柱状图
package util;
import java.awt.Color;
import java.awt.Font;
import java.io.FileOutputStream;
import java.io.IOException;
import org.jfree.chart.ChartFactory;
import org.jfree.chart.ChartUtilities;
import org.jfree.chart.JFreeChart;
import org.jfree.chart.axis.CategoryAxis;
import org.jfree.chart.axis.CategoryLabelPositions;
import org.jfree.chart.axis.NumberAxis;
import org.jfree.chart.axis.ValueAxis;
import org.jfree.chart.labels.StandardCategoryItemLabelGenerator;
import org.jfree.chart.plot.CategoryPlot;
import org.jfree.chart.plot.PlotOrientation;
import org.jfree.chart.renderer.category.BarRenderer;
import org.jfree.chart.title.LegendTitle;
import org.jfree.chart.title.TextTitle;
import org.jfree.data.category.CategoryDataset;
import org.jfree.data.category.DefaultCategoryDataset;
public class BarChart3DDemo
{
public static void main(String[] args) throws IOException
{
JFreeChart chart = ChartFactory.createBarChart3D(
"图书销量统计图", // 图表标题
"图书", // 目录轴的显示标签
"销量", // 数值轴的显示标签
getDataSet(), // 数据集
//PlotOrientation.HORIZONTAL , // 图表方向:水平
PlotOrientation.VERTICAL , // 图表方向:垂直
true, // 是否显示图例(对于简单的柱状图必须是false)
false, // 是否生成工具
false // 是否生成URL链接
);
//重新设置图标标题,改变字体
chart.setTitle(new TextTitle("图书销量统计图", new Font("黑体", Font.ITALIC , 22)));
//取得统计图标的第一个图例
LegendTitle legend = chart.getLegend(0);
//修改图例的字体
legend.setItemFont(new Font("宋体", Font.BOLD, 14));
CategoryPlot plot = (CategoryPlot)chart.getPlot(); //设置柱状图到图片上端的距离
ValueAxis rangeAxis = plot.getRangeAxis();
rangeAxis.setUpperMargin(0.1);
//取得横轴
CategoryAxis categoryAxis = plot.getDomainAxis();
//设置横轴显示标签的字体
categoryAxis.setLabelFont(new Font("宋体" , Font.BOLD , 22));
//分类标签以45度角倾斜
categoryAxis.setCategoryLabelPositions(CategoryLabelPositions.UP_45);
categoryAxis.setTickLabelFont(new Font("宋体" , Font.BOLD , 18));
//取得纵轴
NumberAxis numberAxis = (NumberAxis)plot.getRangeAxis();
//设置纵轴显示标签的字体
numberAxis.setLabelFont(new Font("宋体" , Font.BOLD , 22)); //在柱体的上面显示数据
BarRenderer custombarrenderer3d = new BarRenderer();
custombarrenderer3d.setBaseItemLabelPaint(Color.BLACK);//数据字体的颜色
custombarrenderer3d
.setBaseItemLabelGenerator(new StandardCategoryItemLabelGenerator());
custombarrenderer3d.setBaseItemLabelsVisible(true);
plot.setRenderer(custombarrenderer3d); FileOutputStream fos = null;
fos = new FileOutputStream("d:book1.jpg");
//将统计图标输出成JPG文件
ChartUtilities.writeChartAsJPEG(
fos, //输出到哪个输出流
1, //JPEG图片的质量,0~1之间
chart, //统计图标对象
800, //宽
600,//宽
null //ChartRenderingInfo 信息
);
fos.close();
}
//返回一个CategoryDataset实例
private static CategoryDataset getDataSet()
{
DefaultCategoryDataset dataset = new DefaultCategoryDataset();
dataset.addValue(45000 , "北京" , "Spring2.0宝典");
dataset.addValue(38000 , "北京" , "轻量级J2EE企业实战");
dataset.addValue(24000 , "北京" , "基于J2EE的Ajax宝典");
dataset.addValue(32000 , "北京" , "JavaScript权威指南");
dataset.addValue(21000 , "北京" , "Ajax In Action");
dataset.addValue(37000 , "上海" , "Spring2.0宝典");
dataset.addValue(36000 , "上海" , "轻量级J2EE企业实战");
dataset.addValue(34000 , "上海" , "基于J2EE的Ajax宝典");
dataset.addValue(42000 , "上海" , "JavaScript权威指南");
dataset.addValue(12000 , "上海" , "Ajax In Action");
dataset.addValue(42000 , "广州" , "Spring2.0宝典");
dataset.addValue(40000 , "广州" , "轻量级J2EE企业实战");
dataset.addValue(34000 , "广州" , "基于J2EE的Ajax宝典");
dataset.addValue(18000 , "广州" , "JavaScript权威指南");
dataset.addValue(26000 , "广州" , "Ajax In Action");
return dataset;
}
}饼状图
package test;
import java.awt.Color;
import java.awt.Font;
import java.io.File;
import java.io.IOException;
import java.text.DecimalFormat;
import java.text.NumberFormat;
import org.jfree.chart.ChartFactory;
import org.jfree.chart.ChartUtilities;
import org.jfree.chart.JFreeChart;
import org.jfree.chart.labels.StandardPieSectionLabelGenerator;
import org.jfree.chart.plot.PiePlot3D;
import org.jfree.chart.title.LegendTitle;
import org.jfree.chart.title.TextTitle;
import org.jfree.data.general.DefaultPieDataset;
public class testPieChart {
public static void main(String []args){
DefaultPieDataset dataset = new DefaultPieDataset();
dataset.setValue(" 市场前期", new Double(10));
dataset.setValue(" 立项", new Double(15));
dataset.setValue(" 计划", new Double(10));
dataset.setValue(" 需求与设计", new Double(10));
dataset.setValue(" 执行控制", new Double(35));
dataset.setValue(" 收尾", new Double(10));
dataset.setValue(" 运维",new Double(10));
JFreeChart chart = ChartFactory.createPieChart3D("项目状态分布", dataset,
true, true, false);
PiePlot3D plot = (PiePlot3D) chart.getPlot();
// 图片中显示百分比:默认方式
// plot.setLabelGenerator(new
// StandardPieSectionLabelGenerator(StandardPieToolTipGenerator.DEFAULT_TOOLTIP_FORMAT));
// 图片中显示百分比:自定义方式,{0} 表示选项, {1} 表示数值, {2} 表示所占比例 ,小数点后两位
plot.setLabelGenerator(new StandardPieSectionLabelGenerator(
"{0}({2})", NumberFormat.getNumberInstance(),
new DecimalFormat("0.00%")));
// 图例显示百分比:自定义方式, {0} 表示选项, {1} 表示数值, {2} 表示所占比例
plot.setLegendLabelGenerator(new StandardPieSectionLabelGenerator(
"{0}={1}({2})"));
// 设置背景色为白色
chart.setBackgroundPaint(Color.white);
// 指定图片的透明度(0.0-1.0)
plot.setForegroundAlpha(1.0f);
// 指定显示的饼图上圆形(false)还椭圆形(true)
plot.setCircular(true);
// 设置图标题的字体
Font font = new Font(" 黑体", Font.CENTER_BASELINE, 20);
TextTitle title = new TextTitle("项目状态分布");
title.setFont(font);
chart.setTitle(title);
plot.setLabelFont(new Font("SimSun", 0, 12));//
LegendTitle legend = chart.getLegend(0);
legend.setItemFont(new Font("宋体", Font.BOLD, 14)); try {
ChartUtilities.saveChartAsJPEG(
new File("d:\\PieTest9.jpg"), //输出到哪个输出流
1, //JPEG图片的质量,0~1之间
chart, //统计图标对象
640, //宽
300,//宽
null //ChartRenderingInfo 信息
);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}}
折线图
package chart;
import java.awt.Font;
import javax.swing.JPanel;
import org.jfree.chart.ChartFactory;
import org.jfree.chart.ChartPanel;
import org.jfree.chart.JFreeChart;
import org.jfree.chart.axis.CategoryAxis;
import org.jfree.chart.axis.NumberAxis;
import org.jfree.chart.plot.CategoryPlot;
import org.jfree.chart.plot.PlotOrientation;
import org.jfree.chart.title.LegendTitle;
import org.jfree.data.category.DefaultCategoryDataset;
import org.jfree.ui.ApplicationFrame;
import org.jfree.ui.RefineryUtilities;
public class LineCharts extends ApplicationFrame {
public LineCharts(String s) {
super(s);
setContentPane(createDemoLine());
}
public static void main(String[] args) {
LineCharts fjc = new LineCharts("折线图");
fjc.pack();
RefineryUtilities.centerFrameOnScreen(fjc);
fjc.setVisible(true);
}
// 生成显示图表的面板
public static JPanel createDemoLine() {
JFreeChart jfreechart = createChart(createDataset());
return new ChartPanel(jfreechart);
}
// 生成图表主对象JFreeChart
public static JFreeChart createChart(DefaultCategoryDataset linedataset) {
// 定义图表对象
JFreeChart chart = ChartFactory.createLineChart("折线图", // chart title
"时间", // domain axis label
"销售额(百万)", // range axis label
linedataset, // data
PlotOrientation.VERTICAL, // orientation
true, // include legend
true, // tooltips
false // urls
);
CategoryPlot plot = chart.getCategoryPlot();
// 设置图示字体
chart.getTitle().setFont(new Font("宋体", Font.BOLD, 22));
//设置横轴的字体
CategoryAxis categoryAxis = plot.getDomainAxis();
categoryAxis.setLabelFont(new Font("宋体", Font.BOLD, 22));//x轴标题字体
categoryAxis.setTickLabelFont(new Font("宋体", Font.BOLD, 18));//x轴刻度字体 //以下两行 设置图例的字体
LegendTitle legend = chart.getLegend(0);
legend.setItemFont(new Font("宋体", Font.BOLD, 14));
//设置竖轴的字体
NumberAxis rangeAxis = (NumberAxis) plot.getRangeAxis();
rangeAxis.setLabelFont(new Font("宋体" , Font.BOLD , 22)); //设置数轴的字体
rangeAxis.setTickLabelFont(new Font("宋体" , Font.BOLD , 22));
rangeAxis.setStandardTickUnits(NumberAxis.createIntegerTickUnits());//去掉竖轴字体显示不全
rangeAxis.setAutoRangeIncludesZero(true);
rangeAxis.setUpperMargin(0.20);
rangeAxis.setLabelAngle(Math.PI / 2.0);
return chart;
}// 生成数据
public static DefaultCategoryDataset createDataset() {
DefaultCategoryDataset linedataset = new DefaultCategoryDataset();
// 各曲线名称
String series1 = "冰箱";
String series2 = "彩电";
String series3 = "洗衣机";
// 横轴名称(列名称)
String type1 = "1月";
String type2 = "2月";
String type3 = "3月";
linedataset.addValue(0.0, series1, type1);
linedataset.addValue(4.2, series1, type2);
linedataset.addValue(3.9, series1, type3);
linedataset.addValue(1.0, series2, type1);
linedataset.addValue(5.2, series2, type2);
linedataset.addValue(7.9, series2, type3);
linedataset.addValue(2.0, series3, type1);
linedataset.addValue(9.2, series3, type2);
linedataset.addValue(8.9, series3, type3);
return linedataset;
}}
JFreeChart生成柱状图、饼状图、折线图详解
原创wx63046db09e68a 博主文章分类:JFreeChart ©著作权
©著作权归作者所有:来自51CTO博客作者wx63046db09e68a的原创作品,请联系作者获取转载授权,否则将追究法律责任
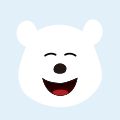
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
R语言基于Excel数据绘制条形图、柱状图的方法
本文介绍基于R语言中的readxl包与ggplot2包,读取Excel表格文件数据,并绘制具有多个系列的柱状图、条形图的方法~
R语言 表格数据 直方图 柱状图 数据可视化 -
Python绘制多时相栅格图像的像素数值折线图
本文介绍基于Python中的gdal模块,对大量长时间序列的栅格遥感影像文件,绘制其每一个波段中、若干随机指定的像元的时间序列曲线图的方法~
Python GDAL 栅格文件 像素数值 遥感影像 -
JFreeChart折线图,饼图,柱状图
java 柱状图 折线图 饼状图
java 柱状图 折线图 饼状图 -
ECharts之柱状图 饼状图 折线图
ECharts之柱状图 饼状图 折线图
javascript ECharts 柱状图 饼状图 折线图 -
echart折线图,柱状图,饼图设置颜色
之前在做报表的时候用过echart 用完也就完了,而这次在用的时候已经忘了
chart javascript 饼图 f5 -
柱状图,折线图,饼图的js插件
http://www.highcharts.com/
clementine -
[echarts] 饼图折线图柱状图相互转换
【代码】[echarts] 饼图折线图柱状图相互转换。
echarts 前端 javascript 数据转换 柱状图