import java.awt.Graphics2D;
import java.awt.Image;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
import javax.imageio.ImageIO;
public class ImageCutUtils {
public static void main(String[] args){
cutImg("/Users/jinx/Downloads/21.jpg",500,600,"/Users/jinx/Downloads/24.jpg");
}
public static void cutImg(String path,int x,int y,String outPath) {//图片路径,截取位置坐标,输出新突破路径
try {
File sourcePic = new File(path);
File outPic = new File(outPath);
BufferedImage pic = ImageIO.read(sourcePic);
int imgwidth = pic.getWidth();
int imgheight = pic.getHeight();
int widthInt = imgwidth/4;//截取宽度为原图的四分之一宽
int heightInt = imgheight/4;//截取高度为原图的四分之一高
//参数依次为,截取起点的x坐标,y坐标,截取宽度,截取高度
BufferedImage pic2 = pic.getSubimage(x, y, widthInt, heightInt);
//将截取的子图另行存储
Image _img = pic2.getScaledInstance(imgwidth, imgheight, Image.SCALE_DEFAULT);
BufferedImage image = new BufferedImage(imgwidth, imgheight, BufferedImage.TYPE_INT_RGB);
Graphics2D graphics = image.createGraphics();
graphics.drawImage(_img, 0, 0, null);
graphics.dispose();
OutputStream out = new FileOutputStream(outPic);
ImageIO.write(image, "jpg", out);
out.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
java截取unity图片 java截取图片的一部分
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
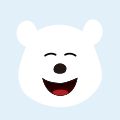
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章