Ajax:
通过异步请求 不刷新页面的情况下通过javascript与服务器端进行通讯
可以达到页面的局部刷新
异步-》 多个执行或者同时执行
同步-》 按照一定的顺序一次执行
ajax 服务器端进行通讯核心的对象 XMLHttpRequest
浏览器上都有这个对象,但不同的浏览器创建方式不一样
使用ajax步骤
1:创建XMLHttpRequest 对象
2:XMLHttpRequest 对象与服务器建立连接
open("请求方式get||post" ,"url",“是否异步请求true||false”,)
3:监听服务器的处理状态(两种:请求的处理状态和响应状态)
指派一个监听函数
XMLHttpRequest 对象
onreadystatechange
readyState (请求的处理状态 )0,连接未建立
1,连接已建立但请求未处理
2,请求在处理中
3,请求在响应中
4 请求处理完成,相应完成
XMLHttpRequest 对象responeseText;服务器的响应值
XMLHttpRequest 对象 status (响应状态 )
5xx--服务器的错误
4xx--客户端页面出现的错误
200-》正常响应状态,服务器
4. send();发送请求
eg:用ajax实现局部刷新
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<script type="text/javascript">
var xmlRequest =null;
//火狐内核浏览器
if (window.XMLHttpRequest) {
xmlRequest=new XMLHttpRequest();
}else{
if (window.ActiveXObject) {
try {
//低版本IE内核浏览器 8以下
xmlRequest=new ActiveXObject("Microsoft.XMLHTTP");
} catch (Exception) {
//高版本IE内核浏览器
xmlRequest=new ActiveXObject("MSXML2.XMLHTTP");
}
}
}
//alert(xmlRequest);
function getMessage(){
//1:创建 XMLHttpRequest ajax核心对象
//2:核心对象.open("请求方式get||post" ,"url",“是否异步请求true||false”,);与服务器建立连接
xmlRequest.open("get", "getMessage", true);
//3.监听服务器的处理状态 0 1 2 3 4
/* 0,连接未建立
1,连接已建立但请求未处理
2,请求在处理中
3,请求在响应中
4 请求处0理完成,相应完成 */
xmlRequest.onreadystatechange =callBack; /* //callBackh后面不需要大括号 */
xhr.setRequestHeader("Content-Type","application/x-www-form-urlencoded");
xmlRequest.send(null);
}
function callBack(){
//5.:判断服务器状态
if (xmlRequest.readyState==4) {
//xmlRequest.status
/* 5xx--服务器的错误
4xx--客户端页面出现的错误
200-》成功处理
*/
if (xmlRequest.status ==200) {
//成功处理,获取服务器返回值 核心对象.responseText;此处在servlet中写入了一个admin字符串,所有在info标签中会显示admin字样
var data =xmlRequest.responseText;
//6:局部刷新
document.getElementById("info").innerHTML=data;
}
}
}
</script>
<title>Insert title here</title>
</head>
<body>
<input type ="button" value="提交" οnclick="getMessage()">
<span id ="info"></span>
</body>
</html>
eg:用ajax来实现显示数据库省份信息
1:先封装好一个createXMLHttpRequest.js文件,内容如下:
function createRequest(){
var xmlHttpRequest =null;
if (window.XMLHttpRequest) {
xmlHttpRequest=new XMLHttpRequest();
}else {
if (window.ActiveXObject) {
try {
xmlHttpRequest=new ActiveXObject("Microsoft.XMLHTTP");
} catch (Exception) {
xmlHttpRequest=new ActiveXObject("MSXML2.XMLHTTP");
}
}
}
return xmlHttpRequest;
2:前端页面代码
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
<script type="text/javascript " src="js/createXMLHttpRequest.js"></script>
<script type="text/javascript">
var xhr = createRequest();//调用createXMLHttpRequest.js文件里的createRequest()方法得到XMLHttpRequest 对象
function loadProvince() {
xhr.open("get", "province", true); //province 是在web.xml文件中配置好的访问servlet路径
xhr.onreadystatechange = callProvince;
xhr.send(null);
}
function callProvince() {
if (xhr.readyState == 4) {
if (xhr.status == 200) {
// alert(xhr.responseText);
var result = xhr.responseText;
var provinces = result.split("|");
//["1,江西","2,江苏"]
var pDom = document.getElementById("provinces");
pDom.options.length = provinces.length+1 ;
pDom.options[0] = new Option("请选择", 0);//默认显示请选择
for (var i = 0; i < provinces.length; i++) {
var str = provinces[i];
// [ "1,江西" ]
var strArray = str.split(",");
// [ "1", "江西" ]
pDom.options[i+1] = new Option(strArray[1], strArray[0]);
}
}
}
}
function loadCity() {
var provincesId = document.getElementById("provinces").value;
xhr.open("get", "getCity?provincesId="+ provincesId, true);
xhr.onreadystatechange = callCity;
xhr.send(null);
}
function callCity() {
if (xhr.readyState == 4) {
if (xhr.status == 200) {
//alert(xhr.responseText);
var result = xhr.responseText;
var provinces = result.split("|");
//["1,江西","2,江苏"]
var pDom = document.getElementById("citys");
pDom.options.length = provinces.length + 1;
pDom.options[0] = new Option("请选择", 0);
for (var i = 0; i < provinces.length; i++) {
var str = provinces[i];//["1,江西"]
var strArray = str.split(",");//["1","江西"]
pDom.options[i+1] = new Option(strArray[1], strArray[0]);
}
}
}
}
</script>
</head>
<body οnlοad="loadProvince()">
省份:
<select id="provinces" οnchange="loadCity()">
</select>
城市:
<select id="citys">
</select>
</body>
</html>
}2.
java怎么处理ajax请求 java中的ajax
转载文章标签 java怎么处理ajax请求 java中Ajax的使用 ajax java xml 文章分类 Java 后端开发
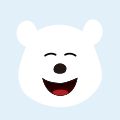
-
java 中ajax操作 java处理ajax请求
1. 什么是 AJAX 请求 AJAX 即“ A synchronous J avascript A nd X ML ” (异步 JavaScript 和 XML ),是指一种创建交互式 网页 应用的网页开发技术。 &nbs
java 中ajax操作 ajax javascript servlet html -
java 发送ajax请求 java处理ajax请求
java后台处理ajax请求_Java后台判断ajax请求及处理过程详解
java后端收到莫名的请求地址怎么回事 java后台处理ajax请求 ajax java User -
java ajax后台 java ajax请求
AJAXAJAX请求jQuery使用Ajax请求$.ajax 方法 . g e
java ajax后台 ajax json jquery AJAX