@Override
public List<SchoolTaskAllocation> findAll(HttpServletRequest request, HttpServletResponse response)
throws Exception {
List<SchoolTaskAllocation> schoolTaskAllocation =null;
List<String> KemuList = schoolTaskAllocationDao.selectKemu();
String serverPath = request.getSession().getServletContext().getRealPath("/") + "\\upload\\tempExcel"; // 设置下载excel的临时文件夹
File file = new File(serverPath);
List<File> srcFile = new ArrayList<>();
if (!file.exists()) {
file.mkdirs();
}
for (int k = 0; k < KemuList.size(); k++) {
XSSFWorkbook hwb = new XSSFWorkbook();// 第一步,创建一个workbook(一个excel文件)
XSSFSheet hs = hwb.createSheet("表格详情信息");// 第二步,在workbook中添加一个sheet,对应excel文件中sheet
XSSFRow hr = hs.createRow((int) 0);// 第三部,在sheet中添加表头第0行(相当于解释字段)
// 1.生成字体对象
XSSFFont font = hwb.createFont();
font.setFontName("仿宋_GB2312");
font.setBoldweight(XSSFFont.BOLDWEIGHT_BOLD);// 粗体显示
font.setFontHeightInPoints((short) 16);// 设置字体大小 XSSFCellStyle hcs = hwb.createCellStyle();// 第四步,设置第0行(表头)居中
hcs.setAlignment(XSSFCellStyle.ALIGN_CENTER);// 创建居中格式
hcs.setFont(font); XSSFCellStyle hcs2 = hwb.createCellStyle();// 第四步,设置第0行(表头)居中
hcs2.setAlignment(XSSFCellStyle.ALIGN_CENTER);// 创建居中格式
// 将表头的字段放入数组当中
String[] arr = new String[4];
arr[0] = "学校名称";
arr[1] = "题型";
arr[2] = "分配题目数";
for (int i = 0; i < arr.length; i++) {
XSSFCell hc = hr.createCell(i);
hc.setCellValue(arr[i]);
hc.setCellStyle(hcs);
hs.autoSizeColumn(i);
}
// excel插入表内容
schoolTaskAllocation = schoolTaskAllocationDao.selectAllocationExcelContents();
String subjectName = schoolTaskAllocation.get(0).getSubjectName();
List<SchoolTaskAllocation> addList = new ArrayList<>();
Map<String, List<SchoolTaskAllocation>> maps = new HashMap<String, List<SchoolTaskAllocation>>();
for (int i = 0; i < schoolTaskAllocation.size(); i++) {
if (!schoolTaskAllocation.get(i).getSubjectName().equals(subjectName)) {
maps.put(subjectName, addList);
subjectName = schoolTaskAllocation.get(i).getSubjectName();
addList = new ArrayList<>();
}
addList.add(schoolTaskAllocation.get(i));
if (i == schoolTaskAllocation.size() - 1) {
maps.put(subjectName, addList);
}
}
if (maps.containsKey(KemuList.get(k))) {
List<SchoolTaskAllocation> listF = maps.get(KemuList.get(k));
calculateExcel(hs, listF, hcs2);
String title = KemuList.get(k) + ".xlsx";
FileOutputStream fos = new FileOutputStream(serverPath + "\\" + title);// 先
// new
// 出文件存放的位置
hwb.write(fos);// 写入
fos.close();// 关闭资源
srcFile.add(new File(serverPath + "\\" + title));
}
}
// 将服务器上存放Excel的文件夹打成zip包
File zipfile = new File(serverPath + "\\" + "Excel" + ".zip");
zipFiles(srcFile, zipfile);// 实现将多个excel打包成zip文件
// 下载
downFile(response, serverPath, "Excel" + ".zip");
return schoolTaskAllocation;
} private void downFile(HttpServletResponse response, String serverPath, String str) {
try {
String path = serverPath + "\\" + str;
File file = new File(path);
if (file.exists()) {
InputStream ins = new FileInputStream(path);
BufferedInputStream bins = new BufferedInputStream(ins);// 放到缓冲流里面
OutputStream outs = response.getOutputStream();// 获取文件输出IO流
BufferedOutputStream bouts = new BufferedOutputStream(outs);
response.setContentType("application/ostet-stream");// 设置response内容的类型
response.setHeader("Content-disposition", "attachment;filename=" + URLEncoder.encode(str, "UTF-8"));// 设置头部信息
int bytesRead = 0;
byte[] buffer = new byte[8192];
// 开始向网络传输文件流
while ((bytesRead = bins.read(buffer, 0, 8192)) != -1) {
bouts.write(buffer, 0, bytesRead);
}
bouts.flush();// 这里一定要调用flush()方法
ins.close();
bins.close();
outs.close();
bouts.close();
} else {
response.sendRedirect("../error.jsp");
}
} catch (IOException e) {
e.printStackTrace();
} finally {
File file1 = new File(serverPath);
deleteExcelPath(file1); // 删除临时目录
}
} private boolean deleteExcelPath(File file) {
String[] files = null;
if (file != null) {
files = file.list();
} if (file.isDirectory()) {
for (int i = 0; i < files.length; i++) {
boolean bol = deleteExcelPath(new File(file, files[i]));
if (bol) {
System.out.println("删除成功!");
} else {
System.out.println("删除失败!");
}
}
}
return file.delete(); }
private void zipFiles(List<File> srcFile, File zipfile) {
byte[] buf = new byte[1024];
try {
// Create the ZIP file
ZipOutputStream out = new ZipOutputStream(new FileOutputStream(zipfile));
// Compress the files
for (int i = 0; i < srcFile.size(); i++) {
File file = srcFile.get(i);
FileInputStream in = new FileInputStream(file);
// Add ZIP entry to output stream.
out.putNextEntry(new ZipEntry(file.getName()));
// Transfer bytes from the file to the ZIP file
int len;
while ((len = in.read(buf)) > 0) {
out.write(buf, 0, len);
}
// Complete the entry
out.closeEntry();
in.close();
}
// Complete the ZIP file
out.close();
} catch (IOException e) {
e.printStackTrace();
}
下载打包多个excel表格上传到浏览器
原创
©著作权归作者所有:来自51CTO博客作者后端从入门到精通的原创作品,请联系作者获取转载授权,否则将追究法律责任
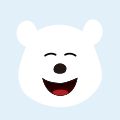
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
java怎么接入 百望云系统统一开票
相信只要报过税的小伙伴都知道,无论是属于季报的小规模纳税人还是个体工商户,只
java怎么接入 百望云系统统一开票 百旺如何看是否清卡 数据 服务器 表数据