使用Java的两个数组的递归组合项(非空或重复)(Recursive Combinations Items of Two Arrays (not null or repeated), using Java)
我有两个数组(长度相同),大小可变。
为了做最简单的例子,我有两个长度= 3的数组。
Persons -> P1, P2, P3
Seats -> Sa, Sb, Sc
我需要生成所有组合Cn
如果人P1拿Sa ,可能是:
人P2采取Sb然后P3将采取Sc 。 组合将是P1Sa, P2Sb, P3Sc 。
或者P2取Sc然后P3取Sb 。 组合将是P1Sa, P2Sc, P3Sb 。
现在这里所有可能的组合。
C0 -> P1Sa, P2Sb, P3Sc
C1 -> P1Sa, P2Sc, P3Sb
C2 -> P1Sb, P2Sa, P3Sc
C3 -> P1Sb, P2Sc, P3Sa
C4 -> P1Sc, P2Sa, P3Sb
C5 -> P1Sc, P2Sb, P3Sa
这是我糟糕的设计。
private void recursiveSeatPerson(String c, List p, List s) {
if (p.size() > 1) {
for (int i = 0; i < s.size(); i++) {
String combination = p.get(0)+s.get(i) + ", " + c;
System.out.print(combination);
List new_s = new ArrayList<>();
for (int index_s = 0; index_s < s.size(); index_s++) {
if (index_s != i) {
new_s.add(s.get(i));
}
}
List new_p = new ArrayList<>();
for (int index_p = 1; index_p < p.size(); index_p++) {
new_p.add(p.get(index_p));
}
recursiveSeatPerson(combination, new_p, new_s);
}
} else {
System.out.print(c + p.get(0)+s.get(0) + " ");
System.out.println();
}
}
这是我的测试。
List persons = Arrays.asList("P1", "P2", "P3");
List seats = Arrays.asList("Sa", "Sb", "Sc");
recursiveSeatPerson("", persons, seats);
这是我的结果:
P1Sa, P2Sa, P1Sa, P2Sa, P1Sa, P3Sa
P2Sa, P1Sa, P2Sa, P1Sa, P3Sa
P1Sb, P2Sb, P1Sb, P2Sb, P1Sb, P3Sb
P2Sb, P1Sb, P2Sb, P1Sb, P3Sb
P1Sc, P2Sc, P1Sc, P2Sc, P1Sc, P3Sc
P2Sc, P1Sc, P2Sc, P1Sc, P3Sc
现在在此行中发送类似参数的空String:
recursiveSeatPerson("", new_p, new_s);
这是糟糕的结果(再次)。
P1Sa, P2Sa, P3Sa
P2Sa, P3Sa
P1Sb, P2Sb, P3Sb
P2Sb, P3Sb
P1Sc, P2Sc, P3Sc
P2Sc, P3Sc
如何解决我的代码?
I have two arrays (with same length), the size is variable.
To do simplest example I have two arrays with length=3.
Persons -> P1, P2, P3
Seats -> Sa, Sb, Sc
I need generate all combinations Cn
If person P1 take Sa, is possible that:
person P2 take Sb then P3 will take Sc. The combination will be P1Sa, P2Sb, P3Sc.
or Person P2 take Scthen P3 will take Sb. The combination will be P1Sa, P2Sc, P3Sb.
Now Here all possible combinations.
C0 -> P1Sa, P2Sb, P3Sc
C1 -> P1Sa, P2Sc, P3Sb
C2 -> P1Sb, P2Sa, P3Sc
C3 -> P1Sb, P2Sc, P3Sa
C4 -> P1Sc, P2Sa, P3Sb
C5 -> P1Sc, P2Sb, P3Sa
This is my bad design.
private void recursiveSeatPerson(String c, List p, List s) {
if (p.size() > 1) {
for (int i = 0; i < s.size(); i++) {
String combination = p.get(0)+s.get(i) + ", " + c;
System.out.print(combination);
List new_s = new ArrayList<>();
for (int index_s = 0; index_s < s.size(); index_s++) {
if (index_s != i) {
new_s.add(s.get(i));
}
}
List new_p = new ArrayList<>();
for (int index_p = 1; index_p < p.size(); index_p++) {
new_p.add(p.get(index_p));
}
recursiveSeatPerson(combination, new_p, new_s);
}
} else {
System.out.print(c + p.get(0)+s.get(0) + " ");
System.out.println();
}
}
This is my Testing.
List persons = Arrays.asList("P1", "P2", "P3");
List seats = Arrays.asList("Sa", "Sb", "Sc");
recursiveSeatPerson("", persons, seats);
This is my results:
P1Sa, P2Sa, P1Sa, P2Sa, P1Sa, P3Sa
P2Sa, P1Sa, P2Sa, P1Sa, P3Sa
P1Sb, P2Sb, P1Sb, P2Sb, P1Sb, P3Sb
P2Sb, P1Sb, P2Sb, P1Sb, P3Sb
P1Sc, P2Sc, P1Sc, P2Sc, P1Sc, P3Sc
P2Sc, P1Sc, P2Sc, P1Sc, P3Sc
Now sending empty String like argument in this line:
recursiveSeatPerson("", new_p, new_s);
This is the bad result (again).
P1Sa, P2Sa, P3Sa
P2Sa, P3Sa
P1Sb, P2Sb, P3Sb
P2Sb, P3Sb
P1Sc, P2Sc, P3Sc
P2Sc, P3Sc
How solve my code?
原文:https://stackoverflow.com/questions/49806512
更新时间:2020-01-05 19:55
最满意答案
工作代码:
private void recursiveSeatPerson(String snippet, List p, List s) {
if (p.size() > 1) {
for (int i = 0; i < s.size(); i++) {
String combination = snippet + p.get(0) + s.get(i) + ", ";
List new_s = new ArrayList<>();
for (int index_s = 0; index_s < s.size(); index_s++) {
if (index_s != i) {
new_s.add(s.get(index_s));
}
}
List new_p = new ArrayList<>();
for (int index_p = 1; index_p < p.size(); index_p++) {
new_p.add(p.get(index_p));
}
recursiveSeatPerson(combination, new_p, new_s);
}
} else {
System.out.println(snippet + p.get(0)+s.get(0) + " ");
}
}
使用4个长度的阵列进行测试。
List persons = Arrays.asList("P1", "P2", "P3", "P4");
List seats = Arrays.asList("Sa", "Sb", "Sc", "Sd");
recursiveSeatPerson("", persons, seats);
检查输出:
P1Sa, P2Sb, P3Sc, P4Sd
P1Sa, P2Sb, P3Sd, P4Sc
P1Sa, P2Sc, P3Sb, P4Sd
P1Sa, P2Sc, P3Sd, P4Sb
P1Sa, P2Sd, P3Sb, P4Sc
P1Sa, P2Sd, P3Sc, P4Sb
P1Sb, P2Sa, P3Sc, P4Sd
P1Sb, P2Sa, P3Sd, P4Sc
P1Sb, P2Sc, P3Sa, P4Sd
P1Sb, P2Sc, P3Sd, P4Sa
P1Sb, P2Sd, P3Sa, P4Sc
P1Sb, P2Sd, P3Sc, P4Sa
P1Sc, P2Sa, P3Sb, P4Sd
P1Sc, P2Sa, P3Sd, P4Sb
P1Sc, P2Sb, P3Sa, P4Sd
P1Sc, P2Sb, P3Sd, P4Sa
P1Sc, P2Sd, P3Sa, P4Sb
P1Sc, P2Sd, P3Sb, P4Sa
P1Sd, P2Sa, P3Sb, P4Sc
P1Sd, P2Sa, P3Sc, P4Sb
P1Sd, P2Sb, P3Sa, P4Sc
P1Sd, P2Sb, P3Sc, P4Sa
P1Sd, P2Sc, P3Sa, P4Sb
P1Sd, P2Sc, P3Sb, P4Sa
Working code:
private void recursiveSeatPerson(String snippet, List p, List s) {
if (p.size() > 1) {
for (int i = 0; i < s.size(); i++) {
String combination = snippet + p.get(0) + s.get(i) + ", ";
List new_s = new ArrayList<>();
for (int index_s = 0; index_s < s.size(); index_s++) {
if (index_s != i) {
new_s.add(s.get(index_s));
}
}
List new_p = new ArrayList<>();
for (int index_p = 1; index_p < p.size(); index_p++) {
new_p.add(p.get(index_p));
}
recursiveSeatPerson(combination, new_p, new_s);
}
} else {
System.out.println(snippet + p.get(0)+s.get(0) + " ");
}
}
Testing with a 4 length arrays.
List persons = Arrays.asList("P1", "P2", "P3", "P4");
List seats = Arrays.asList("Sa", "Sb", "Sc", "Sd");
recursiveSeatPerson("", persons, seats);
Check the output:
P1Sa, P2Sb, P3Sc, P4Sd
P1Sa, P2Sb, P3Sd, P4Sc
P1Sa, P2Sc, P3Sb, P4Sd
P1Sa, P2Sc, P3Sd, P4Sb
P1Sa, P2Sd, P3Sb, P4Sc
P1Sa, P2Sd, P3Sc, P4Sb
P1Sb, P2Sa, P3Sc, P4Sd
P1Sb, P2Sa, P3Sd, P4Sc
P1Sb, P2Sc, P3Sa, P4Sd
P1Sb, P2Sc, P3Sd, P4Sa
P1Sb, P2Sd, P3Sa, P4Sc
P1Sb, P2Sd, P3Sc, P4Sa
P1Sc, P2Sa, P3Sb, P4Sd
P1Sc, P2Sa, P3Sd, P4Sb
P1Sc, P2Sb, P3Sa, P4Sd
P1Sc, P2Sb, P3Sd, P4Sa
P1Sc, P2Sd, P3Sa, P4Sb
P1Sc, P2Sd, P3Sb, P4Sa
P1Sd, P2Sa, P3Sb, P4Sc
P1Sd, P2Sa, P3Sc, P4Sb
P1Sd, P2Sb, P3Sa, P4Sc
P1Sd, P2Sb, P3Sc, P4Sa
P1Sd, P2Sc, P3Sa, P4Sb
P1Sd, P2Sc, P3Sb, P4Sa
2018-04-13
相关问答
列出monad 谁写了那么长的关于划定的问题的东西是坚果 - 在我花了3个小时试图搞清楚之后,我将在30秒内忘记它的一切! 更严重的是,与这个答案相比, shift / reset是如此令人难以置信的不切实际,这是一个笑话。 但是,如果我没有先分享这个答案,我们就不会有把内心彻底改变的快乐! 所以,请不要进行shift / reset除非他们对手头的任务至关重要 - 请原谅我,如果你觉得自己被学习的东西弄得很酷! 让我们不要忽视一个更直接的解决方案,List monad - 在这里用Array.p
...
解决任何参数(只要结果是可数的) 编辑 :该版本避免了len = Math.pow(left.length, right.length)和长度超过36(cnr!)的问题。 怎么运行的: 例如: combine(['A', 'B'], [1, 2, 3])所有可能的行都是2 ^ 3 = 8。分配在这个例子中是二进制的,但是具有更多的left参数它改变了基数。 distribution included in set
i c A B
...
Group.Where(x => x != null)
.SelectMany(g => combination.Where(c => c != null)
.Select(c => new {Group = g, Combination = c}));
或者: from g in Group where g != null
from c in combination where c != null
select new
...
要合并数组(不删除重复),请使用Array.concat : var array1 = ["Vijendra","Singh"];
var array2 = ["Singh", "Shakya"];
var array3 = array1.concat(array2); // Merges both arrays
// [ 'Vijendra', 'Singh', 'Singh', 'Shakya' ]
因为没有“内置”的方式来删除重复( ECMA-262实际上有Array.forEach这将
...
你必须认为它是Java方式。 完全忘记数组 。 使用两个字段(称为name和price )创建一个类(如Item ),并将每个Item放在LinkedList 。 或者像其他人建议的那样创建一个地图,但这不是那么简单,而且删除数组更加紧迫。 这甚至允许您在之后添加第三个字段,而无需重新考虑您的代码。 它被称为面向对象的编程。 但是在C中你会创建一个包含两个元素的结构,以免发疯。 在Java中,这甚至更简单,因为Java是一种高级的面向对象语言,并且没有内存管理可供您使用。 只需使用编程语言提供的功
...
工作代码: private void recursiveSeatPerson(String snippet, List p, List s) {
if (p.size() > 1) {
for (int i = 0; i < s.size(); i++) {
String combination = snippet + p.get(0) + s.get(i) + ", ";
List new_s = new Ar
...
这被称为cartesian积。 您可以使用ES6功能来实现此目的: reduce和map方法。 在集合论中(通常,在数学的其他部分),笛卡尔积是一种从多个集合返回集合的数学运算。 function cartesianProduct(array) {
return array.reduce((a, b) =>
a.map(x => b.map(y => x.concat(y)))
.reduce((a, b) => a.concat(b), []), [[]]);
}
...
你应该考虑做这样的事情(请记住这是伪代码): group1 = {m1, m2, m3}
group2 = {m4, m5}
group3 = {m6, m7, m8, m9}
len1 = group1.size
len2 = group2.size
len3 = group3.size
max = max(len1, len2, len3)
combination = {}
for i from 0 to max:
combination = {}
if(group1(
...
尝试添加一个contain()方法(注意,这段代码的灵感来自LinkedList代码源,你可以检查一下LinkedList.contains(Object o) ) public boolean contain(T o) {
if (o == null) { // assuming that your list accept to add null object
for (Node x = first; x != null; x = x.next) {
...
如果在两个输入数组都为nil的情况下你可以使用空数组,那么你可以按如下方式合并它: (old_duplicate_items.to_a + new_duplicate_items.to_a).map(&:id)
但这就是我所能看到的一切。 If you're ok with an empty array as a result in the case of both input arrays being nil, then you could consolidate it as follows:
...
java定义双层数组 java双重数组
转载文章标签 java定义双层数组 java递归处理多层数组 List System 数组 文章分类 Java 后端开发
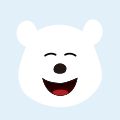
-
Java 中数组的使用(一维数组和二维数组)
本文详细的介绍了,在Java 中数组的使用,并对其内存存在形式进行了详细的分析。
数组 一维数组 二维数组 java基础 -
在Java中使用二维数组生成迷宫
在Java中使用二维数组生成迷宫
深度优先搜索 Java 二维数组 -
mongodbshell如何启动
DOS(Windows)端启动 MongoDB 服务配置环境变量 在电脑->属性->环境变量->Path中添加 MongoDB 安装路径(根据自己的安装路径添加),如图 1 所示: &n
mongodbshell如何启动 mongodb 数据库 配置文件 日志文件