import java.io.IOException;
import java.io.InputStreamReader;
import java.io.BufferedReader;/**
* Class SortedLink Description 有序链表,首先的搜索链表,直到找到合适的位置,它恰好在第一个比它大的数据项的前面,
* 主要是插入是必须使整个链表有序。 Company OpenData Author Chenlly Date 09-03-05 Version 1.0
*/class Link {
public Long lData;
public Link next; // 构造方法
public Link(Long lData) {
this.lData = lData;
} // 显示链表信息
public void displayLink() {
System.out.println("" + lData + ",");
}
}public class SortedLink {
private Link firstLink;
private int size; public SortedLink() {
// to do something
firstLink = null;
} // 构造一个新节点
public Link createNode(Long lData) {
Link link = new Link(lData);
return link;
} // 插入一个新节点使整个链表有序
public void insert(Link link) {
Link current = firstLink;
Link previous = null;
//链表从头到尾,按升序排列
while (current != null && current.lData < link.lData) {
previous = current;
current = current.next;
}
if (previous == null) {
firstLink=link;//第一次插入的情况
} else {
link.next = current;
previous.next = link;
}
} // 删除一个最小的节点
public Link remove() throws Exception {
if (firstLink==null){
throw new Exception ("WARN - 链表中还没有数据项,不能做删除操作");
}
Link temp = firstLink;
firstLink = firstLink.next;
return temp;
} // 显示链表信息
public void display() {
System.out.println("from first-->end:");
Link current = firstLink;
while (current != null) {
current.displayLink();
current = current.next;
}
} // 从键盘输入数据
public static String readStr() {
InputStreamReader ir = new InputStreamReader(System.in);
BufferedReader br = new BufferedReader(ir);
String str = "";
try {
str = br.readLine();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return str;
} public static void main(String[] args) {
System.out.println("链表基本算法请选择:");
System.out.println("1 插入新节点");
System.out.println("2 删除新节点");
System.out.println("3 显示链表信息");
System.out.println("4 退出");
SortedLink sortedLink = new SortedLink();
while (true) {
System.out.println("请输入操作序列:");
int op = Integer.parseInt(SortedLink.readStr());
switch (op) {
case 1:
System.out.println("请输入节点信息");
Long lData = new Long(Long.parseLong(SortedLink.readStr()));
Link newLink = sortedLink.createNode(lData);
sortedLink.insert(newLink);
break;
case 2:
Link oldLink = null;
try {
oldLink = sortedLink.remove();
} catch (Exception ex){
ex.printStackTrace();
}
if (oldLink != null) {
System.out.println("删除的节点信息:" + oldLink.lData);
} else {
System.out.println("删除节点失败");
}
break;
case 3:
sortedLink.display();
break;
case 4:
default:
System.exit(0);
}// end switch
}// end while
}
}
链表-有序链表操作
原创
©著作权归作者所有:来自51CTO博客作者wx63086371c7e9c的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:哈希表-开发地址法
下一篇:链表-基本算法(增、删)
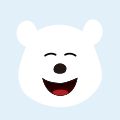
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
【数据结构】链式家族的成员——循环链表与静态链表
【数据结构】第二章——线性表(8)详细介绍了循环链表与静态链表的相关内容……
数据结构 C语言 循环链表 静态链表 -
合并两个有序链表【链表】
时间复杂度:空间复杂度:
链表 python 数据结构 空间复杂度 时间复杂度 -
数据结构面试之二——双向链表表、循环链表、有序链表的常见操作
数据结构面试之二——双向链表表、循环链表、有序链表的常见操作题注:《面试宝典》有相关习题,但思路相对不清晰,排版有错误
数据结构 面试 null delete list -
【LeetCode】有序链表合并
【LeetCode】经典题目之合并有序链表
leetcode 链表 头结点 python