import com.test.action.monitor.service.CommonService;
import org.apache.poi.hssf.usermodel.HSSFFont;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import org.apache.poi.ss.usermodel.CellStyle;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.ss.util.CellRangeAddress;
import org.apache.struts2.ServletActionContext;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.util.StringUtils;
import javax.mail.internet.MimeUtility;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.FileInputStream;
import java.io.OutputStream;
import java.net.URLEncoder;
import java.util.*;
import java.util.List;
public class exportTest {
/* iText是一个开源的PDF、Word工具,小巧而又便捷。
官方网站是:http://www.lowagie.com/iText/
官方的解释:iText is a library that allows you to generate PDF files . the fly
最新版本为:2.1.7
下载地址是:http://nchc.dl.sourceforge.net/sourceforge/itext/iText-2.1.7.jar
*/
protected HttpServletRequest getRequest() {
return ServletActionContext.getRequest();
}
protected HttpServletResponse getResponse() {
return ServletActionContext.getResponse();
}
public HttpServletRequest request;
public FileInputStream inputStream;
@Autowired
private CommonService commonService;
/**
* 综合报表统计导出Excel
*
* @author QC
*/
public String exportPDF() {
try {
String exportname = "测试报表";
String webTempDir = "/test/app/config";
String filePath = webTempDir + UUID.randomUUID().toString().replace("-", "") + ".pdf";
Workbook wb = new HSSFWorkbook();
Sheet sheet = wb.createSheet(exportname);
org.apache.poi.ss.usermodel.Row titleRow1 = sheet.createRow(0);
for (int columnIndex = 0; columnIndex < 8; columnIndex++) {
org.apache.poi.ss.usermodel.Cell cell = titleRow1.createCell(columnIndex);
if (columnIndex == 0) sheet.setColumnWidth(columnIndex, 30 * 200);
else if (columnIndex == 1) sheet.setColumnWidth(columnIndex, 30 * 250);
else if (columnIndex == 3) sheet.setColumnWidth(columnIndex, 30 * 200);
else if (columnIndex == 6) sheet.setColumnWidth(columnIndex, 30 * 200);
else sheet.setColumnWidth(columnIndex, 30 * 100);
}
// 设置字体样式 "黑体 "
HSSFFont font = (HSSFFont) wb.createFont();
font.setFontName("宋体");
font.setFontHeightInPoints((short) 10);// 设置字体大小
font.setBoldweight(HSSFFont.BOLDWEIGHT_BOLD);// 粗体显示
HSSFFont fontTitle = (HSSFFont) wb.createFont();
fontTitle.setFontName("宋体");
fontTitle.setFontHeightInPoints((short) 16);// 设置字体大小
fontTitle.setBoldweight(HSSFFont.BOLDWEIGHT_BOLD);// 粗体显示
HSSFFont fontTitle2 = (HSSFFont) wb.createFont();
fontTitle2.setFontName("宋体");
fontTitle2.setFontHeightInPoints((short) 10);// 设置字体大小
fontTitle2.setBoldweight(HSSFFont.BOLDWEIGHT_BOLD);// 粗体显示
HSSFFont fontCommit = (HSSFFont) wb.createFont();
fontCommit.setFontName("宋体");
fontCommit.setFontHeightInPoints((short) 12);// 设置字体大小
fontCommit.setBoldweight(HSSFFont.BOLDWEIGHT_BOLD);// 粗体显示
//填表说明内容的样式
HSSFFont fontCommitContext = (HSSFFont) wb.createFont();
fontCommitContext.setFontName("宋体");
fontCommitContext.setFontHeightInPoints((short) 8);// 设置字体大小
CellStyle centerStyle = wb.createCellStyle();
centerStyle.setAlignment(CellStyle.ALIGN_CENTER);
centerStyle.setVerticalAlignment(CellStyle.VERTICAL_CENTER);
centerStyle.setBorderBottom(CellStyle.BORDER_THIN);
centerStyle.setBorderTop(CellStyle.BORDER_THIN);
centerStyle.setBorderLeft(CellStyle.BORDER_THIN);
centerStyle.setBorderRight(CellStyle.BORDER_THIN);
centerStyle.setWrapText(true);
CellStyle reportTitleStyle = wb.createCellStyle();
reportTitleStyle.setAlignment(CellStyle.ALIGN_CENTER);
reportTitleStyle.setVerticalAlignment(CellStyle.VERTICAL_CENTER);
reportTitleStyle.setBorderBottom(CellStyle.BORDER_THIN);
reportTitleStyle.setBorderTop(CellStyle.BORDER_THIN);
reportTitleStyle.setBorderLeft(CellStyle.BORDER_THIN);
reportTitleStyle.setBorderRight(CellStyle.BORDER_THIN);
reportTitleStyle.setFont(fontTitle);
CellStyle headerStyleTitle = wb.createCellStyle();
headerStyleTitle.setAlignment(CellStyle.ALIGN_CENTER);
headerStyleTitle.setVerticalAlignment(CellStyle.VERTICAL_CENTER);
headerStyleTitle.setBorderBottom(CellStyle.BORDER_THIN);
headerStyleTitle.setBorderTop(CellStyle.BORDER_THIN);
headerStyleTitle.setBorderLeft(CellStyle.BORDER_THIN);
headerStyleTitle.setBorderRight(CellStyle.BORDER_THIN);
headerStyleTitle.setFont(fontTitle2);
CellStyle headerStyle = wb.createCellStyle();
headerStyle.setAlignment(CellStyle.ALIGN_CENTER);
headerStyle.setVerticalAlignment(CellStyle.VERTICAL_CENTER);
headerStyle.setBorderBottom(CellStyle.BORDER_THIN);
headerStyle.setBorderTop(CellStyle.BORDER_THIN);
headerStyle.setBorderLeft(CellStyle.BORDER_THIN);
headerStyle.setBorderRight(CellStyle.BORDER_THIN);
headerStyle.setFont(font);
CellStyle centerStyle2 = wb.createCellStyle();
centerStyle2.setAlignment(CellStyle.ALIGN_CENTER);
centerStyle2.setVerticalAlignment(CellStyle.VERTICAL_CENTER);
//填表说明的样式
CellStyle commitStyleTitle = wb.createCellStyle();
commitStyleTitle.setAlignment(CellStyle.ALIGN_LEFT);
commitStyleTitle.setVerticalAlignment(CellStyle.VERTICAL_CENTER);
commitStyleTitle.setFont(fontCommit);
//填表说明内容的样式
CellStyle commitContextStyleTitle = wb.createCellStyle();
commitContextStyleTitle.setAlignment(CellStyle.ALIGN_LEFT);
commitContextStyleTitle.setVerticalAlignment(CellStyle.VERTICAL_CENTER);
commitContextStyleTitle.setFont(fontCommitContext);
//表头
sheet.addMergedRegion(new CellRangeAddress(0, 0, 0, 9));
org.apache.poi.ss.usermodel.Row rwT = sheet.createRow(0);
org.apache.poi.ss.usermodel.Cell cellT = rwT.createCell(0);
cellT.setCellValue(exportname);
cellT.setCellStyle(reportTitleStyle);
rwT.createCell(1).setCellStyle(reportTitleStyle);
rwT.createCell(2).setCellStyle(reportTitleStyle);
rwT.createCell(3).setCellStyle(reportTitleStyle);
rwT.createCell(4).setCellStyle(reportTitleStyle);
rwT.createCell(5).setCellStyle(reportTitleStyle);
rwT.createCell(6).setCellStyle(reportTitleStyle);
rwT.createCell(7).setCellStyle(reportTitleStyle);
rwT.createCell(8).setCellStyle(reportTitleStyle);
rwT.createCell(9).setCellStyle(reportTitleStyle);
//填表说明
int commitBeginRow = 2;//开始行结束行索引
int commitFirstCol = 0;//第一列索引号
int commitLastCol = 9;//最后一列索引号
sheet.addMergedRegion(new CellRangeAddress(commitBeginRow, commitBeginRow, commitFirstCol, commitLastCol));//(开始行号,结束行号,开始列号,结束列
org.apache.poi.ss.usermodel.Row rwCommit = sheet.createRow(commitBeginRow);
org.apache.poi.ss.usermodel.Cell cellCommit = rwCommit.createCell(0);
cellCommit.setCellValue("一、测试");
cellCommit.setCellStyle(commitStyleTitle);
//填表说明
int serverbeginrow = 10;
commitBeginRow = serverbeginrow-1;
sheet.addMergedRegion(new CellRangeAddress(commitBeginRow, commitBeginRow, commitFirstCol, commitLastCol));//(开始行号,结束行号,开始列号,结束列号)
rwCommit = sheet.createRow(commitBeginRow);//创建行,commitbeainrow表示坐在列
cellCommit = rwCommit.createCell(0);
cellCommit.setCellValue("二、服务器运行指标");
cellCommit.setCellStyle(commitStyleTitle);
//服务器统计Demo-------------------------------------------------------------------------------------------------------------------------
//服务器报表标题
sheet.addMergedRegion(new CellRangeAddress(serverbeginrow, serverbeginrow, 0, 9));
org.apache.poi.ss.usermodel.Row rwTS = sheet.createRow(serverbeginrow);
org.apache.poi.ss.usermodel.Cell cellTS = rwTS.createCell(0);
cellTS.setCellValue("服务器");
cellTS.setCellStyle(headerStyleTitle);
rwTS.createCell(1).setCellStyle(headerStyleTitle);
rwTS.createCell(2).setCellStyle(headerStyleTitle);
rwTS.createCell(3).setCellStyle(headerStyleTitle);
rwTS.createCell(4).setCellStyle(headerStyleTitle);
rwTS.createCell(5).setCellStyle(headerStyleTitle);
rwTS.createCell(6).setCellStyle(headerStyleTitle);
rwTS.createCell(7).setCellStyle(headerStyleTitle);
rwTS.createCell(8).setCellStyle(headerStyleTitle);
rwTS.createCell(9).setCellStyle(headerStyleTitle);
sheet.addMergedRegion(new CellRangeAddress(serverbeginrow + 1, serverbeginrow + 2, 0, 1));
org.apache.poi.ss.usermodel.Row rw = sheet.createRow(serverbeginrow + 1);
org.apache.poi.ss.usermodel.Cell cell0 = rw.createCell(0);
cell0.setCellValue("名称");
cell0.setCellStyle(headerStyle);
rw.createCell(1).setCellStyle(headerStyle);
sheet.addMergedRegion(new CellRangeAddress(serverbeginrow + 1, serverbeginrow + 1, 2, 4));
org.apache.poi.ss.usermodel.Cell cell2 = rw.createCell(2);
cell2.setCellValue("CPU使用率");
cell2.setCellStyle(headerStyle);
sheet.addMergedRegion(new CellRangeAddress(serverbeginrow + 1, serverbeginrow + 1, 5, 7));
org.apache.poi.ss.usermodel.Cell cell3 = rw.createCell(5);
cell3.setCellValue("内存使用率");
cell3.setCellStyle(headerStyle);
sheet.addMergedRegion(new CellRangeAddress(serverbeginrow + 1, serverbeginrow + 1, 8, 9));
org.apache.poi.ss.usermodel.Cell cell4 = rw.createCell(8);
cell4.setCellValue("逻辑分区使用率");
cell4.setCellStyle(headerStyle);
rw.createCell(9).setCellStyle(headerStyle);
org.apache.poi.ss.usermodel.Row rw1 = sheet.createRow(serverbeginrow + 2);
rw1.createCell(0).setCellStyle(headerStyle);
rw1.createCell(1).setCellStyle(headerStyle);
org.apache.poi.ss.usermodel.Cell c2 = rw1.createCell(2);
c2.setCellValue("最高值");
c2.setCellStyle(headerStyle);
org.apache.poi.ss.usermodel.Cell c3 = rw1.createCell(3);
c3.setCellValue("最高值时间");
c3.setCellStyle(headerStyle);
org.apache.poi.ss.usermodel.Cell c4 = rw1.createCell(4);
c4.setCellValue("平均值");
c4.setCellStyle(headerStyle);
org.apache.poi.ss.usermodel.Cell c5 = rw1.createCell(5);
c5.setCellValue("最高值");
c5.setCellStyle(headerStyle);
org.apache.poi.ss.usermodel.Cell c6 = rw1.createCell(6);
c6.setCellValue("最高值时间");
c6.setCellStyle(headerStyle);
org.apache.poi.ss.usermodel.Cell c7 = rw1.createCell(7);
c7.setCellValue("平均值");
c7.setCellStyle(headerStyle);
org.apache.poi.ss.usermodel.Cell c8 = rw1.createCell(8);
c8.setCellValue("分区名称");
c8.setCellStyle(headerStyle);
org.apache.poi.ss.usermodel.Cell c9 = rw1.createCell(9);
c9.setCellValue("分区使用率");
c9.setCellStyle(headerStyle);
int beginrow = serverbeginrow + 3;
List<Map<String, Object>> list = this.getServerList();//得到数据
if (list.size() > 0) {
List<Map<String, Object>> mergedList = new ArrayList();
if (null != list && list.size() > 0) {
boolean b = false;
String paramname = "";
int begrow = 0;
int endrow = 0;
String param1 = "";
String NAME = "";
String MAXVAL = "";
String RTIME = "";
String AVGVAL = "";
String MAXMEMUSAGE = "";
String MEMTIME = "";
String AVGMEM = "";
String PARAM2 = "";
String PARAM4 = "";
for (int i = 0; i < list.size(); i++) {
Map<String, Object> temp = list.get(i);
org.apache.poi.ss.usermodel.Row row = sheet.createRow(i + beginrow);
param1 = temp.get("PARAM1") != null ? temp.get("PARAM1").toString() : "";
NAME = temp.get("NAME") != null ? temp.get("NAME").toString() : "";
MAXVAL = temp.get("MAXVAL") != null ? temp.get("MAXVAL").toString() : "";
RTIME = temp.get("RTIME") != null ? temp.get("RTIME").toString() : "";
AVGVAL = temp.get("AVGVAL") != null ? temp.get("AVGVAL").toString() : "";
MAXMEMUSAGE = temp.get("MAXMEMUSAGE") != null ? temp.get("MAXMEMUSAGE").toString() : "";
MEMTIME = temp.get("MEMTIME") != null ? temp.get("MEMTIME").toString() : "";
AVGMEM = temp.get("AVGMEM") != null ? temp.get("AVGMEM").toString() : "";
PARAM2 = temp.get("PARAM2") != null ? temp.get("PARAM2").toString() : "";
PARAM4 = temp.get("PARAM4") != null ? temp.get("PARAM4").toString() : "";
org.apache.poi.ss.usermodel.Cell ce0 = row.createCell(0);
ce0.setCellValue(param1);
ce0.setCellStyle(centerStyle2);
org.apache.poi.ss.usermodel.Cell ce1 = row.createCell(1);
ce1.setCellValue(NAME);
ce1.setCellStyle(centerStyle2);
org.apache.poi.ss.usermodel.Cell ce2 = row.createCell(2);
ce2.setCellStyle(centerStyle2);
ce2.setCellValue(MAXVAL);
org.apache.poi.ss.usermodel.Cell ce3 = row.createCell(3);
ce3.setCellStyle(centerStyle2);
ce3.setCellValue(RTIME);
org.apache.poi.ss.usermodel.Cell ce4 = row.createCell(4);
ce4.setCellStyle(centerStyle2);
ce4.setCellValue(AVGVAL);
org.apache.poi.ss.usermodel.Cell ce5 = row.createCell(5);
ce5.setCellStyle(centerStyle2);
ce5.setCellValue(MAXMEMUSAGE);
org.apache.poi.ss.usermodel.Cell ce6 = row.createCell(6);
ce6.setCellStyle(centerStyle2);
ce6.setCellValue(MEMTIME);
org.apache.poi.ss.usermodel.Cell ce7 = row.createCell(7);
ce7.setCellStyle(centerStyle2);
ce7.setCellValue(AVGMEM);
org.apache.poi.ss.usermodel.Cell ce8 = row.createCell(8);
ce8.setCellStyle(centerStyle2);
ce8.setCellValue(PARAM2);
org.apache.poi.ss.usermodel.Cell ce9 = row.createCell(9);
ce9.setCellStyle(centerStyle2);
ce9.setCellValue(PARAM4);
if (!paramname.equals(param1)) {
if (!paramname.equals(param1) && begrow < endrow) {
sheet.addMergedRegion(new CellRangeAddress(begrow, endrow, 0, 0));
}
paramname = param1;
begrow = i + beginrow;
endrow = begrow;
} else {
endrow++;
if ((i + 1) == list.size() && begrow < endrow) {
sheet.addMergedRegion(new CellRangeAddress(begrow, endrow, 0, 0));
}
}
}
}
}
//输入文件-------------------------------------------------------------------------------------------
String filename = exportname + ".xls";//设置下载时客户端Excel的名称
filename = encodeFilename(filename, request);//处理中文文件名
this.getResponse().setContentType("application/vnd.ms-excel");
this.getResponse().setHeader("Content-disposition", "attachment;filename=" + filename);
OutputStream os = this.getResponse().getOutputStream();
wb.write(os);
os.flush();
os.close();
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "redirectDownloadAction";
}
public List<Map<String, Object>> getServerList() throws Exception {
String sql = "select r.id setid,r.param1,r.param2,r.param3,r.param4,r.param5,r.field_name name,r.order_no,r.field_value devid from report r ";
List<Map<String, Object>> list = this.commonService.queryMapList(sql);
return list;
}
/**
* 设置下载文件中文件的名称
*
* @param filename
* @param request
* @return
* @author QC
*/
public static String encodeFilename(String filename, HttpServletRequest request) {
/**
* 获取客户端浏览器和操作系统信息
* 在IE浏览器中得到的是:User-Agent=Mozilla/4.0 (compatible; MSIE 6.0; Windows NT 5.1; SV1; Maxthon; Alexa Toolbar)
* 在Firefox中得到的是:User-Agent=Mozilla/5.0 (Windows; U; Windows NT 5.1; zh-CN; rv:1.7.10) Gecko/20050717 Firefox/1.0.6
*/
String agent = request.getHeader("USER-AGENT");
try {
if ((agent != null) && (-1 != agent.indexOf("MSIE"))) {
String newFileName = URLEncoder.encode(filename, "UTF-8");
newFileName = StringUtils.replace(newFileName, "+", "%20");
if (newFileName.length() > 150) {
newFileName = new String(filename.getBytes("GB2312"), "ISO8859-1");
newFileName = StringUtils.replace(newFileName, " ", "%20");
}
return newFileName;
}
if ((agent != null) && (-1 != agent.indexOf("Mozilla")))
return MimeUtility.encodeText(filename, "UTF-8", "B");
return filename;
} catch (Exception ex) {
return filename;
}
}
}
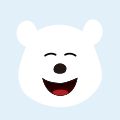
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
Java Excel导入导出
Java的两种方式操作Excel
数据 java excel 导出数据 -
使用poi导出excel
使用poi导出excel,其中涉及到居中、字体加粗、合并单元
java 导出excel List 下载文件 -
POI操作Excel文档(一)
一.POI简介 Jakarta POI 是apache的子项目,目标是处理ole2对象。它提供了一组操纵Windows文档的Java API 目前比较
excel 文档 spreadsheet file exception -
Java使用poi导入导出Excel java poi导出excel
相信在大部分的web项目中都会有导出导入Excel的需求,今天我们就来看看如何用Java代码去实现 用POI导出Excel表格。之前我也写过两篇导出Excel的文章,没看过的小伙伴可以去看哈,链接也给大家放出来了:1.导出多个sheet2.导出zip压缩包一、pom引用pom文件中,添加以下依赖<
Java使用poi导入导出Excel java 前端 spring boot spring cloud