interface Collection{...}
interface Set extends Collection{...}
interface NavigableMap{}
class TreeMap implements NavigableMap{
public V put(K key, V value) {
Entry<K,V> t = root;
if (t == null) {
// TBD:
// 5045147: (coll) Adding null to an empty TreeSet should
// throw NullPointerException
//
// compare(key, key); // type check
root = new Entry<K,V>(key, value, null);
size = 1;
modCount++;
return null;
}
int cmp;
Entry<K,V> parent;
// split comparator and comparable paths
Comparator<? super K> cpr = comparator;
if (cpr != null) {
do {
parent = t;
cmp = cpr.compare(key, t.key);
if (cmp < 0)
t = t.left;
else if (cmp > 0)
t = t.right;
else
return t.setValue(value);
} while (t != null);
}
else {
if (key == null)
throw new NullPointerException();
Comparable<? super K> k = (Comparable<? super K>) key;
do {
parent = t;
cmp = k.compareTo(t.key);
if (cmp < 0)
t = t.left;
else if (cmp > 0)
t = t.right;
else
return t.setValue(value);
} while (t != null);
}
Entry<K,V> e = new Entry<K,V>(key, value, parent);
if (cmp < 0)
parent.left = e;
else
parent.right = e;
fixAfterInsertion(e);
size++;
modCount++;
return null;
}
}
class TreeSet implements Set{
private transient NavigableMap<E,Object> m;
public TreeSet(){
this(new TreeMap<E,Object>());
}
public boolean add(E e) {
return m.put(e, PRESENT)==null;
}
}
真正比较的是依赖于元素的compareTo()方法,而这个方法是定义在Comparable里面的
所以,你想要重写该方法,就必须是先Comparable接口。这个接口表示的就是自然排序。
集合框架_TreeSet保证元素排序的源码解析
原创wx62dfdc6aea345 博主文章分类:Java ©著作权
©著作权归作者所有:来自51CTO博客作者wx62dfdc6aea345的原创作品,请联系作者获取转载授权,否则将追究法律责任
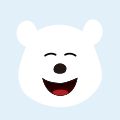
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
集合框架_TreeSet保证元素唯一性和比较器排序的原理及代码实现
package cn.itcast_07;
java 匿名内部类 比较器 -
java123-treeset排序集合
java
大数据 System java 排序方法 -
java集合框架保证元素插入顺序排序 java集合框架体系
java学习总结——集合框架 集合框架是为表示和操作集合而规定的一种统一的标准的体系结构。任何集合框架都包含三大块内容:对外的接口、接口的实现和对集合运算的算法。 (以上内容摘自百度百科) &nbs
java集合框架保证元素插入顺序排序 java 集合框架 List Set