from django.shortcuts import render
# Create your views here.
from rest_framework.response import Response
from rest_framework.views import APIView
import requests
import os
# Create your views here.
from PIL import Image, ImageWin
import json
import re
def download_image(url, directory):
# 确保目录存在
if not os.path.exists(directory):
os.makedirs(directory)
# 从URL下载图片
response = requests.get(url, stream=True)
response.raise_for_status()
filename = url.split("/")[-1]
# 生成一个临时文件名
file_path = os.path.join(directory,filename)
# 保存图片到文件
with open(file_path, 'wb') as file:
for chunk in response.iter_content(chunk_size=1024):
file.write(chunk)
return file_path
def delete_image(file_path):
# 删除图片文件
os.remove(file_path)
class printquestions(APIView):
def post(self, request, *args, **kwargs):
data = request.data.get("data")
data1 = json.loads(data)
# 定义字符映射表,将标点符号映射为空字符串
import win32print, win32ui, time
#from PIL import Image, ImageWin
printers = [printer[2] for printer in win32print.EnumPrinters(2)]
for i, printer in enumerate(printers):
print(f"{i + 1}: {printer}")
print(data1)
print(type(data1))
# 选择打印机
# choice = int(input("选择要使用的打印机 (输入对应的序号): ")) - 1
printer_name = '1' #printers[0]
hDC = win32ui.CreateDC()
hDC.CreatePrinterDC(printer_name) #
hDC.StartDoc("标签名")
hDC.StartPage()
Ctime = time.strftime("%H:%M", time.localtime())
Num = 'A201'
Name = '张三 男 66'
DataList = [
[190, 0, '用时:', {'name': '黑体', 'height': 30}],
[15, 0, '姓名:', {'name': '黑体', 'height': 30}],
# [15, 94, '项目:', {'name': '宋体', 'height': 27}],
# [90, 10, Num, {'name': '宋体', 'height': 33, 'weight': 1000}],
[340,0,"发卷时间:"+Ctime, {'name': '黑体', 'height': 30}],
# [462, 10, Name, {'name': '宋体', 'height': 33, 'weight': 1000}],
]
for data in DataList:
font = win32ui.CreateFont(data[3])
hDC.SelectObject(font)
hDC.TextOut(data[0], data[1], data[2])
#'http://haikou.91yuwen.com/lessonvideo/640.jpg'
font = win32ui.CreateFont({'name': '宋体', 'height': 22, })
hDC.SelectObject(font)
# 长文本换行
fsize = 40 # 字体大小
min_x =50 # X轴最小值
max_x = 560 # X轴最大值
text_x = 20 # 字X坐标
text_y = 60 # 字Y坐标
font = win32ui.CreateFont({'name': '宋体', 'height': fsize})
hDC.SelectObject(font)
print(data1)
# 用list(项目)转为列表,后用for加位置判断一个个字打入,实现换行
for x in data1:
if x is not None and x.startswith("http"):
print("字符串以'http'开头")
# 使用示例
url = x # 替换为你要下载的图片的URL
directory = "E:/代码库/print/printing/" # 替换为你要保存图片的目录
# 下载图片并获取文件路径
file_path = download_image(url, directory)
print(f"图片已下载到: {file_path}")
# 在这里,你可以对下载的图片进行任何操作,例如打开它、处理它等。
# # 加载图片
image = Image.open(file_path)
# 绘制位图
dib = ImageWin.Dib(image)
dib.draw(hDC.GetHandleOutput(), (text_x, text_y, 400 + text_x, 400 + text_y))
text_y += 400
# 删除图片文件
#delete_image(file_path)
#print("图片已删除。")
# # 加载图片
# image_path = 'E:/代码库/print/printing/微信截图_20231223104345.png'
# image = Image.open(image_path)
# print(text_x + 50, 300, 400, image.height + 300)
# # 绘制位图
# dib = ImageWin.Dib(image)
# dib.draw(hDC.GetHandleOutput(), (text_x, text_y, 400 + text_x, 400 + text_y))
# text_y += 400
else:
match = re.search(r'\([^()]*\)', x)
if match:
# 提取找到的部分
extracted = match.group()
print(f"提取的部分: {extracted}")
font = win32ui.CreateFont({'name': '黑体', 'height': 16})
hDC.SelectObject(font)
hDC.TextOut(text_x-20, text_y-5, extracted)#让序号保持在最边上
text_x += fsize + 3 # 字间距0
# 从原字符串中移除找到的部分
x = x.replace(extracted, '', 1)
print(f"移除后的字符串: {x}")
else:
print("没有找到带有括号的部分")
for text in list(x):
if text_x > max_x and text not in ['%']: # 判断 text_x是否超过最大设定值 及 是否'、'开头
text_y += fsize + 25 # 行间距3
text_x = min_x
print(text_y,'每行y轴')
font = win32ui.CreateFont({'name': '黑体', 'height': 35})
hDC.SelectObject(font)
hDC.TextOut(text_x, text_y, text)
text_x += fsize + 6 # 字间距0
text_x = 20
text_y += fsize + 60 # 行间距3
hDC.TextOut(text_x, text_y + 60, "豌豆语文 严选100 高效刷题营")
hDC.EndPage()
hDC.EndDoc()
return Response(200)
热敏打印 加入打印图片 记录下
精选 原创
©著作权归作者所有:来自51CTO博客作者小胖子就是我的原创作品,请联系作者获取转载授权,否则将追究法律责任
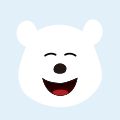
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
日志打印
日志打印
日志打印 Spring日志 日志框架选择与转换