package com.visystem.util;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import org.apache.commons.io.FileUtils;
import org.apache.commons.io.IOUtils;
import org.apache.commons.vfs2.FileObject;
import org.apache.commons.vfs2.FileSystemException;
import org.apache.commons.vfs2.FileSystemManager;
import org.apache.commons.vfs2.FileSystemOptions;
import org.apache.commons.vfs2.VFS;
import org.apache.commons.vfs2.provider.ftp.FtpFileSystemConfigBuilder;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* vfs使用工具类
* @author julong
* @date 2019年3月29日 下午3:06:22
* @desc 下
*
*/
public class VFSUtils {
private static final Logger logger = LoggerFactory.getLogger(VFSUtils.class);
private static FileSystemManager instance = null;
/**
* 可以操作的文件
* jar:../lib/classes.jar!/META-INF/manifest.mf
* zip:http://somehost/downloads/somefile.zip
* jar:zip:outer.zip!/nested.jar!/somedir
* jar:zip:outer.zip!/nested.jar!/some%21dir
* tar:gz:http://anyhost/dir/mytar.tar.gz!/mytar.tar!/path/in/tar/README.txt
* tgz:file://anyhost/dir/mytar.tgz!/somepath/somefile
* gz:/my/gz/file.gz
* hdfs://somehost:8080/downloads/some_dir
* hdfs://somehost:8080/downloads/some_file.ext
* webdav://somehost:8080/dist
* http://somehost:8080/downloads/somefile.jar
* http://myusername@somehost/index.html
* ftp://myusername:mypassword@somehost/pub/downloads/somefile.tgz
* ftps://myusername:mypassword@somehost/pub/downloads/somefile.tgz
* sftp://myusername:mypassword@somehost/pub/downloads/somefile.tgz
* tmp://dir/somefile.txt
* res:path/in/classpath/image.png
* ram:///any/path/to/file.txt
* mime:file:///your/path/mail/anymail.mime!/
* mime:file:///your/path/mail/anymail.mime!/filename.pdf
* mime:file:///your/path/mail/anymail.mime!/_body_part_0
* @return
* @throws FileSystemException
* @author julong
* @date 2019年3月29日 下午6:34:23
* @desc
*/
public static synchronized FileSystemManager getInstanceManager() throws FileSystemException{
logger.debug("【vfs使用工具类】-初始化信息!");
if (instance == null) {
try {
FtpFileSystemConfigBuilder builder = FtpFileSystemConfigBuilder.getInstance();
FileSystemOptions options = new FileSystemOptions();
//解决中文乱码
builder.setControlEncoding(options, "UTF-8");
// builder.setServerLanguageCode(options, "zh");
instance = VFS.getManager();
} catch (FileSystemException e) {
// TODO: handle exception
logger.debug("【vfs使用工具类】-初始化信息失败!",e);
throw new FileSystemException(e);
}
}
return instance;
}
/**
* 拼接ftpURL
* @param username
* @param password
* @param hostname
* @param port
* @param relativePath
* @return
* @author julong
* @date 2019年3月29日 下午6:38:46
* @desc
*/
public static String concatFTPURL(String username,String password,String hostname,String port,String relativePath){
StringBuffer sb = new StringBuffer();
sb.append("ftp://");
sb.append(username);
sb.append(":");
sb.append(password);
sb.append("@");
sb.append(hostname);
sb.append(":");
sb.append(port);
sb.append(relativePath);
return sb.toString();
}
/**
* vfs工具下载文件
* @param downloadURL
* @return InputStream
* @throws IOException
* @author julong
* @date 2019年3月29日 下午3:42:09
* @desc
*/
public static InputStream downloadFileToInputStream(String downloadURL) throws IOException{
logger.debug("【vfs使用工具类】-downloadFileToInputStream下载文件downloadURL:{}",downloadURL);
FileObject ftpFile = instance.resolveFile(downloadURL);
return ftpFile.getContent().getInputStream();
}
/**
* vfs工具下载文件
* @param downloadURL
* @return byte[]
* @throws IOException
* @author julong
* @date 2019年3月29日 下午3:43:24
* @desc
*/
public static byte[] downloadFileToByte(String downloadURL) throws IOException{
logger.debug("【vfs使用工具类】-downloadFileToByte下载文件downloadURL:{}",downloadURL);
FileObject ftpFile = instance.resolveFile(downloadURL);
return IOUtils.toByteArray(ftpFile.getContent().getInputStream());
}
/**
* vfs工具写入文件
* @param filePath
* @param bytes
* @throws IOException
* @author julong
* @date 2019年3月29日 下午3:43:35
* @desc
*/
public static void writeByteArrayToFile(String filePath,byte[] bytes) throws IOException{
logger.debug("【vfs使用工具类】-文件写入filePath:{}",filePath);
FileUtils.writeByteArrayToFile(new File(filePath), bytes);
}
/**
* 上传文件
* @param uploadURL
* @param bytes
* @return
* @author julong
* @date 2019年3月29日 下午5:44:30
* @desc
*/
public static boolean uploadFileToByte(String uploadURL,byte[] bytes){
logger.debug("【vfs使用工具类】-文件上传uploadURL:{}",uploadURL);
OutputStream output = null;
try {
long byteLength = bytes.length;
FileObject ftpFile = instance.resolveFile(uploadURL);
if(null != ftpFile && ftpFile.exists() == false){
ftpFile.createFile();
}
output = ftpFile.getContent().getOutputStream();
IOUtils.write(bytes,output);
IOUtils.closeQuietly(output);
if(ftpFile.isFile()){
long size = ftpFile.getContent().getSize();
if(byteLength == size){
logger.debug("【vfs使用工具类】-文件上传成功size:{}",size);
return true;
}
}else{
logger.debug("【vfs使用工具类】-创建文件夹成功getURL:{}",ftpFile.getURL());
return true;
}
return false;
}catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
logger.error("【vfs使用工具类】-文件上传发生异常:",e);
}finally{
if(output != null){
try {
output.close();
output.flush();
} catch (Exception e2) {
// TODO: handle exception
}
}
}
return false;
}
public static void main(String[] args) {
// TODO Auto-generated method stub
try {
VFSUtils.getInstanceManager();
String concatFTPURL = VFSUtils.concatFTPURL("vicp", "vicp", "192.168.10.132", "21", "/git.txt");
System.out.println(concatFTPURL);
byte[] bytes = VFSUtils.downloadFileToByte(concatFTPURL);
System.out.println(bytes.length);
boolean result = VFSUtils.uploadFileToByte("ftp://vicp:vicp@192.168.10.132/gggg/vvv/bbb/git1.txt", bytes);
System.out.println(result);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
commons-vfs 工具实现上传下载
原创
©著作权归作者所有:来自51CTO博客作者口袋里的小龙的原创作品,请联系作者获取转载授权,否则将追究法律责任
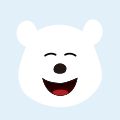
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
SpringBoot项目整合MinIO实现文件的上传下载
SpringBoot项目整合MinIO实现文件的上传下载
spring java 文件路径 -
上传下载
上传与下载从互联网上下载https://mirrors.huaweicloud.com/wget格式:wget 需要被下载的连接**
linux nginx https ssl docker -
Commons-VFS 使用SFTP
http://pro.ctlok.com/2011/06/apache-commons-vfs-for-sftp.html
Commons-VFS apache html -
java文件上传下载视频 java文件上传下载实现
数据上传是客户端向服务器端上传数据,客户端向服务器发送的所有请求都属于数据上传。文件上传是数据上传的一种特例,指客户端向服务器上传文件。即将保存在客户端的文件上传一个副本到服务器,并保存在服务器中。
java文件上传下载视频 java上传下载 服务器 客户端 数据