<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>汇率计算器</title>
</head>
<body>
<div id="app">
<p class="title">汇率计算器</p>
<ul>
<li>
<span>{{from.currency}}</span>
<input type="text" v-model="from.amount">
</li>
<li v-for="item in to">
<span>{{item.currency}}</span>
<span>{{item.amount}}</span>
</li>
</ul>
<p class="intro">用鼠标单击可以切换货币种类</p>
</div>
<script src="../js/vue.js"></script>
<script>
let rate = {
CNY:{
CNY: 1,
JPY:16.876,
HKD:1.1870,
USD:0.1526,
EUR:0.1294,
},
JPY:{
CNY:0.0595,
JPY:1,
HKD:0.0702,
USD:0.0090,
EUR:0.0077,
},
HKD:{
CNY:0.8463,
JPY:14.226,
HKD:1,
USD:0.1286,
EUR:0.10952,
},
USD:{
CNY:6.5813,
JPY:110.62,
HKD:7.7759,
USD:1,
EUR:0.85164,
},
EUR:{
CNY:7.7278,
JPY:129.89,
HKD:9.1304,
USD:1.1742,
EUR:1,
}
};
let vm = new Vue({
el:'#app',
data:{
from:{
currency:'CNY',
amount:100
},
to:[
{
currency: 'JPY',
amount: 0,
},
{
currency: 'HKD',
amount: 0,
},
{
currency: 'USD',
amount: 0,
},
{
currency: 'EUR',
amount: 0,
},
]
},
watch:{
from:{
handler(value){
this.to.forEach(item=>{
item.amount = this.exchange(this.from.currency,this.from.amount,item.currency)
});
},
deep:true,
immediate:true
}
},
methods:{
exchange(from,amount,to){
return (amount * rate[from][to]).toFixed(2);
}
}
});
</script>
</body>
</html>
vue-前端第8章demo03.html
原创
©著作权归作者所有:来自51CTO博客作者虾米大王的原创作品,请联系作者获取转载授权,否则将追究法律责任
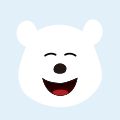
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
vue-前端第11章ajax-demo03.html
【代码】vue-前端第11章ajax-demo03.html。
vue.js html ios ajax -
vue-前端第8章demoTwo03.html
【代码】vue-前端第8章demoTwo03.html。
vue.js javascript java sed html -
vue-前端第8章demo02.html
【代码】vue-前端第8章demo02.html。
javascript 前端 html vue.js Vue -
vue-前端第8章demo04.html
【代码】vue-前端第8章demo04.html。
javascript html 前端 vue.js Vue -
vue-前端第8章demo01.html
【代码】vue-前端第8章demo01.html。
html servlet java vue.js Vue -
vue-前端第6章form03.html
【代码】vue-前端第6章form03.html。
vue.js javascript 前端 html Vue -
vue-前端第4章class03.html
【代码】vue-前端第4章class03.html。
javascript vue.js css html -
vue-前端第12章transition03.html
【代码】vue-前端第12章transition03.html。
vue.js html 开发技术 css -
vue-前端第9章component03.html
【代码】vue-前端第9章component03.html。
vue.js html Vue -
vue-前端第3章watch03.html
【代码】vue-前端第3章watch03.html。
vue.js javascript 前端 html ci -
vue-前端第3章computed03.html
【代码】vue-前端第3章computed03.html。
vue.js javascript 前端 html Math -
vue-前端第2章instance03.html
【代码】vue-前端第2章instance03.html。
vue.js javascript 前端 html ci -
vue-前端第7章v-if03.html
【代码】vue-前端第7章v-if03.html。
vue.js javascript 前端 html Vue -
vue-前端第5章event03.html
【代码】vue-前端第5章event03.html。
vue.js javascript 前端 html Vue -
vue-前端第14章cart03.html
【代码】vue-前端第14章cart03.html。
vue.js Vue html Math -
vue-前端第1章basic03.html
【代码】vue-前端第1章basic03.html。
vue.js javascript 前端 sed html -
vue-前端第11章ajax-demo06.html
【代码】vue-前端第11章ajax-demo06.html。
javascript 前端 html ios ajax -
swift 音量改变监控
本文所提到的音频处理,特指以电脑为主要工具的数字化音频的制作与处理(数码音频),关于它的基本概念及原理,请参阅相关资料。 1、音频卡(声卡)的播放属性设置 正确安装好音频卡(声卡)的驱动程序后,Windows的状态栏右边将出现一个小喇叭
swift 音量改变监控 linux录制声卡声音 Windows 属性设置 状态栏