<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>双向链表</title>
</head>
<body>
<script>
function DoubleList() {
this.head = null;
this.tail = null;
this.length = 0;
function Node(data) {
this.data = data;
this.prev = null;
this.next = null;
}
DoubleList.prototype.append = function (data) {
var newNode = new Node(data);
if (this.length == 0) {
this.head = newNode;
} else {
var current = this.head;
while (current.next) {
current = current.next;
}
current.next = newNode;
}
this.length += 1;
};
DoubleList.prototype.backwardString = function (data) {
//定义变量
var current = this.head;
var resultString = "";
while (current) {
resultString += current.data + " ";
current = current.next;
}
return resultString;
};
DoubleList.prototype.forwardString = function (data) {
//定义变量
var current = this.tail;
var resultString = "";
while (current) {
resultString += current.data + " ";
current = current.prev;
}
return resultString;
};
DoubleList.prototype.toString = function (data) {
//定义变量
return this.backwardString();
};
DoubleList.prototype.insert = function (position, data) {
if (position < 0 || position > this.length) return false;
var newNode = new Node(data);
if (this.length == 0) {
this.head = newNode;
this.tail = newNode;
} else {
if (position == 0) {
this.head.prev = newNode;
newNode.next = this.head;
this.head = newNode;
} else if (position == this.length) {
newNode.prev = this.tail;
this.tail.next = newNode;
this.tail = newNode;
} else {
var current = this.head;
var index = 0;
while (index++ < position) {
current = current.next;
}
newNode.next = current;
newNode.prev = current.prev;
current.prev.next = newNode;
current.prev = newNode;
}
}
this.length += 1;
return true;
};
DoubleList.prototype.get = function (position, data) {
if (position < 0 || position > this.length) return null;
//获取元素
var current = this.head;
var index = 0;
while (index++ < position) {
current = current.next;
}
return current.data;
};
DoubleList.prototype.indexOf = function (data) {
var current = this.head;
var index = 0;
//开始查找
while (current) {
if (current.data == data) {
return index;
}
current = current.next;
index += 1;
}
return -1;
};
DoubleList.prototype.indexOf = function (position, newData) {
if (position < 0 || position > this.length) return false;
var current = this.head;
var index = 0;
while (index++ < position) {
current = current.next;
}
current.data = newData;
return true;
};
DoubleList.prototype.removeAt = function (position) {
if (position < 0 || position >= this.length) return null;
var current=this.head
if(this.length==1){
this.head=null
this.tail=null
}else{
if(position==0){
this.head.next.prev=null
this.head=this.head.next
}else if(position=this.length){
current=this.tail
this.tail.next.prev=null
this.tail=this.tail.prev
}else{
var index=0
while(index++<position){
current=current.next
}
current.prev.next=current.next
current.next.prev=current.prev
}
}
this.length-=1
return current.data
}
}
</script>
</body>
</html>
数据结构69-双向链表removeAt方法代码
原创
©著作权归作者所有:来自51CTO博客作者前端导师歌谣的原创作品,请联系作者获取转载授权,否则将追究法律责任
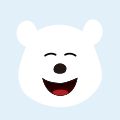
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
【数据结构】链式家族的成员——循环链表与静态链表
【数据结构】第二章——线性表(8)详细介绍了循环链表与静态链表的相关内容……
数据结构 C语言 循环链表 静态链表 -
数据结构45-链表removeAt方法实现链表 数据结构
-
数据结构64-双向链表indexOf方法代码
前端
数据结构 链表 javascript html 双向链表 -
Java数据结构——代码实现双向链表的方法
一文速学双向链表方法的实现
链表 数据结构 java 双向链表 结点 -
Redis 数据结构-双向链表
Redis 数据结构-双向链表 最是人间留不住,朱颜辞镜花辞树。 1、简介 Redis 之所以
Redis-双向链表 链表 双向链表 数据结构