android-Intent电话、短信、联系人跳转
原创
©著作权归作者所有:来自51CTO博客作者徐的张的原创作品,请联系作者获取转载授权,否则将追究法律责任
首先看一下布局文件:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<EditText
android:id="@+id/edit"
android:hint="13057655618"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:onClick="dial"
android:text="Dial" />
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:onClick="call"
android:text="Call" />
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:onClick="add"
android:text="add" />
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:onClick="send"
android:text="send" />
</LinearLayout>
一个EditText用于记录输入,四个Button,分别设置了响应的onClick。
首先看一下第一个Button的逻辑处理如下:
public void dial(View view) {
phone = mEditText.getText().toString();
Intent intent = new Intent(Intent.ACTION_DIAL);
Uri data = Uri.parse("tel:" + phone);
intent.setData(data);
startActivity(intent);
}
初始化Intent时传入Intent.ACTION_DIAL(Intent隐式启动),也就是:
public static final String ACTION_DIAL = "android.intent.action.DIAL";
通过setData将电话传入,调用startActivity启动Intent。
运行实例如下:

public void call(View view) {
phone = mEditText.getText().toString();
Intent intent = new Intent(Intent.ACTION_CALL);
Uri data = Uri.parse("tel:" + phone);
intent.setData(data);
if (ActivityCompat.checkSelfPermission(this, Manifest.permission.CALL_PHONE)
!= PackageManager.PERMISSION_GRANTED) {
if (Build.VERSION.SDK_INT >= 23) {
ActivityCompat.requestPermissions(
this,new String[]{Manifest.permission.CALL_PHONE},123);
}
}else {
startActivity(intent);
}
}
@Override
public void onRequestPermissionsResult(int requestCode,
@NonNull String[] permissions, @NonNull int[] grantResults) {
super.onRequestPermissionsResult(requestCode, permissions, grantResults);
if(requestCode==123){
Intent intent = new Intent(Intent.ACTION_CALL);
Uri data = Uri.parse("tel:" + phone);
intent.setData(data);
startActivity(intent);
Log.d("Yayun", "onRequestPermissionsResult: ");
}
}
直接拨打电话,6.0以上需要运行时权限,首先判断是否有 Manifest.permission.CALL_PHONE 权限,如果有则直接调用startActivity启动Intent。如果没有则调用requestPermission方法请求权限。需要传入三个参数:上下文对象、权限、返回码。
onRequestPermissionsResult方法是回调方法,根据返回码判断是否是电话请求权限的返回,若是,则再次启动Intent。
运行实例如下:
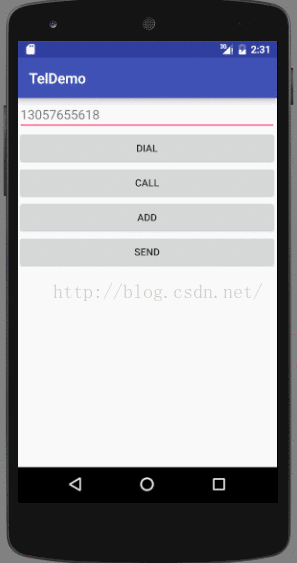
public void add(View view) {
phone = mEditText.getText().toString();
Intent it = new Intent(Intent.ACTION_INSERT, Uri.withAppendedPath(
Uri.parse("content://com.android.contacts"), "contacts"));
it.setType("vnd.android.cursor.dir/person");
it.putExtra(android.provider.ContactsContract.Intents.Insert.NAME, " ");
it.putExtra(android.provider.ContactsContract.Intents.Insert.PHONE, phone);
startActivity(it);
}
实例化时传入Intent.ACTION_INSERT值,设置type为vnd.android.cursor.dir/person,并设置NAME和PHONE的默认值。运行实例如下:

public void send(View view){
phone = mEditText.getText().toString();
String tel = "smsto:" + phone;
Uri uri = Uri.parse(tel);
Intent it = new Intent(Intent.ACTION_SENDTO);
it.setData(uri);
it.putExtra("sms_body", "The SMS text");
startActivity(it);
}
实例化时传入Intent.ACTION_SENDTO值 ,并设置key为sms_body的值为The SMS text,也就是短信内容。运行实例如下:

谢谢关注微信公众号,欢迎分享到朋友圈哦.
