#!/usr/bin/python
#encoding:utf-8
#version:1.1
import sys,os,commands;
def exec_test(cmd):
stat,output = commands.getstatusoutput(cmd);
if stat == 0:
return True;
else:
file = open('nginx_install_error.log','a');
file.write('%s \n' %output);
file.close();
return False;
#预安装环境升级
def install_gcc_make():
print '\033[32mstart install and upgrade environment...\033[0m';
cmd = 'yum -y install gcc automake autoconf libtool make gcc-c++ pcre* zlib* openssl openssl-devel';
if exec_test(cmd):
pass;
else:
print '\033[31minstall environment failed....\033[0m';
sys.exit();
def adduser():
add_cmd = 'useradd -s /sbin/nologin nginx';
if exec_test(add_cmd):
pass;
else:
print '\033[31madd user nginx failed...\033[0m';
sys.exit();
#下载nginx并安装
def download_nginx():
download_url = 'http://nginx.org/download/nginx-1.4.2.tar.gz';
download_cmd = 'wget %s' %(download_url);
if exec_test(download_cmd):
pass;
else:
print '\033[31mdownload nginx install packet failed...\033[0m';
sys.exit();
def install_nginx():
tar_cmd = 'tar -zxf nginx-1.4.2.tar.gz';
if exec_test(tar_cmd):
#chroot_cmd = 'cd nginx-1.4.2 && ';
os.chdir('./nginx-1.4.2');
config_cmd = './configure --user=nginx --group=nginx \
--with-http_stub_status_module \
--with-http_ssl_module \
--with-http_gzip_static_module \
--with-http_flv_module';
#install_cmd = chroot_cmd + config_cmd;
if exec_test(config_cmd):
os.system('make && make install');
#更改nginx目录的拥护者
os.system('chown -R nginx:nginx /usr/local/nginx');
os.system('cp -p /usr/local/nginx/sbin/nginx /usr/sbin/nginx');
os.system('ln -s /usr/local/nginx/conf/nginx.conf /etc/');
else:
print '\033[31mconfigure nginx failed..\033[0m';
sys.exit();
else:
print '\033[31m tar -zxf nginx-1.4.2.tar.gz failed...\033[0m';
sys.exit();
#添加nginx服务到系统并开机启动
def nginx_service():
download_cmd = 'wget -c ftp.sosolinux.com/nginx/nginx.sh';
if exec_test(download_cmd):
os.system('chmod u+x nginx.sh');
os.system('cp -p ./nginx.sh /etc/init.d/nginx');
#添加到开机启动
os.system('chkconfig --level 35 nginx on');
os.system('/etc/init.d/nginx restart');
else:
os.system('rm -rf nginx.sh');
print '\033[31mdownload nginx.sh failed... \033[0m';
os.exit();
def install():
install_gcc_make();
adduser();
download_nginx();
install_nginx();
nginx_service();
install();
shell脚本之nginx安装
原创wx61c45e4794bd5 ©著作权
文章标签 nginx 开机启动 javascript 文章分类 代码人生
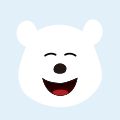
-
自动安装nginx,shell脚本
自动安装nginx脚本,自动配置nginx虚拟机脚本
nginx安装 nginx1.9安装 nginx安装脚本 配置nginx虚拟机 自动配置nginx虚拟机脚本 -
【shell案例】nginx编译安装脚本
前言本脚本没有使用函数,脚本安装的是nginx-1.16.1
nginx编译安装脚本 nginx 字符串 压缩包 -
Shell脚本编程--Nginx自动化安装
Shell脚本编程--Nginx自动化安装
Shell Nginx