public static Map po2Map(Object po) {
Map poMap = new HashMap();
Map map = new HashMap();
try {
map = BeanUtils.describe(po);
} catch (Exception ex) {
}
Object[] keyArray = map.keySet().toArray();
for (int i = 0; i < keyArray.length; i++) {
String str = keyArray[i].toString();
if (str != null && !str.equals("class")) {
if (map.get(str) != null) {
poMap.put(str, map.get(str));
}
}
}
Method[] ms =po.getClass().getMethods();
for(Method m:ms){
String name = m.getName();
if(name.startsWith("get")){
if(m.getAnnotation(NotDbField.class)!=null){
poMap.remove(getFieldName(name));
}
}
}
return poMap;
}
public void insert(String table, Object po) {
Map poMap = ReflectionUtil.po2Map(po);
table = this.dbRouter.getTableName( table);
this.jdbcDaoSupport.insert(table, poMap);
}
public void insert(String table, Map fields) {
String sql = "";
try {
Assert.hasText(table, "表名不能为空");
Assert.notEmpty(fields, "字段不能为空");
table = quoteCol(table);
Object[] cols = fields.keySet().toArray();
Object[] values = new Object[cols.length];
for (int i = 0; i < cols.length; i++) {
if (fields.get(cols[i]) == null) {
values[i] = null;
} else {
values[i] = fields.get(cols[i]).toString();
}
cols[i] = quoteCol(cols[i].toString());
}
sql = "INSERT INTO " + table + " ("
+ StringUtil.implode(", ", cols) + ") VALUES ("
+ StringUtil.implodeValue(", ", values) + ")";
jdbcTemplate.update(sql, values);
} catch (Exception e) {
e.printStackTrace();
throw new DBRuntimeException(e, sql);
}
}
insert daoSupport
原创mb6444ed45406a4 ©著作权
©著作权归作者所有:来自51CTO博客作者mb6444ed45406a4的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:10个让你变成糟糕的程序员的行为
下一篇:js format date
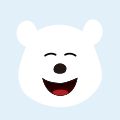
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
根据Excel生成Insert或Update语句
根据Excel生成SQL
EXCEL SQL -
MySQL全文索引源码剖析之Insert语句执行过程
全文索引是信息检索领域的一种常用的技术手段,用于全文搜索问题。
全文索引 mysql MySQL Insert语句