/****************************************************************
*filename:myvm.c
*author:hemmingway
time:2012年05月25日 星期五 17时46分39秒
*compile with:gcc -g -Wall myvm.c -o myvm -lvirt
****************************************************************/#include <stdio.h>
#include <stdlib.h>
#include <libvirt/libvirt.h>#define MAXID 50
/*the data strucure of time*/
typedef struct _timeInfo
{
long long cpu_time;
struct timeval real_time;
}TIMEINFONODE;/*the hypervisor connection*/
static virConnectPtr conn = NULL;/*release the connecton of hypervisor*/
void closeConn()
{
if(conn != NULL)
virConnectClose(conn);
}/*release the domain pointer*/
void freeDom(virDomainPtr dom)
{
if(dom != NULL)
virDomainFree(dom);
}/*get start time of each domain*/
void getTimeInfo(int id,TIMEINFONODE *infos)
{
virDomainPtr dom = NULL;
virDomainInfo info;
int ret;
/*find the domain of the given id*/
dom = virDomainLookupByID(conn,id);
if(NULL == dom)
{
fprintf(stderr,"failed to find domain by id \'%d\'.\n",id);
freeDom(dom);
closeConn();
}
/*get the information of the domain*/
ret = virDomainGetInfo(dom,&info);
if(ret < 0) /*-1 ?*/
{
fprintf(stderr,"failed to get informaton for domain \'%d\'.\n",id);
freeDom(dom);
closeConn();
}
/*retrive the start time*/
if(gettimeofday(&(infos->real_time),NULL) == -1)
{
fprintf(stderr,"oh my god!failed to get start time.\n");
return;
}
/**/
infos->cpu_time = info.cpuTime;
freeDom(dom);
}void getDomainInfo(int id,TIMEINFONODE infos)
{
virDomainPtr dom = NULL;
virDomainInfo info;
int ret;
struct timeval realTime;
int cpu_diff,real_diff;
float usage;
/*find the domain of the given id*/
dom = virDomainLookupByID(conn,id);
if(NULL == dom)
{
fprintf(stderr,"failed to find domain by id \'%d\'.\n",id);
freeDom(dom);
closeConn();
}
/*get the information of the domain*/
ret = virDomainGetInfo(dom,&info);
if(ret < 0) /*-1 ?*/
{
fprintf(stderr,"failed to get informaton for domain \'%d\'.\n",id);
freeDom(dom);
closeConn();
}
/*retrive the start time*/
if(gettimeofday(&(infos.real_time),NULL) == -1)
{
fprintf(stderr,"oh my god!failed to get start time.\n");
return;
}
cpu_diff = (info.cpuTime - infos.cpu_time) / 10000;
real_diff = 1000 * (realTime.tv_sec - infos.real_time.tv_sec) +
(realTime.tv_usec - infos.real_time.tv_usec);
usage = cpu_diff / (float)(real_diff);
printf("%d\t%.3f\t%lu\t%lu\t%d\t%0x\t%s\n",
id,usage,info.memory/1024,info.maxMem/1024,
info.nrVirtCpu,info.state,virDomainGetName(dom)); freeDom(dom);
}int main()
{
int idCount;
int i;
int id;
int ids[MAXID];
char *host;
unsigned long ver;
virNodeInfo nodeinfo;
TIMEINFONODE timeInfos[MAXID];
printf("----------------------------------------\n");
printf(" xen domain monitor vrsion 0.1 \n");
printf(" build by somebody. \n");
printf("----------------------------------------\n");
/*connect to local xen hypervisor*/
conn = virConnectOpenReadOnly(NULL);
if(conn == NULL)
{
fprintf(stderr,"failed to connect to xen hypervisor\n");
closeConn();
return 0;
} host = virConnectGetHostname(conn);
printf("Hostname:%s\n",host); printf("Virtualization type:%s\n",virConnectGetType(conn));
virConnectGetVersion(conn, &ver);
printf("Version:%lu\n",ver);/*
virConnectGetLibVersion(conn, &ver);
printf("Libvirt Version:%lu\n",ver);
*/ virNodeGetInfo(conn, &nodeinfo);
printf("==========node info===============\n");
printf("Model:%s\n",nodeinfo.model);
printf("Memory:%lukb\n",nodeinfo.memory);
printf("Number of CPUs:%u\n",nodeinfo.cpus);
printf("MHz:%u\n",nodeinfo.mhz);
printf("Number of NUMA nodes:%u\n",nodeinfo.nodes);
printf("===============================\n"); idCount = virConnectListDomains(conn,&ids[0],MAXID);
if(idCount < 0)
{
fprintf(stderr,"failed to list domains\n");
closeConn();
return 0;
}
printf("Domain Totals: %d\n", idCount);
printf("ID\tCPU\tMEM\tMaxMEM\tVCPUs\tState\tNAME\n");
/* loop get the CPUtime info by IDs */
for (i = 0; i < idCount; i++)
{
id = ids[i];
getTimeInfo(id, &(timeInfos[i]));
}
sleep(1); //1 sec
/* loop print the domain info and calculate the usage of cpus*/
for (i = 0; i < idCount; i++)
{
id = ids[i];
getDomainInfo(id, timeInfos[i]);
}
printf("--------------------------------------------------------\n");
closeConn();
return 0;
}
test libvirt
原创
©著作权归作者所有:来自51CTO博客作者mb63982c735c3d9的原创作品,请联系作者获取转载授权,否则将追究法律责任
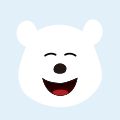
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
libvirt TLS
libvirt TLS
html 显式 访问者 -
libvirt监控其他