Java入门, 泛型编程
原创
©著作权归作者所有:来自51CTO博客作者mb63982c735c3d9的原创作品,请联系作者获取转载授权,否则将追究法律责任
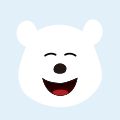
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
泛型入门
本章目标掌握泛型的产生意义掌握泛型的基本使用了解泛型的警告信息及泛型的擦除为什么要有泛型?现在有以下的实例要求: ...
java 泛型 System 泛型类型 -
Java泛型的入门
Java泛型的入门
jar Java java -
mysql rpm离线包下载
Linux平台上推荐使用RPM包来安装Mysql,MySQL 提供了以下RPM包的下载地址:MySQL-MySQL服务器。你需要该选项,除非你只想连接运行在另一台机器上的MySQL服务器。MySQL-client - MySQL 客户端程序,用于连接并操作Mysql服务器。MySQL-devel - 库和包含文件,如果你想要编译其它MySQL客户端,例如Perl模块,则需要安装该RPM包。MySQ
mysql rpm离线包下载 mysql mysql安装 mysql rpm包安装 linux安装mysql -
less 可以定义css变量吗
一、LESS概述:less是css的一种概述,在CSS的语法基础之上,它引入了变量,Mixin(混合),运算以及 函数等功能。大大的简
less 可以定义css变量吗 javascript ViewUI Less CSS