import smtplib, email
class SendEmail(object):
def __init__(self):
pass
def sendmail(self, mFrom, mTo, mSubject, mContent, ishtml = True, attachFile = None):
msg = MIMEMultipart()
msg['From'] = mFrom
msg['To'] = mTo
msg['Subject'] = mSubject
msgAlernative = MIMEMultipart('alernative')
msg.attach(msgAlernative)
if ishtml: #判断发送邮件的文本格式
#add html mail
html=mContent
msgHtml = MIMEText(html, 'html')
msgAlernative.attach(msgHtml)
else:
#add plain mail
txt = MIMEText(mContent)
msgAlernative.attach(txt)
#添加二进制附件
if attachFile:
fileName = attachFile
ctype, encoding = mimetypes.guess_type(fileName)
if ctype is None or encoding is not None:
ctype = 'application/octet-stream'
maintype, subtype = ctype.split('/', 1)
att1 = MIMEImage((lambda f: (f.read(), f.close())) \
(open(fileName, 'rb'))[0], _subtype = subtype)
att1.add_header('Content-Disposition', 'attachment', \
filename = path.basename(attachFile))
msg.attach(att1)
#发送邮件
smtp = smtplib.SMTP()
smtp.connect('smtp.server.com:25') ####需要修改邮件服务器
smtp.login('user', 'passwd') ###需要修改
smtp.sendmail(mFrom, mTo, msg.as_string())
smtp.quit()
return 'ok'
if __name__ == '__main__':
sm = SendEmail()
print sm.sendmail('mFrom', 'mTo','subject', 'html/plain content', 1/0, r'attach file path')
python邮件【mail】相关模块简单操作
原创
©著作权归作者所有:来自51CTO博客作者testqa_cn的原创作品,请联系作者获取转载授权,否则将追究法律责任
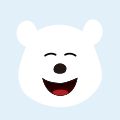
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章