreElements()) {
ZipEntry zipEntry =(ZipEntry)e.nextElement();
if(zipEntry.isDirectory()){
String name=zipEntry.getName();
name=name.substring(0,name.length()-1);
File f=new File(unZipAddress+name);
f.mkdirs();
}else {
File f = new File(unZipAddress+zipEntry.getName());
f.getParentFile().mkdirs();
f.createNewFile();
InputStream is=zipFile.getInputStream(zipEntry);
FileOutputStream fos=new FileOutputStream(f);
int length=0;
byte[] b=new byte[1024];
while((length=is.read(b,0,1024))!=-1){
fos.write(b, 0, length);
}
is.close();
fos.close();
}
}
if(zipFile != null){
zipFile.close();
}
//file.deleteOnExit();
System.out.println("ok");
}
}
java 解压zip 包含多个csv java实现解压zip文件
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
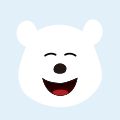
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
Java实现解压ZIP文件
一、概述 这篇文章主要是
java zip压缩 工具类 Java -
java中zip解压漏洞 java实现zip解压
介绍Java提供的java.util.zip包只支持zip和gzip。至于更多格式的压缩可以选择apache的Commons Compress。
java zip java List System