#pragma once
class Cstring{
Cstring():m_pData(new char[1]){ *m_pData='\0';} //默认构造
Cstring(const char *pStr):m_pData(new char[strlen(pStr)+1]){ strcpy(m_pData,pStr); }
Cstring(const Cstring &str):m_pData(new char[str.size()+1]){strcpy(m_pData,str.m_pData);} //赋值构造
friend ostream& operator<<(ostream& os,Cstring& str);
friend istream& operator>>(istream& is,Cstring& str);
~Cstring(){ delete [] m_pData;} //析构
public:
size_t size() const
{
return strlen(m_pData);
}
Cstring & operator = ( const Cstring & other);
Cstring & operator = ( const char * pchar);
Cstring & operator + ( const Cstring & other);
Cstring & operator + ( const Cstring & other) const;
private:
char * m_pData;
};
inline Cstring& Cstring ::operator =( const Cstring & other){
if (this != &other)
{
delete [] m_pData;
m_pData = new char[other.size()+1];
strcpy(m_pData,other.m_pData);
}
return *this;
}
Cstring & Cstring::operator = ( const char * pchar)
{
if (m_pData != NULL)
{
delete [] m_pData;
}
size_t sz = strlen(pchar);
m_pData = new char[sz+1];
strcpy(m_pData,pchar);
return *this;
}
inline Cstring& Cstring::operator +(const Cstring & other) //改变被加数
{
char * temp = m_pData;
m_pData = new char[strlen(m_pData)+strlen(other.m_pData)+1];
strcpy(m_pData,temp);
delete []temp;
strcat(m_pData,other.m_pData);
return *this;
}
inline Cstring& Cstring::operator +(const Cstring & other) const //不改变被加数
{
Cstring strnew(); //Cstring* pstrnew = new Cstring();
delete [] strnew.m_pData;
strnew.m_pData = new char[strlen(m_pData)+strlen(other.m_pData)+1];
strcpy(strnew.m_pData,m_pData);
strcat(strnew.m_pData,other.m_pData);
return strnew;
}
ostream& Cstring::operator<<(ostream& os,Cstring& str)
{
os<< str.m_pData;
return os;
}
istream& Cstring::operator>>(istream& is,Cstring& str)
{
char arrchar[100];
is.getline(arrchar,100);
str = arrchar;
return is;
}
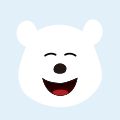
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
CString::Format
CString::Format// 看代码,针对format带参数列表的情况没整明白,于是开始百度,搜
cstring format ide Time 百度 -
CString基础
1、2、3、4、
CString bc 字符串 ico -
CString常用方法
CString::Compare int Compare( LPCTSTR lpsz ) const;返回值 字符串一样 返回0 小于lpsz 返回 -1 大于lpsz 返回1 区分大小字
insert delete windows null file -
CString源代码
CString使用的是引用技术,可以共享数据(这个大家都知道),另外空的CStirng是使用长度 int nAllocLength; //分配
null string insert delete struct -
SendMessage 传递 CString
1. CString作为参数传递MFC编程中,CString可以直接作为SendMessage()参数来传递。推荐用阻塞方式发送消息,这样能防
SendMessage CString char* ide 多字节