2. public class BaseDaoHibernate extends HibernateDaoSupport implements BaseDao {
3.
4. private static Log log = LogFactory.getLog(BaseDaoHibernate.class);
5.
6. public void save(final Object obj) {
7. getHibernateTemplate().save(obj);
8. // save2(obj);
9. }
10.
11. private Serializable save(Session session, Object p) {
12. Transaction t = session.beginTransaction();
13. Serializable o = session.save(p);
14. t.commit();
15. session.flush();
16. session.close();
17. return o;
18. }
19.
20. public Serializable save2(final Object entity) throws DataAccessException {
21. Object o = getHibernateTemplate().execute(new HibernateCallback() {
22. public Object doInHibernate(final Session session)
23. throws HibernateException {
24. return save(session, entity);
25. }
26. });
27. return null;
28. }
29.
30. public void deleteAll(final Object o) {
31. String hql = " delete from " + o.getClass().getName() + " ";
32. executeUpdate(hql);
33. }
34.
35. /**
36. * 根据查询语句,返回对象列表
37. *
38. * @param hql
39. * 查询语句
40. * @return 符合查询语句的对象列表;最多返回 Constants.maxResults 条
41. */
42. public List find(final String hql) {
43. final int firstResult = 0;
44. return find(hql, firstResult, Constants.MAXRESULTS);
45. }
46.
47. /**
48. * 根据查询语句,返回对象列表
49. *
50. * @param hql
51. * 查询语句
52. * @return 符合查询语句的对象列表;最多返回 Constants.maxResults 条
53. */
54. public List find(final String hql, List tablesName) {
55. final int firstResult = 0;
56. return find(hql, firstResult, Constants.MAXRESULTS);
57. }
58.
59. /**
60. * 返回指定起始位置,指定条数的对象
61. *
62. * @param hql
63. * 查询语句
64. * @param firstResult
65. * 起始位置
66. * @param maxResults
67. * 最多纪录数
68. * @return list 结果列表
69. */
70. public List find(final String hql, final int firstResult,
71. final int maxResults) {
72. if (log.isDebugEnabled()) {
73. log.debug("hql=" + hql);
74. }
75. return getHibernateTemplate().executeFind(new HibernateCallback() {
76. public Object doInHibernate(final Session session)
77. throws HibernateException {
78. try {
79. session.connection().setReadOnly(true);
80. } catch (SQLException e) {
81. // TODO Auto-generated catch block
82. e.printStackTrace();
83. }
84. Query queryObject = session.createQuery(hql);
85. queryObject.setReadOnly(true);
86. queryObject.setFirstResult(firstResult);
87. queryObject.setMaxResults(maxResults);
88. return queryObject.list();
89. }
90. });
91. }
92.
93. /**
94. * 返回指定起始位置,指定条数的对象
95. *
96. * @param hql
97. * 查询语句
98. * @param firstResult
99. * 起始位置
100. * @param maxResults
101. * 最多纪录数
102. * @return list 结果列表
103. */
104. public List findByIterate(final String hql) {
105. return getHibernateTemplate().executeFind(new HibernateCallback() {
106. public Object doInHibernate(final Session session)
107. throws HibernateException {
108. Query queryObject = session.createQuery(hql);
109. queryObject.setCacheable(true);
110. Iterator iter = queryObject.iterate();
111. List ls = new ArrayList();
112. while (iter.hasNext()) {
113. ls.add(iter.next());
114. }
115. return ls;
116. }
117. });
118. }
119.
120. /**
121. * 查询语句需要的条件参数。通过map传递
122. *
123. * @param hql
124. * 查询语句
125. * @param map
126. * 参数
127. * @param firstResult
128. * 起始位置
129. * @param maxResults
130. * 最多纪录数
131. * @return list 结果列表
132. */
133. public List find(final String hql, final Map map, final int firstResult,
134. final int maxResults) {
135. return getHibernateTemplate().executeFind(new HibernateCallback() {
136. public Object doInHibernate(final Session session)
137. throws HibernateException {
138. Query queryObject = session.createQuery(hql);
139. String[] params = queryObject.getNamedParameters();
140. for (int i = 0, max = params.length; i < max; i++) {
141. queryObject.setParameter(params[i], map.get(params[i]));
142. }
143. queryObject.setFirstResult(firstResult);
144. queryObject.setMaxResults(maxResults);
145. return queryObject.list();
146. }
147. });
148. }
149.
150. /**
151. * 根据查询语句,返回对象列表
152. *
153. * @param hql
154. * 查询语句
155. * @return 符合查询语句的对象列表;最多返回 Constants.maxResults 条
156. */
157. public List find(final String hql, final Map map) {
158. final int firstResult = 0;
159. return find(hql, map, firstResult, Constants.MAXRESULTS);
160. }
161.
162. /**
163. * 通过Hql 执行update/delete操作
164. *
165. * @param hql
166. * @return
167. */
168. public int executeUpdate(final String hql) {
169. int result = 0;
170. Object o = getHibernateTemplate().execute(new HibernateCallback() {
171. public Object doInHibernate(final Session session)
172. throws HibernateException {
173. int result = 0;
174. Query queryObject = session.createQuery(hql);
175.
176. result = queryObject.executeUpdate();
177. return result;
178.
179. }
180. });
181. Integer i = (Integer) o;
182. result = i.intValue();
183. return result;
184. }
185.
186. public void deleteAllByObject(Object obj) {
187. List l = null;
188. String hql = " from " + obj.getClass().getName() + " ";
189. if (log.isDebugEnabled()) {
190. log.debug("hql=" + hql);
191. }
192. l = find(hql);
193. Iterator ait = l.iterator();
194. while (ait != null && ait.hasNext()) {
195. getHibernateTemplate().delete(ait.next());
196. }
197. }
198.
199. /**
200. * 通过 DetachedCriteria 进行查询
201. *
202. * @param dc
203. * @return
204. */
205. public List findByCriteria(final DetachedCriteria dc) {
206. return (List) getHibernateTemplate().execute(new HibernateCallback() {
207. public Object doInHibernate(Session session)
208. throws HibernateException {
209. if (log.isDebugEnabled()) {
210. log.debug("dc=" + dc);
211. }
212. Criteria criteria = dc.getExecutableCriteria(session);
213. return criteria.list();
214. }
215. }, true);
216.
217. }
218.
219. /**
220. * 通过 DetachedCriteria 进行查询
221. *
222. * @param dc
223. * @return
224. */
225. public List findByCriteria(final DetachedCriteria dc,
226. final int firstResult, final int maxResults) {
227. return (List) getHibernateTemplate().execute(new HibernateCallback() {
228. public Object doInHibernate(final Session session)
229. throws HibernateException {
230. if (log.isDebugEnabled()) {
231. log.debug("findByCriteria dc=" + dc);
232. }
233. Criteria criteria = dc.getExecutableCriteria(session);
234. criteria.setFirstResult(firstResult);
235. criteria.setMaxResults(maxResults);
236. return criteria.list();
237. }
238. }, true);
239.
240. }
241.
242. public int countByCriteria(final DetachedCriteria dc) {
243. Integer count = (Integer) getHibernateTemplate().execute(
244. new HibernateCallback() {
245. public Object doInHibernate(Session session)
246. throws HibernateException {
247. if (log.isDebugEnabled()) {
248. log.debug("countByCriteria dc=" + dc);
249. }
250. Criteria criteria = dc.getExecutableCriteria(session);
251. return criteria.setProjection(Projections.rowCount())
252. .uniqueResult();
253. }
254. }, true);
255. if (count != null) {
256. return count.intValue();
257. } else {
258. return 0;
259. }
260. }
261.
262. /**
263. * 通过 DetachedCriteria 进行查询
264. *
265. * @param dc
266. * @return
267. */
268. public List findByCriteriaCache(final DetachedCriteria dc) {
269. return (List) getHibernateTemplate().execute(new HibernateCallback() {
270. public Object doInHibernate(Session session)
271. throws HibernateException {
272. if (log.isDebugEnabled()) {
273. log.debug("dc=" + dc);
274. }
275. Criteria criteria = dc.getExecutableCriteria(session);
276. criteria.setCacheable(true);
277. return criteria.list();
278. }
279. }, true);
280.
281. }
282.
283. /**
284. * 通过 DetachedCriteria 进行查询
285. *
286. * @param dc
287. * @return
288. */
289. public List findByCriteriaCache(final DetachedCriteria dc,
290. final int firstResult, final int maxResults) {
291. return (List) getHibernateTemplate().execute(new HibernateCallback() {
292. public Object doInHibernate(final Session session)
293. throws HibernateException {
294. if (log.isDebugEnabled()) {
295. log.debug("dc=" + dc);
296. }
297. Criteria criteria = dc.getExecutableCriteria(session);
298. criteria.setCacheable(true);
299. criteria.setFirstResult(firstResult);
300. criteria.setMaxResults(maxResults);
301. return criteria.list();
302. }
303. }, true);
304.
305. }
306.
307. /**
308. * count search cache
309. */
310. public int countByCriteriaCache(final DetachedCriteria dc) {
311. Integer count = (Integer) getHibernateTemplate().execute(
312. new HibernateCallback() {
313. public Object doInHibernate(Session session)
314. throws HibernateException {
315. if (log.isDebugEnabled()) {
316. log.debug("dc=" + dc);
317. }
318. Criteria criteria = dc.getExecutableCriteria(session);
319. criteria.setCacheable(true);
320. return criteria.setProjection(Projections.rowCount())
321. .uniqueResult();
322. }
323. }, true);
324. if (count != null) {
325. return count.intValue();
326. } else {
327. return 0;
328. }
329. }
330.
331. public int executeUpdate(final String hql, final Map pMap) {
332. int result = 0;
333. Object o = getHibernateTemplate().execute(new HibernateCallback() {
334. public Object doInHibernate(final Session session)
335. throws HibernateException {
336. int result = 0;
337. Query queryObject = session.createQuery(hql);
338. String[] params = queryObject.getNamedParameters();
339. for (int i = 0, max = params.length; i < max; i++) {
340. queryObject.setParameter(params[i], pMap.get(params[i]));
341. }
342. result = queryObject.executeUpdate();
343. return result;
344.
345. }
346. });
347. Integer i = (Integer) o;
348. result = i.intValue();
349. return result;
350. }
351.
352. public void delete(Object obj) {
353. getHibernateTemplate().delete(obj);
354. }
355.
356. public Object load(Class aclass, Serializable id)
357. throws DataAccessException {
358. Object obj = getHibernateTemplate().load(aclass, id);
359. return obj;
360. }
361.
362. public Object get(Class aclass, Serializable id) {
363. Object obj = getHibernateTemplate().get(aclass, id);
364. return obj;
365. }
366.
367. public void saveorUpdate(Object obj) {
368. getHibernateTemplate().saveOrUpdate(obj);
369. getHibernateTemplate().flush();
370. }
371.
372. public void update(Object o) {
373. getHibernateTemplate().update(o);
374. }
375.
376. /**
377. * count hql 方法 .
378. */
379. public int count(String hql, List ls) {
380. String countQueryString = " select count (*) " + hql;
381. List countlist = getHibernateTemplate().find(countQueryString);
382. Long count = (Long) countlist.get(0);
383. return count.intValue();
384. }
385.
386. /**
387. * count hql 方法 .
388. */
389. public int count(String hql, Map params) {
390. String countQueryString = " select count (*) " + hql;
391. List countlist = find(countQueryString, params);
392. Object co = countlist.get(0);
393. if (co instanceof Integer) {
394. Integer count = (Integer) countlist.get(0);
395. return count.intValue();
396. } else {
397. Long count = (Long) countlist.get(0);
398. return count.intValue();
399. }
400. }
401.
402. /**
403. * TODO count hql 方法 .
404. */
405. public int countCache(String hql, Map params) {
406. String countQueryString = " select count (*) " + hql;
407. List countlist = findCache(countQueryString, params);
408. Object co = countlist.get(0);
409. if (co instanceof Integer) {
410. Integer count = (Integer) countlist.get(0);
411. return count.intValue();
412. } else {
413. Long count = (Long) countlist.get(0);
414. return count.intValue();
415. }
416. }
417.
418. /**
419. * 根据查询语句,返回对象列表 TODO
420. *
421. * @param hql
422. * 查询语句
423. * @return 符合查询语句的对象列表;最多返回 Constants.maxResults 条
424. */
425. public List findCache(final String hql, final Map map) {
426. final int firstResult = 0;
427. return findCache(hql, map, firstResult, Constants.MAXRESULTS);
428. }
429.
430. /**
431. * 查询语句需要的条件参数。通过map传递 TODO
432. *
433. * @param hql
434. * 查询语句
435. * @param map
436. * 参数
437. * @param firstResult
438. * 起始位置
439. * @param maxResults
440. * 最多纪录数
441. * @return list 结果列表
442. */
443. public List findCache(final String hql, final Map map,
444. final int firstResult, final int maxResults) {
445. return getHibernateTemplate().executeFind(new HibernateCallback() {
446. public Object doInHibernate(final Session session)
447. throws HibernateException {
448. Query queryObject = session.createQuery(hql);
449. // 设置 查询cache
450. queryObject.setCacheable(true);
451. String[] params = queryObject.getNamedParameters();
452. for (int i = 0, max = params.length; i < max; i++) {
453. queryObject.setParameter(params[i], map.get(params[i]));
454. }
455. queryObject.setFirstResult(firstResult);
456. queryObject.setMaxResults(maxResults);
457. return queryObject.list();
458. }
459. });
460. }
461.
462. public int count(String hql) {
463. String countQueryString = " select count (*) " + hql;
464. List countlist = find(countQueryString);
465. Object co = countlist.get(0);
466. if (co instanceof Integer) {
467. Integer count = (Integer) countlist.get(0);
468. return count.intValue();
469. } else {
470. Long count = (Long) countlist.get(0);
471. return count.intValue();
472. }
473. }
474.
475. @Override
476. public HibernateTemplate getTemplate() {
477. return getHibernateTemplate();
478. }
转载通用dao
原创
©著作权归作者所有:来自51CTO博客作者mb6434c781b2176的原创作品,请联系作者获取转载授权,否则将追究法律责任
下一篇:对Java同步一些理解
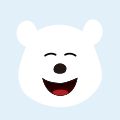
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
MyBatis通用dao和通用service
更新于2015/01/29,增加了第三种方法##更新于2015/02/09,第三种方法
mybatis ide spring java -
通用DAO设计(泛型方法)
import java.io.Serializable;
java ide List -
Mybatis通用DAO设计封装(mybatis)
关键字:Mybatis通用DAO设计封装(mybatis)
java 命名空间 SQL -
Dao层通用化,Spring3.0+Hibernate3.3.2通用Dao层整合
Dao层通用化,Spring3.0+Hibernate3.3.2通用Dao层整合
Dao层通用化 Spring3.0+Hibernate3